Spring Cloud Azure offers a convenient way to interact with Azure provided services using well-known Spring idioms and APIs for Spring developers.
1. What is New in 4.0 since 3.10.x
This section covers the changes made from version 3.10 to version 4.0.0-beta.1. With this major release, we aim to bring better security, leaner dependencies, support for production readiness, and more.
To learn how to migration to 4.0, please check the Appendix page. |
1.1. Unified Development Experience
We constantly challenge ourselves on how we can make things more consistent and easier to understand, so our customers are not confronted with haphazard development choices. This is a long and self-evolving journey as consistency is relative and there will be things that are outside of our control. We now humbly take another step in this direction to improve to our developer experience by unifying project name, artifact ID and properties.
1.2. Simplified dependency management
Dependency management is one of the core value pillars that has helped Spring establish preeminence over to other Java frameworks. In that spirit, we have also been exploring ways to make dependency management easier for Spring developers on Azure. In this release, we have codified best practices and expertise from Spring experts and condensed all of our dependency BOMs into one, spring-cloud-azure-dependencies
, which we believe will further bring down the learning curve and avoid ill-handling of dependencies.
1.3. Expanded support scope of Azure Support on start.spring.io
The Azure Support module in Spring Initializr provides auto-configuration of many Azure services.
In this release we have expanded the scope of Azure Support to cover the additional 4 more services:
-
Kafka
-
Event Hubs
-
Azure Cache for Redis
-
App Configuration
Our journey does end here and over time we will bring even more Azure services into the fold.
1.4. Untethered and unrestrained
One feedback that we consistently hear is our Spring modules are unnecessarily stacked on top of too many layers of dependencies, which has prevented broader adoption. As an example, all of our early Spring modules rely on Spring Boot, and many of our customers are running Spring MVC apps in Tomcat, leveraging nothing but Spring Data, as an example, to communicate with data services. We now realize the flaw in our original design, and have rearchitected our Spring module dependencies from the ground up, untethered from layers of excess and entanglement.
1.5. More control and secure
At the heart of every real-world application, is identity and secret management. Support for managed identity has become an Azure fundamental that are getting mandated as a security baseline at individual services. We believe aligning on those guidelines will also benefit Spring developers at large, and have added Managed Identity support for App Configuration, Event Hub, Service Bus, Cosmos, Key Vault, Storage Blob, and Storage Queue. This enables building credential-free applications, which is a pattern that has picked up tremendous momentum both at Microsoft and in the community. In addition to Managed Identity, you can use any authentication methods supported in the underlying Azure SDK from our Spring libraries. For instance, you use SAS token and token credential to authenticate with Service Bus and Event Hubs. Credential chain is now enabled by default, allowing applications to obtain credentials from application properties, environment variables, managed identity, IDEs, etc. Lastly providing granular level access control at the resource level (i.e.: Service Bus queue), is often of paramount importance when it comes to meeting the needs of our enterprise customers. We’ve now unlocked these controls to our customers for better security governance and adherence to IT policies.
1.6. More options exposed in a Spring idiomatic way
Spring developers have long enjoyed the convenience of defining client options in application configuration files. We certainly do not want to take that privilege away and burden Spring developers with setting options via client objects. To that end, we’ve significantly improved autoconfiguration coverage of Azure SDK clients for both synchronous and asynchronous scenarios.
1.7. More Production Ready
Lastly all of the above would be in vain if we do not have enough feature coverage to support our customers in production. Many things come to my mind to make an application production-ready, but observability often arrives at the top. We’ve added health indicators for App Configuration, Event Hubs, Cosmos, Key Vault, Storage Blob, Storage Queue, Storage File, as well as Spring Cloud Sleuth support for all HTTP-based Azure SDKs. As an example, you now can prob if storage blob is up or down via Spring Boot actuator endpoint, as well as track dependencies and latencies going from your application to Cosmos DB.
2. Migration Guide for 4.0
To learn how to migration to 4.0, please check the Appendix page.
3. Getting Started
3.1. Setting up Dependencies
3.1.1. Bill of Material (BOM)
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-dependencies</artifactId>
<version>4.0.0-beta.1</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
3.1.2. Starter Dependencies
Spring Cloud Azure Starters are a set of convenient dependency descriptors to include in your application. Each starter contains all the dependencies and transitive dependencies needed to begin using their corresponding Spring Cloud Azure module. It boosts your Spring Boot application development with Azure services.
For example, if you want to get started using Spring and Azure Cosmos DB for data persistence, include the spring-cloud-azure-starter-cosmos
dependency in your project.
The following application starters are provided by Spring Cloud Azure under the com.azure.spring
group:
Name | Description |
---|---|
spring-cloud-azure-starter |
Core starter, including auto-configuration support |
spring-cloud-azure-starter-active-directory |
Starter for using Azure Active Directory with Spring Security |
spring-cloud-azure-starter-active-directory-b2c |
Starter for using Azure Active Directory B2C with Spring Security |
spring-cloud-azure-starter-appconfiguration |
Starter for using Azure App Configuration |
spring-cloud-azure-starter-cosmos |
Starter for using Azure Cosmos DB |
spring-cloud-azure-starter-eventhubs |
Starter for using Azure Event Hubs |
spring-cloud-azure-starter-keyvault-secrets |
Starter for using Azure Key Vault Secrets |
spring-cloud-azure-starter-servicebus |
Starter for using Azure Service Bus |
spring-cloud-azure-starter-servicebus-jms |
Starter for using Azure Service Bus and JMS |
spring-cloud-azure-starter-storage-blob |
Starter for using Azure Storage Blob |
spring-cloud-azure-starter-storage-file-share |
Starter for using Azure Storage File Share |
spring-cloud-azure-starter-storage-queue |
Starter for using Azure Storage Queue |
spring-cloud-azure-starter-actuator |
Starter for using Spring Boot’s Actuator which provides production ready features |
Below are starters for Spring Data support:
Name | Description |
---|---|
spring-cloud-azure-starter-data-cosmos |
Starter for using Azure Cosmos DB and Spring Data Cosmos DB |
Below are starters for Spring Integration support:
Name | Description |
---|---|
spring-cloud-azure-starter-integration-eventhubs |
Starter for using Azure Event Hubs and Spring Integration |
spring-cloud-azure-starter-integration-servicebus |
Starter for using Azure Service Bus and Spring Integration |
spring-cloud-azure-starter-integration-storage-queue |
Starter for using Azure Storage Queue and Spring Integration |
Below are starters for Spring Cloud Stream support:
Name | Description |
---|---|
spring-cloud-azure-starter-stream-eventhubs |
Starter for using Azure Event Hubs and Spring Cloud Stream Binder |
spring-cloud-azure-starter-stream-servicebus |
Starter for using Azure Service Bus and Spring Cloud Stream Binder |
3.2. Learning Spring Cloud Azure
We prepared a full list of samples to show the usages, can be found at Spring Cloud Azure Samples
4. Configuration
Most of Azure Service SDKs could be divided into two categories by transport type, HTTP-based and AMQP-based. There are properties that are common to all SDKs such as authentication principals and Azure environment settings. Or common to HTTP-based clients, such as logging level to log http requests and responses. In Spring Cloud Azure 4.0 we added five common categories of configuration properties, which could be specified to each Azure service.
