2. Phonon Dispersion#
This is a simple example of how to compute the phonon dispersion using the MatterSim.
2.1. Import the necessary modules#
First we import the necessary modules. It is worth noting
that we have a built-in workflow for phonon calculations using phonopy
in MatterSim.
1import numpy as np
2from ase.build import bulk
3from ase.units import GPa
4from ase.visualize import view
5from mattersim.forcefield.potential import MatterSimCalculator
6from mattersim.applications.phonon import PhononWorkflow
2.2. Set up the MatterSim calculator#
1# initialize the structure of silicon
2si = bulk("Si")
3
4# attach the calculator to the atoms object
5si.calc = MatterSimCalculator()
2.3. Set up the phonon workflow#
1ph = PhononWorkflow(
2 atoms=si,
3 find_prim = False,
4 work_dir = "/tmp/phonon_si_example",
5 amplitude = 0.01,
6 supercell_matrix = np.diag([4,4,4]),
7)
2.4. Compute the phonon dispersion#
1has_imag, phonons = ph.run()
2print(f"Has imaginary phonon: {has_imag}")
3print(f"Phonon frequencies: {phonons}")
2.5. Inspect the phonon dispersion#
Once the calculation is done, you can find the phonon plot in the work directory.
In this case, you find the plot in the directory /tmp/phonon_si_example
,
and here is the phonon dispersion plot for the example above.
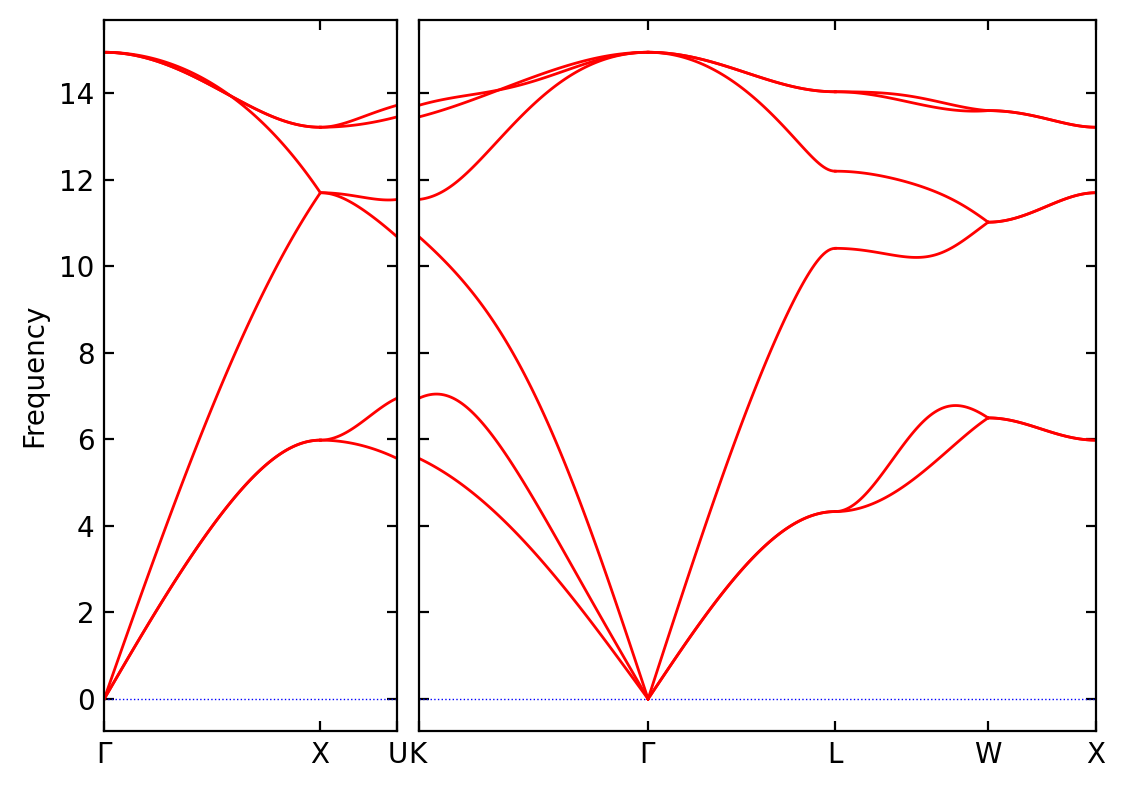