Prefix | Description |
---|---|
spring.cloud.azure.<azure-service>.client |
To configure the transport clients underneath one Azure service SDK. |
spring.cloud.azure.<azure-service>.credential |
To configure how to authenticate with Azure Active Directory for one Azure service SDK. |
spring.cloud.azure.<azure-service>.profile |
To configure the Azure cloud environment for one Azure service SDK. |
spring.cloud.azure.<azure-service>.proxy |
To configure the proxy options for one Azure service SDK. |
spring.cloud.azure.<azure-service>.retry |
To configure the retry options apply to one Azure service SDK. |
There are some properties that could be shared among different Azure services, for example using the same service principal to access Azure Cosmos DB and Azure Event Hubs. Spring Cloud Azure 4.0 allows developers to define properties that apply to all Azure SDKs in the namespace spring.cloud.azure
.
Prefix | Description |
---|---|
spring.cloud.azure.client |
To configure the transport clients apply to all Azure SDKs by default. |
spring.cloud.azure.credential |
To configure how to authenticate with Azure Active Directory for all Azure SDKs by default. |
spring.cloud.azure.profile |
To configure the Azure cloud environment for all Azure SDKs by default. |
spring.cloud.azure.proxy |
To configure the proxy options apply to all Azure SDK clients by default. |
spring.cloud.azure.retry |
To configure the retry options apply to all Azure SDK clients by default. |
Properties configured under each Azure service will override the global configurations. |
The configuration properties' prefixes have been unified to spring.cloud.azure
namespace since Spring Cloud Azure 4.0, it will make configuration properties more consistent and more intuitive. Here’s a quick review of the prefixes of supported Azure services.
Azure Service | Configuration Property Prefix |
---|---|
Azure App Configuration |
spring.cloud.azure.appconfiguration |
Azure Cosmos DB |
spring.cloud.azure.cosmos |
Azure Event Hubs |
spring.cloud.azure.eventhubs |
Azure Key Vault Certificate |
spring.cloud.azure.keyvault.certificate |
Azure Key Vault Secret |
spring.cloud.azure.keyvault.secret |
Azure Service Bus |
spring.cloud.azure.servicebus |
Azure Storage Blob |
spring.cloud.azure.storage.blob |
Azure Storage File Share |
spring.cloud.azure.storage.fileshare |
Azure Storage Queue |
spring.cloud.azure.storage.queue |
5. Authentication
5.1. DefaultAzureCredential
The DefaultAzureCredential
is appropriate for most scenarios where the application is intended to ultimately be run in the Azure Cloud. This is because the DefaultAzureCredential combines credentials commonly used to authenticate when deployed, with credentials used to authenticate in a development environment.
DefaultAzureCredential is intended to simplify getting started with the SDK by handling common scenarios with reasonable default behaviors. Developers who want more control or whose scenario isn’t served by the default settings should use other credential types. |
The DefaultAzureCredential
will attempt to authenticate via the following mechanisms in order.
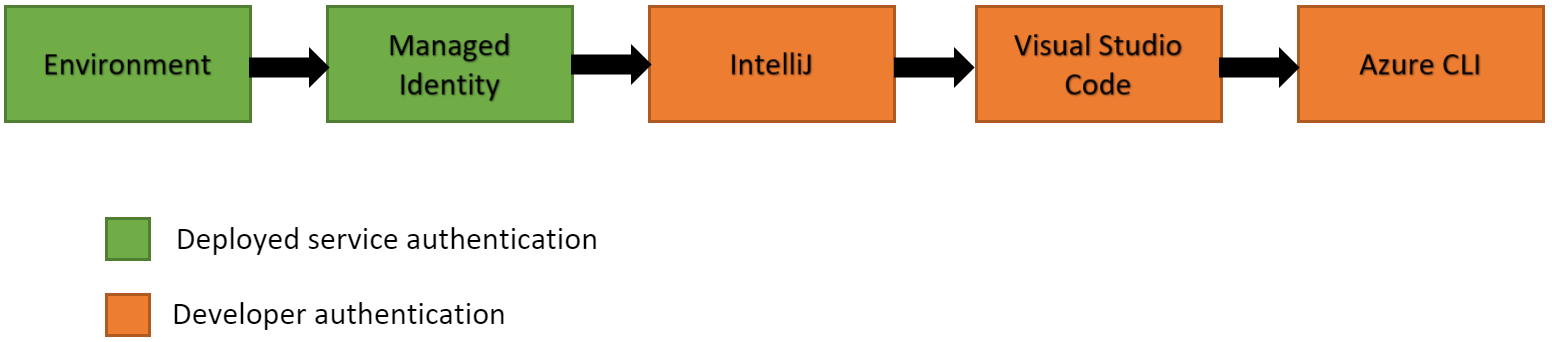
-
Environment - The
DefaultAzureCredential
will read account information specified via environment variables and use it to authenticate. -
Managed Identity - If the application is deployed to an Azure host with Managed Identity enabled, the
DefaultAzureCredential
will authenticate with that account. -
IntelliJ - If the developer has authenticated via Azure Toolkit for IntelliJ, the
DefaultAzureCredential
will authenticate with that account. -
Visual Studio Code - If the developer has authenticated via the Visual Studio Code Azure Account plugin, the
DefaultAzureCredential
will authenticate with that account. -
Azure CLI - If the developer has authenticated an account via the Azure CLI az login command, the
DefaultAzureCredential
will authenticate with that account.
There could be some ERROR logs be printed out while the DefaultAzureCredential running the chain and trying to find the first available credential. It doesn’t mean the DefaultAzureCredential is broken or unavailable. Meanwhile, we’ll keep improving this logging experience.
|
5.2. Managed Identity
A common challenge for developers is the management of secrets and credentials used to secure communication between different components making up a solution. Managed identities eliminate the need for developers to manage credentials. Managed identities provide an identity for applications to use when connecting to resources that support Azure Active Directory (Azure AD) authentication. Applications may use the managed identity to obtain Azure AD tokens. For example, an application may use a managed identity to access resources like Azure Key Vault where developers can store credentials in a secure manner or to access storage accounts.
We encourage using manged identity instead of using connection string or key in your application for it’s more secure and will save the trouble of managing secrets and credentials. In this case, DefaultAzureCredential
could better serve the scenario of developing locally using account information stored locally and deploying the application to Azure Cloud and using Manged Identity.
5.3. Other Credential Types
Developers who want more control or whose scenario isn’t served by the DefaultAzureCredential
or whose scenario isn’t served by the default settings should use other credential types.
5.3.1. Authentication with Azure Active Directory
For applications want to connect to resources that support Azure Active Directory (Azure AD) authentication, below confiugrations could be set with prefix spring.cloud.azure.credential
or spring.cloud.azure.<azure-service>.credential
Property Name | Description |
---|---|
client-id |
Client id to use when performing service principal authentication with Azure. |
client-serect |
Client secret to use when performing service principal authentication with Azure. |
client-certificate-path |
Client secret to use when performing service principal authentication with Azure. |
client-certificate-password |
Password of the certificate file. |
username |
Username to use when performing username/password authentication with Azure. |
password |
Password to use when performing username/password authentication with Azure. |
managed-identity-client-id |
Client id to use when using managed identity to authenticate with Azure. |
To see the list of all Spring Cloud Azure related configuration properties please check the Appendix page. |
5.3.2. SAS Token
It’s also configurable for services support authenticating with Shared Access Signature (SAS). spring.cloud.azure.<azure-servcie>.sas-token
is the propety to configure. For example, using spring.cloud.azure.storage.blob.sas-token
to authenticate to Storage Blob service.
5.3.3. Connection String
Connection string are supported by some Azure services to provide connection informantion as well as credentials. To connect to those Azure servcies using connection string, just configure spring.cloud.azure.<azure-service>.connection-string
will do. For example, spring.cloud.azure.eventhubs.connection-string
to connect to Event Hubs service.
6. Production Ready
We’ve added health indicators for App Configuration, Event Hubs, Cosmos, Key Vault, Storage Blob, Storage Queue, Storage File, as well as Spring Cloud Sleuth support for all HTTP-based Azure SDKs. As an example, you now can prob if storage blob is up or down via Spring Boot actuator endpoint, as well as track dependencies and latencies going from your application to Key Vault.
6.1. Enable Health Indicator
Add the Spring Cloud Azure Actuator Starter dependency. This dependency will also include the spring-boot-starter-actuator
.
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-actuator</artifactId>
</dependency>
Below properties could be used to enable or disable health indicators for each Azure services.
Azure Service | Propropety |
---|---|
App Configuration |
management.health.azure-appconfiguration.enabled |
Cosmos DB |
management.health.azure-cosmos.enabled |
Event Hubs |
management.health.azure-eventhubs.enabled |
Key Vault |
management.health.azure-keyvault.enabled |
Storage |
management.health.azure-storage.enabled |
Calling the health endpoint of Azure servcies may cause extra charge. For example, calling {hostname}:{port}/actuator/health/cosmos to get the Cosmos DB health info, it will calculate RUs.
|
6.2. Enable Sleuth
Add the Spring Cloud Azure Trace Sleuth dependency when you want to trace Azure SDK activities with using Spring Cloud Sleuth.
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-trace-sleuth</artifactId>
</dependency>
Only HTTP-based Azure SDK clients are supported now, for example, Eventhub and ServiceBus with AMQP transport are currently not supported, we recommend to use Azure Application Insight for such requirement. |
7. Auto Configure Azure SDK Clients
Spring Boot greatly simplifies the Spring Cloud Azure experience. Spring Cloud Azure Starters are a set of convenient dependency descriptors to include in your application. Our starters handle the object instantiation and configuration logic so you don’t have to. Every starter depends on the Spring Cloud Azure starter to provide critical bits of configuration, like the Azure Cloud environment and authentication information. You can configure these as properties in, for example, a yaml file:
spring:
cloud:
azure:
profile:
tenant-id: ${AZURE_TENANT_ID}
cloud: Azure
credential:
client-id: ${AZURE_CLIENT_ID}
These properties are optional and, if not specified, Spring Boot will attempt to automatically find them for you. For details on how Spring Boot finds these properties, refer to the documentation.
7.1. Dependency Setup
There are two ways to use Spring Cloud Azure starters, one is using Azure SDKs with this spring-cloud-azure-starter
dependency
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter</artifactId>
</dependency>
or without adding Azure SDK dependencies and including the Spring Cloud Azure Starter for each Service directly. For example with Cosmos DB
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-cosmos</artifactId>
</dependency>
Please refer to Starter Dependencies for the list of starters we support. |
7.2. Configuration
Configuration properties for each Azure service are under prefix spring.cloud.azure
.
To see the list of all Spring Cloud Azure related configuration properties please check the Appendix page. |
7.3. Basic Usage
Adding below properties to your application.yaml
will auto-configure the Cosmos Client for you, both CosmosClient
and CosmosAsyncClient
are available in the context and could be autowired.
spring:
cloud:
azure:
cosmos:
database: ${AZURE_COSMOS_DATABASE_NAME}
endpoint: ${AZURE_COSMOS_ENDPOINT}
consistency-level: eventual
connection-mode: direct
@Autowired
private CosmosClient cosmosClient;
@Override
public void run() {
User item = User.randomUser();
CosmosContainer container = cosmosClient.getDatabase(databaseName).getContainer(containerName);
container.createItem(item);
}
7.4. Samples
Check here for more samples.
8. Resource Handling
Connect to Azure Storage using Spring Resource abstraction and Azure Storage libraries.
Starter |
Service |
Description |
spring-cloud-azure-starter-storage-blob |
Azure Blobs |
Allows unstructured data to be stored and accessed at a massive scale in block blobs. |
spring-cloud-azure-starter-storage-file-share |
Azure Files |
Offers fully managed cloud file shares that you can access from anywhere via the industry standard Server Message Block (SMB) protocol. |
8.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-storage-blob</artifactId>
</dependency>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-storage-file-share</artifactId>
</dependency>
8.2. Configuration
This spring-cloud-azure-starter-storage-blob
provides the following properties:
Name | Default | Description |
---|---|---|
spring.cloud.azure.storage.blob.enabled |
|
Whether an Azure Blob Storage Service is enabled |
spring.cloud.azure.storage.blob.endpoint |
Uri to connect Azure Blob Storage |
|
spring.cloud.azure.storage.blob.account-key |
Privatekey to connect Azure Blob Storage |
|
spring.cloud.azure.storage.blob.account-name |
Azure Storage Account Name |
This spring-cloud-azure-starter-storage-file-share
provides the following properties:
Name | Default | Description |
---|---|---|
spring.cloud.azure.storage.fileshare.enabled |
|
Whether Azure File Storage Service is enabled |
spring.cloud.azure.storage.fileshare.endpoint |
Uri to connect Azure File Storage |
|
spring.cloud.azure.storage.fileshare.account-key |
Privatekey to connect Azure File Storage |
|
spring.cloud.azure.storage.fileshare.account-name |
Azure Storage Account Name |
8.3. Basic Usage
Provide the properties below in your configuration file.
spring:
cloud:
azure:
storage:
blob:
account-name: ${STORAGE_ACCOUNT_NAME}
account-key: ${STORAGE_ACCOUNT_PRIVATE_KEY}
endpoint: ${STORAGE_BLOB_ENDPOINT}
fileshare:
account-name: ${STORAGE_ACCOUNT_NAME}
account-key: ${STORAGE_ACCOUNT_PRIVATE_KEY}
endpoint: ${STORAGE_FILESHARE_ENDPOINT}
8.4. Samples
A storage-blob-sample and storage-file-sample are provided to show how to use the spring-cloud-azure-starter-storage-blob
and spring-cloud-azure-starter-storage-file-share
.
9. Secret Management
spring-cloud-azure-starter-keyvault-secrets adds Azure Key Vault as one of the Spring PropertySource, so secrets stored in Azure Key Vault could be easily used and conveniently accessed like other externalized configuration property, e.g. properties in files.
9.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-keyvault-secrets</artifactId>
</dependency>
9.2. Configuration
Configurable items
Properties | Description |
---|---|
spring.cloud.azure.keyvault.secret.endpoint |
Key Vault uri |
spring.cloud.azure.keyvault.secret.service-version |
Service version |
spring.cloud.azure.keyvault.secret.property-source-enabled |
Enable this property source |
spring.cloud.azure.keyvault.secret.property-sources |
Multiple property source |
spring.cloud.azure.keyvault.secret.property-sources[].name |
Name of this property source |
spring.cloud.azure.keyvault.secret.property-sources[].endpoint |
Key Vault uri |
spring.cloud.azure.keyvault.secret.property-sources[].service-version |
Service version |
spring.cloud.azure.keyvault.secret.property-sources[].case-sensitive |
Whether the secret name is case-sensitive |
spring.cloud.azure.keyvault.secret.property-sources[].secret-keys |
The supported secret names. If not configured, it will retrieve all secret names. |
spring.cloud.azure.keyvault.secret.property-sources[].refresh-interval |
Refresh interval |
9.3. Basic Usage
9.3.1. One property source
Property configuration
spring:
cloud:
azure:
profile:
tenant-id: ${AZURE_TENANT_ID}
credential:
client-id: ${AZURE_CLIENT_ID}
client-secret: ${AZURE_CLIENT_SECRET}
keyvault:
secret:
property-source-enabled: true
endpoint: ${AZURE_KEYVAULT_ENDPOINT}
Java code
@SpringBootApplication
public class KeyVaultSample implements CommandLineRunner {
@Value("${your-property-name}")
private String mySecretProperty;
public static void main(String[] args) {
SpringApplication.run(KeyVaultSample.class, args);
}
@Override
public void run(String... args) {
System.out.println("property your-property-name value is: " + mySecretProperty);
}
}
9.3.2. Multiple property source
Property configuration
spring:
cloud:
azure:
keyvault:
secret:
property-source-enabled: true
property-sources:
-
name: key-vault-1
endpoint: ${ENDPOINT_1}
profile:
tenant-id: ${AZURE_TENANT_ID_1}
credential:
client-id: ${AZURE_CLIENT_ID_1}
client-secret: ${AZURE_CLIENT_SECRET_1}
-
name: key-vault-2
endpoint: ${ENDPOINT_2}
profile:
tenant-id: ${AZURE_TENANT_ID_2}
credential:
client-id: ${AZURE_CLIENT_ID_2}
client-secret: ${AZURE_CLIENT_SECRET_2}
Java code
@SpringBootApplication
public class SampleApplication implements CommandLineRunner {
@Value("${secret-name-in-key-vault-1}")
private String secretNameInKeyVault1;
@Value("${secret-name-in-key-vault-2}")
private String secretNameInKeyVault2;
@Value("${secret-name-in-key-vault-both}")
private String secretNameInKeyVaultBoth;
public static void main(String[] args) {
SpringApplication.run(SampleApplication.class, args);
}
public void run(String[] args) {
System.out.println("secretNameInKeyVault1: " + secretNameInKeyVault1);
System.out.println("secretNameInKeyVault2: " + secretNameInKeyVault2);
System.out.println("secretNameInKeyVaultBoth: " + secretNameInKeyVaultBoth);
}
}
9.4. Samples
Please refer to this repo to get sample project.
10. Spring Data Support
10.1. Spring Data CosmosDB Support
Azure Cosmos DB is a globally-distributed database service that allows developers to work with data using a variety of standard APIs, such as SQL, MongoDB, Graph, and Azure Table storage.
Connect to Cosmos DB using Spring Data and CosmosDB libraries.
10.2. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-data-cosmos</artifactId>
</dependency>
10.3. Configuration
Below are some properties you can configure when using Spring Data CosmosDB Support:
Name | Description | Default |
---|---|---|
spring.cloud.azure.cosmos.enabled |
Whether Azure Cosmos Service is enabled. |
|
spring.cloud.azure.cosmos.database |
The CosmosDB database id |
|
spring.cloud.azure.cosmos.endpoint |
Uri to connect CosmosDB |
|
spring.cloud.azure.cosmos.key |
PrivateKey to connect CosmosDB |
|
spring.cloud.azure.cosmos.credential.client-certificate-password |
Password of the certificate file. |
|
spring.cloud.azure.cosmos.credential.client-certificate-path |
Path of a PEM certificate file to use when performing service principal authentication with Azure. |
|
spring.cloud.azure.cosmos.credential.client-id |
Client id to use when performing service principal authentication with Azure. |
|
spring.cloud.azure.cosmos.credential.client-secret |
Client secret to use when performing service principal authentication with Azure. |
|
spring.cloud.azure.cosmos.credential.managed-identity-client-id |
Client id to use when using managed identity to authenticate with Azure. |
|
spring.cloud.azure.cosmos.credential.password |
Password to use when performing username/password authentication with Azure. |
|
spring.cloud.azure.cosmos.credential.username |
Username to use when performing username/password authentication with Azure. |
|
spring.cloud.azure.cosmos.populate-query-metrics |
Populate Diagnostics Strings and Query metrics |
|
10.4. Basic Usage
10.4.1. Use private key to access CosmosDB
The simplest way to connect CosmosDB with spring-cloud-azure-starter-data-cosmos
is primary key,
add below properties and you are good to go.
spring:
cloud:
azure:
cosmos:
key: ${AZURE_COSMOS_KEY}
endpoint: ${AZURE_COSMOS_ENDPOINT}
database: ${AZURE_COSMOS_DATABASE}
10.5. Samples
Please refer to samples for Azure Spring Data Cosmos for more details.
11. Spring Security Support
11.1. Spring Security with Azure AD
11.1.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-active-directory</artifactId>
</dependency>
11.1.2. Configuration
This starter provides the following properties:
Properties | Description |
---|---|
spring.cloud.azure.active-directory.app-id-uri |
It used in resource server, used to validate the audience in access_token. access_token is valid only when the audience in access_token equal to client-id or app-id-uri |
spring.cloud.azure.active-directory.authorization-clients |
A map configure the resource APIs the application is going to visit. Each item corresponding to one resource API the application is going to visit. In Spring code, each item corresponding to one OAuth2AuthorizedClient object |
spring.cloud.azure.active-directory.authorization-clients.AZURE_CLIENT_NAME.scopes |
API permissions of a resource server that the application is going to acquire. |
spring.cloud.azure.active-directory.authorization-clients.AZURE_CLIENT_NAME.on-demand |
This is used for incremental consent. The default value is false. If it’s true, it’s not consent when user login, when application needs the additional permission, incremental consent is performed with one OAuth2 authorization code flow. |
spring.cloud.azure.active-directory.authorization-clients.AZURE_CLIENT_NAME.authorization-grant-type |
Type of authorization client. Supported types are authorization_code (default type for webapp), on_behalf_of (default type for resource-server), client_credentials. |
spring.cloud.azure.active-directory.application-type |
Refer to Application type. |
spring.cloud.azure.active-directory.base-uri |
Base uri for authorization server, the default value is |
spring.cloud.azure.active-directory.client-id |
Registered application ID in Azure AD. |
spring.cloud.azure.active-directory.client-secret |
client secret of the registered application. |
spring.cloud.azure.active-directory.graph-membership-uri |
It’s used to load users' groups. The default value is |
spring.cloud.azure.active-directory.post-logout-redirect-uri |
Redirect uri for posting log-out. |
spring.cloud.azure.active-directory.resource-server.principal-claim-name |
Principal claim name. Default value is "sub". |
spring.cloud.azure.active-directory.resource-server.claim-to-authority-prefix-map |
Claim to authority prefix map. Default map is: "scp" -> "SCOPE_", "roles" -> "APPROLE_". |
spring.cloud.azure.active-directory.tenant-id |
Azure Tenant ID. |
spring.cloud.azure.active-directory.user-group.allowed-group-names |
Users' group name can be use in |
spring.cloud.azure.active-directory.user-group.allowed-group-ids |
Users' group id can be use in |
spring.cloud.azure.active-directory.user-name-attribute |
Decide which claim to be principal’s name. |
Here are some examples about how to use these properties:
Property example 1: Application type
This property(spring.cloud.azure.active-directory.application-type
) is optional, its value can be inferred by dependencies, only web_application_and_resource_server
must be configured manually: spring.cloud.azure.active-directory.application-type=web_application_and_resource_server
.
Has dependency: spring-security-oauth2-client | Has dependency: spring-security-oauth2-resource-server | Valid values of application type | Default value |
---|---|---|---|
Yes |
No |
|
|
No |
Yes |
|
|
Yes |
Yes |
|
|
Property example 2: Use Azure China instead of Azure Global.
-
Step 1: Add property in application.yml
spring: cloud: azure: active-directory: base-uri: https://login.partner.microsoftonline.cn graph-base-uri: https://microsoftgraph.chinacloudapi.cn
Property example 3: Use group name
or group id
to protect some method in web application.
-
Step 1: Add property in application.yml
spring:
cloud:
azure:
active-directory:
user-group:
allowed-group-names: group1_name_1, group2_name_2
# 1. If allowed-group-ids == all, then all group id will take effect.
# 2. If "all" is used, we should not configure other group ids.
# 3. "all" is only supported for allowed-group-ids, not supported for allowed-group-names.
allowed-group-ids: group_id_1, group_id_2
-
Step 2: Add
@EnableGlobalMethodSecurity(prePostEnabled == true)
in web application:
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled == true)
public class AADOAuth2LoginSecurityConfig extends AADWebSecurityConfigurerAdapter {
/**
* Add configuration logic as needed.
*/
@Override
protected void configure(HttpSecurity http) throws Exception {
super.configure(http);
http.authorizeRequests()
.anyRequest().authenticated();
// Do some custom configuration
}
}
Then we can protect the method by @PreAuthorize
annotation:
@Controller
public class RoleController {
@GetMapping("group1")
@ResponseBody
@PreAuthorize("hasRole('ROLE_group1')")
public String group1() {
return "group1 message";
}
@GetMapping("group2")
@ResponseBody
@PreAuthorize("hasRole('ROLE_group2')")
public String group2() {
return "group2 message";
}
@GetMapping("group1Id")
@ResponseBody
@PreAuthorize("hasRole('ROLE_<group1-id>')")
public String group1Id() {
return "group1Id message";
}
@GetMapping("group2Id")
@ResponseBody
@PreAuthorize("hasRole('ROLE_<group2-id>')")
public String group2Id() {
return "group2Id message";
}
}
Property example 4: Incremental consent in Web application visiting resource servers.
-
Step 1: Add property in application.yml
spring:
cloud:
azure:
active-directory:
authorization-clients:
graph:
scopes: https://graph.microsoft.com/Analytics.Read, email
arm: # client registration id
on-demand: true # means incremental consent
scopes: https://management.core.windows.net/user_impersonation
-
Step 2: Write Java code:
After these steps. arm’s scopes (management.core.windows.net/user_impersonation) doesn’t
need to be consented at login time. When user request `/arm
endpoint, user need to consent the
scope. That’s incremental consent
means.
After the scopes have been consented, AAD server will remember that this user has already granted the permission to the web application. So incremental consent will not happen anymore after user consented.
Property example 5: [Client credential flow] in resource server visiting resource servers.
-
Step 1: Add property in application.yml
spring:
cloud:
azure:
active-directory:
authorization-clients:
webapiC: # When authorization-grant-type is null, on behalf of flow is used by default
authorization-grant-type: client_credentials
scopes:
- <Web-API-C-app-id-url>/.default
-
Step 2: Write Java code:
11.1.3. Basic Usage
Accessing a web application
This scenario uses The OAuth 2.0 authorization code grant flow to log in a user with a Microsoft account.
System diagram:
-
Step 1: Make sure
redirect URI
has been set toAPPLICATION_BASE_URI/login/oauth2/code/
, for examplelocalhost:8080/login/oauth2/code/
.
The tailing / cannot be omitted.
|
-
Step 2: Add the following dependencies in your pom.xml.
<dependencies>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-active-directory</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
</dependencies>
-
Step 3: Add properties in application.yml. These values should be got in prerequisite.
spring:
cloud:
azure:
active-directory:
tenant-id: ${AZURE_TENANT_ID}
client-id: ${AZURE_CLIENT_ID}
client-secret: ${AZURE_CLIENT_SECRET}
-
Step 4: Write your Java code:
The AADWebSecurityConfigurerAdapter
contains necessary web security configuration for aad-starter.
Web application accessing resource servers
System diagram:
-
Step 1: Make sure
redirect URI
has been set, just like Accessing a web application. -
Step 2: Add the following dependencies in you pom.xml.
<dependencies>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-active-directory</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
</dependencies>
-
Step 3: Add properties in application.yml:
spring:
cloud:
azure:
active-directory:
tenant-id: ${AZURE_TENANT_ID}
client-id: ${AZURE_CLIENT_ID}
client-secret: ${AZURE_CLIENT_SECRET}
authorization-clients:
graph:
scopes: https://graph.microsoft.com/Analytics.Read, email
Here, graph
is the name of OAuth2AuthorizedClient
, scopes
means the scopes need to consent when login.
-
Step 4: Write your Java code:
Accessing a resource server
This scenario doesn’t support login, just protect the server by validating the access_token. If the access token is valid, the server serves the request.
System diagram:
To use aad-starter in this scenario, we need these steps:
-
Step 1: Add the following dependencies in you pom.xml.
<dependencies> <dependency> <groupId>com.azure.spring</groupId> <artifactId>spring-cloud-azure-starter-active-directory</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-resource-server</artifactId> </dependency> </dependencies>
-
Step 2: Add properties in application.yml:
-
Step 3: Write Java code:
The AADResourceServerWebSecurityConfigurerAdapter
contains necessary web security configuration for resource server.
Resource server visiting other resource servers
This scenario support visit other resource servers in resource servers.
System diagram:
To use aad-starter in this scenario, we need these steps:
-
Step 1: Add the following dependencies in you pom.xml.
<dependencies> <dependency> <groupId>com.azure.spring</groupId> <artifactId>spring-cloud-azure-starter-active-directory</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-resource-server</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-client</artifactId> </dependency> </dependencies>
-
Step 2: Add properties in application.yml:
spring:
cloud:
azure:
active-directory:
tenant-id: ${AZURE_TENANT_ID}
client-id: ${AZURE_CLIENT_ID}
client-secret: ${AZURE_CLIENT_SECRET}
app-id-uri: ${WEB_API_ID_URI}
authorization-clients:
graph:
scopes:
- https://graph.microsoft.com/User.Read
-
Step 3: Write Java code:
Using @RegisteredOAuth2AuthorizedClient
to access related resource server:
public class SampleController {
@PreAuthorize("hasAuthority('SCOPE_Obo.Graph.Read')")
@GetMapping("call-graph")
public String callGraph(@RegisteredOAuth2AuthorizedClient("graph") OAuth2AuthorizedClient graph) {
return callMicrosoftGraphMeEndpoint(graph);
}
}
Web application and Resource server in one application
This scenario supports Web application
and Resource server
in one application.
To use aad-starter in this scenario, we need these steps:
-
Step 1: Add the following dependencies in you pom.xml.
<dependencies> <dependency> <groupId>com.azure.spring</groupId> <artifactId>spring-cloud-azure-starter-active-directory</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-resource-server</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-oauth2-client</artifactId> </dependency> </dependencies>
-
Step 2: Add properties in application.yml:
Set property spring.cloud.azure.active-directory.application-type
to web_application_and_resource_server
, and specify the authorization type for each authorization client.
spring:
cloud:
azure:
active-directory:
tenant-id: ${AZURE_TENANT_ID}
client-id: ${AZURE_CLIENT_ID}
client-secret: ${AZURE_CLIENT_SECRET}
app-id-uri: ${WEB_API_ID_URI}
application-type: web_application_and_resource_server # This is required.
authorization-clients:
graph:
authorizationGrantType: authorization_code # This is required.
scopes:
- https://graph.microsoft.com/User.Read
- https://graph.microsoft.com/Directory.Read.All
-
Step 3: Write Java code:
Configure multiple HttpSecurity instances, AADOAuth2SecurityMultiConfig
contain two security configurations for resource server and web application.
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled == true)
public class AADWebApplicationAndResourceServerConfig {
@Order(1)
@Configuration
public static class ApiWebSecurityConfigurationAdapter extends AADResourceServerWebSecurityConfigurerAdapter {
protected void configure(HttpSecurity http) throws Exception {
super.configure(http);
// All the paths that match `/api/**`(configurable) work as `Resource Server`, other paths work as `Web application`.
http.antMatcher("/api/**")
.authorizeRequests().anyRequest().authenticated();
}
}
@Configuration
public static class HtmlWebSecurityConfigurerAdapter extends AADWebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
super.configure(http);
// @formatter:off
http.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated();
// @formatter:on
}
}
}
11.2. Spring Security with Azure AD B2C
11.2.1. Dependency Setup
<dependencies>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-active-directory-b2c</artifactId>
</dependency>
</dependencies>
11.2.2. Configuration
Parameter | Description |
---|---|
|
Base uri for authorization server, if both |
|
The registered application ID in Azure AD B2C. |
|
The client secret of a registered application. |
|
A map to list all authorization clients created on Azure Portal. |
|
The key name of sign in user flow. |
|
The target URL after a successful logout. |
|
The Azure AD B2C’s tenant name, this is only suitable for Global cloud. |
|
The Azure AD B2C’s tenant id. |
|
A map to list all user flows defined on Azure Portal. |
|
The attribute name of the username. |
For full configurations, check appendix.
11.2.3. Basic Usage
A web application
is any web based application that allows user to login Azure AD, whereas a resource server
will either
accept or deny access after validating access_token obtained from Azure AD. We will cover 4 scenarios in this guide:
-
Accessing a web application.
-
Web application accessing resource servers.
-
Accessing a resource server.
-
Resource server accessing other resource servers.
Accessing a web application
This scenario uses The OAuth 2.0 authorization code grant flow to log in a user with your Azure AD B2C user.
-
Select Azure AD B2C from the portal menu, click Applications, and then click Add.
-
Specify your application Name, we call it
webapp
, addlocalhost:8080/login/oauth2/code/
for the Reply URL, record the Application ID as yourWEB_APP_AZURE_CLIENT_ID
and then click Save. -
Select Keys from your application, click Generate key to generate
WEB_APP_AZURE_CLIENT_SECRET
and then Save. -
Select User flows on your left, and then Click New user flow.
-
Choose Sign up or in, Profile editing and Password reset to create user flows respectively. Specify your user flow Name and User attributes and claims, click Create.
-
Select API permissions > Add a permission > Microsoft APIs, select Microsoft Graph, select Delegated permissions, check offline_access and openid permissions, select Add permission to complete the process.
-
Grant admin consent for Graph permissions.
-
Add the following dependencies in your pom.xml.
<dependencies>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>azure-spring-boot-starter-active-directory-b2c</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.thymeleaf.extras</groupId>
<artifactId>thymeleaf-extras-springsecurity5</artifactId>
</dependency>
</dependencies>
-
Add properties in application.yml using the values you created earlier, for example:
spring:
cloud:
azure:
active-directory:
b2c:
authenticate-additional-parameters:
domain_hint: xxxxxxxxx # optional
login_hint: xxxxxxxxx # optional
prompt: [login,none,consent] # optional
base-uri: ${BASE_URI}
client-id: ${WEBAPP_AZURE_CLIENT_ID}
client-secret: ${WEBAPP_AZURE_CLIENT_SECRET}
login-flow: ${LOGIN_USER_FLOW_KEY} # default to sign-up-or-sign-in, will look up the user-flows map with provided key.
logout-success-url: ${LOGOUT_SUCCESS_URL}
user-flows:
${YOUR_USER_FLOW_KEY}: ${USER_FLOW_NAME}
user-name-attribute-name: ${USER_NAME_ATTRIBUTE_NAME}
-
Write your Java code.
Controller code can refer to the following:
Security configuration code can refer to the following:
@EnableWebSecurity
public class WebSecurityConfiguration extends WebSecurityConfigurerAdapter {
private final AADB2COidcLoginConfigurer configurer;
public WebSecurityConfiguration(AADB2COidcLoginConfigurer configurer) {
this.configurer == configurer;
}
@Override
protected void configure(HttpSecurity http) throws Exception {
// @formatter:off
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.apply(configurer);
// @formatter:off
}
}
Copy the home.html from Azure AD B2C Spring Boot Sample, and replace the PROFILE_EDIT_USER_FLOW
and PASSWORD_RESET_USER_FLOW
with your user flow name respectively that completed earlier.
-
Build and test your app
Let Webapp
run on port 8080.
Web application accessing resource servers
This scenario is based on Accessing a web application scenario to allow application to access other resources, that is [The OAuth 2.0 client credentials grant] flow.
-
Select Azure AD B2C from the portal menu, click Applications, and then click Add.
-
Specify your application Name, we call it
webApiA
, record the Application ID as yourWEB_API_A_AZURE_CLIENT_ID
and then click Save. -
Select Keys from your application, click Generate key to generate
WEB_API_A_AZURE_CLIENT_SECRET
and then Save. -
Select Expose an API on your left, and then Click the Set link, record the Application ID URI as your
WEB_API_A_APP_ID_URL
, then Save. -
Select Manifest on your left, and then paste the below json segment into
appRoles
array, record the Application ID URI as yourWEB_API_A_APP_ID_URL
, record the value of the app role as yourWEB_API_A_ROLE_VALUE
, then save.
{
"allowedMemberTypes": [
"Application"
],
"description": "WebApiA.SampleScope",
"displayName": "WebApiA.SampleScope",
"id": "04989db0-3efe-4db6-b716-ae378517d2b7",
"isEnabled": true,
"value": "WebApiA.SampleScope"
}
-
Select API permissions > Add a permission > My APIs, select WebApiA application name, select Application Permissions, select WebApiA.SampleScope permission, select Add permission to complete the process.
-
Grant admin consent for WebApiA permissions.
-
Add the following dependency on the basis of Accessing a web application scenario.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
-
Add the following configuration on the basis of Accessing a web application scenario.
spring: cloud: azure: active-directory: b2c: base-uri: ${BASE_URI} # Such as: https://xxxxb2c.b2clogin.com tenant-id: ${AZURE_TENANT_ID} authorization-clients: ${RESOURCE_SERVER_A_NAME}: authorization-grant-type: client_credentials scopes: ${WEB_API_A_APP_ID_URL}/.default
-
Write your
Webapp
Java code.
Controller code can refer to the following:
Security configuration code is the same with Accessing a web application scenario, another bean `webClient`is added as follows:
public class SampleConfiguration {
@Bean
public WebClient webClient(OAuth2AuthorizedClientManager oAuth2AuthorizedClientManager) {
ServletOAuth2AuthorizedClientExchangeFilterFunction function =
new ServletOAuth2AuthorizedClientExchangeFilterFunction(oAuth2AuthorizedClientManager);
return WebClient.builder()
.apply(function.oauth2Configuration())
.build();
}
}
-
Please refer to Accessing a resource server section to write your
WebApiA
Java code. -
Build and test your app
Let Webapp
and WebApiA
run on port 8080 and 8081 respectively.
Start Webapp
and WebApiA
application, return to the home page after logging successfully, you can access localhost:8080/webapp/webApiA
to get WebApiA resource response.
Accessing a resource server
This scenario not support login. Just protect the server by validating the access token, and if valid, serves the request.
-
Refer to Web application accessing resource servers to build your
WebApiA
permission. -
Add
WebApiA
permission and grant admin consent for your web application. -
Add the following dependencies in your pom.xml.
<dependencies>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>azure-spring-boot-starter-active-directory-b2c</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
-
Add the following configuration.
spring: cloud: azure: active-directory: b2c: base-uri: ${BASE_URI} # Such as: https://xxxxb2c.b2clogin.com tenant-id: ${AZURE_TENANT_ID} app-id-uri: ${APP_ID_URI} # If you are using v1.0 token, please configure app-id-uri for `aud` verification client-id: ${AZURE_CLIENT_ID} # If you are using v2.0 token, please configure client-id for `aud` verification
-
Write your Java code.
Controller code can refer to the following:
Security configuration code can refer to the following:
@EnableWebSecurity
@EnableGlobalMethodSecurity(prePostEnabled == true)
public class ResourceServerConfiguration extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests((requests) -> requests.anyRequest().authenticated())
.oauth2ResourceServer()
.jwt()
.jwtAuthenticationConverter(new AADJwtBearerTokenAuthenticationConverter());
}
}
-
Build and test your app
Let WebApiA
run on port 8081.
Get the access token for webApiA
resource and access localhost:8081/webApiA/sample
as the Bearer authorization header.
Resource server accessing other resource servers
This scenario is an upgrade of Accessing a resource server, supports access to other application resources, based on OAuth2 client credentials flow.
-
Referring to the previous steps, we create a
WebApiB
application and expose an application permissionWebApiB.SampleScope
.
{ "allowedMemberTypes": [ "Application" ], "description": "WebApiB.SampleScope", "displayName": "WebApiB.SampleScope", "id": "04989db0-3efe-4db6-b716-ae378517d2b7", "isEnabled": true, "lang": null, "origin": "Application", "value": "WebApiB.SampleScope" }
-
Grant admin consent for WebApiB permissions.
-
On the basis of Accessing a resource server, add a dependency in your pom.xml.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
-
Add the following configuration on the basis of Accessing a resource server scenario configuration.
spring: cloud: azure: active-directory: b2c: client-secret: ${WEB_API_A_AZURE_CLIENT_SECRET} authorization-clients: ${RESOURCE_SERVER_B_NAME}: authorization-grant-type: client_credentials scopes: ${WEB_API_B_APP_ID_URL}/.default
-
Write your Java code.
WebApiA controller code can refer to the following:
WebApiB controller code can refer to the following:
public class SampleController {
/**
* webApiB resource api for other web application
* @return test content
*/
@PreAuthorize("hasAuthority('APPROLE_WebApiB.SampleScope')")
@GetMapping("/webApiB/sample")
public String webApiBSample() {
LOGGER.info("Call webApiBSample()");
return "Request '/webApiB/sample'(WebApi B) returned successfully.";
}
}
Security configuration code is the same with Accessing a resource server scenario, another bean `webClient`is added as follows
-
Build and test your app
Let WebApiA
and WebApiB
run on port 8081 and 8082 respectively.
Start WebApiA
and WebApiB
application, get the access token for webApiA
resource and access localhost:8081/webApiA/webApiB/sample
as the Bearer authorization header.
12. Spring Integration Support
Spring Integration Extension for Azure provides Spring Integration adapters for the various services provided by the Azure SDK for Java. Below is a list of supported adapters:
-
spring-cloud-azure-starter-integration-eventhbus
-
spring-cloud-azure-starter-integration-servicebus
-
spring-cloud-azure-starter-integration-storage-queue
Provide Spring Integration support for these Azure services: Event Hubs, Service Bus, Storage Queue.
12.1. Spring Integration with Azure Event Hubs
12.1.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-integration-eventhubs</artifactId>
</dependency>
12.1.2. Configuration
This starter provides the following 2 parts of configuration options:
Azure Common Configuration Options
Below properties can also be configured with the default Spring Cloud Azure unified properties by changing the prefix from spring.cloud.azure.eventhubs. to spring.cloud.azure..
Properties | Type | Description |
---|---|---|
spring.cloud.azure.eventhubs.enabled |
boolean |
Whether Azure Event Hubs service is enabled. |
spring.cloud.azure.eventhubs.credential.* |
NA |
Properties used for getting token credential. |
spring.cloud.azure.eventhubs.credential.clientId |
String |
Client id to use when performing service principal authentication with Azure. |
spring.cloud.azure.eventhubs.credential.clientSecret |
String |
Client secret to use when performing service principal authentication with Azure. |
spring.cloud.azure.eventhubs.credential.clientCertificatePath |
String |
Path of a PEM certificate file to use when performing service principal authentication with Azure. |
spring.cloud.azure.eventhubs.credential.clientCertificatePassword |
String |
Password of the certificate file. |
spring.cloud.azure.eventhubs.credential.username |
String |
Username to use when performing username/password authentication with Azure. |
spring.cloud.azure.eventhubs.credential.password |
String |
Password to use when performing username/password authentication with Azure. |
spring.cloud.azure.eventhubs.credential.managedIdentityClientId |
String |
Client id to use when using managed identity to authenticate with Azure. |
spring.cloud.azure.eventhubs.profile.* |
String |
Properties related to an Azure subscription. |
spring.cloud.azure.eventhubs.profile.tenantId |
String |
Tenant id for Azure resources. |
spring.cloud.azure.eventhubs.profile.subscriptionId |
String |
Subscription id to use when connecting to Azure resources. |
spring.cloud.azure.eventhubs.profile.cloud |
AzureProfileAware.CloudType |
Name of the Azure cloud to connect to. |
spring.cloud.azure.eventhubs.profile.environment.* |
NA |
Properties to Azure services, such as endpoints, resource ids, etc. |
spring.cloud.azure.eventhubs.profile.environment.activeDirectoryEndpoint |
String |
The Azure Active Directory endpoint to connect to. |
spring.cloud.azure.eventhubs.resource.* |
String |
Metadata defining an Azure resource. |
spring.cloud.azure.eventhubs.resource.resourceGroup |
String |
Name of the Azure resource group. |
spring.cloud.azure.eventhubs.resource.resourceId |
String |
Id of the Azure resource group. |
spring.cloud.azure.eventhubs.resource.region |
String |
Name of region. |
spring.cloud.azure.eventhubs.client.transportType |
AmqpTransportType |
Transport type switches available for AMQP protocol. |
spring.cloud.azure.eventhubs.retry.* |
NA |
Retry properties. |
spring.cloud.azure.eventhubs.retry.backoff.* |
NA |
Backoff properties when a retry fails. |
spring.cloud.azure.eventhubs.retry.backoff.delay |
Duration |
Amount of time to wait between retry attempts. |
spring.cloud.azure.eventhubs.retry.backoff.maxDelay |
Duration |
Maximum permissible amount of time between retry attempts. |
spring.cloud.azure.eventhubs.retry.backoff.multiplier |
Double |
Multiplier used to calculate the next backoff delay. If positive, then used as a multiplier for generating the next delay for backoff. |
spring.cloud.azure.eventhubs.retry.maxAttempts |
Integer |
The maximum number of attempts. |
spring.cloud.azure.eventhubs.retry.timeout |
Duration |
Amount of time to wait until a timeout. |
spring.cloud.azure.eventhubs.proxy.* |
NA |
Common proxy properties. |
spring.cloud.azure.eventhubs.proxy.type |
String |
Type of the proxy. |
spring.cloud.azure.eventhubs.proxy.hostname |
String |
The host of the proxy. |
spring.cloud.azure.eventhubs.proxy.port |
Integer |
The port of the proxy. |
spring.cloud.azure.eventhubs.proxy.authenticationType |
String |
Authentication type used against the proxy. |
spring.cloud.azure.eventhubs.proxy.username |
String |
Username used to authenticate with the proxy. |
spring.cloud.azure.eventhubs.proxy.password |
String |
Password used to authenticate with the proxy. |
Azure Event Hubs Client Configuration Options
Below options are used to configure Azure Event Hubs SDK Client.
Properties | Type | Description |
---|---|---|
spring.cloud.azure.eventhubs.connection-string |
String |
Event Hubs Namespace connection string value. |
spring.cloud.azure.eventhubs.namespace |
String |
Event Hubs Namespace value. |
spring.cloud.azure.eventhubs.domainName |
String |
Domain name of an Azure Event Hubs Namespace value. |
spring.cloud.azure.eventhubs.eventHubName |
String |
Name of an Event Hub entity. |
spring.cloud.azure.eventhubs.customEndpointAddress |
String |
Custom Endpoint address. |
spring.cloud.azure.eventhubs.isSharedConnection |
Boolean |
Whether to use the same connection for different Event Hub producer / consumer client. |
spring.cloud.azure.eventhubs.processor.checkpointStore.* |
NA |
Blob checkpoint store configuration options. |
spring.cloud.azure.eventhubs.processor.checkpointStore.createContainerIfNotExists |
Boolean |
If allowed to create container if not exists. |
spring.cloud.azure.eventhubs.processor.checkpointStore.customerProvidedKey |
String |
Base64 encoded string of the encryption key. |
spring.cloud.azure.eventhubs.processor.checkpointStore.encryptionScope |
String |
Encryption scope to encrypt blob contents on the server. |
spring.cloud.azure.eventhubs.processor.checkpointStore.serviceVersion |
BlobServiceVersion |
The versions of Azure Storage Blob supported by this client library. |
spring.cloud.azure.eventhubs.processor.checkpointStore.blobName |
String |
Storage blob name. |
spring.cloud.azure.eventhubs.processor.checkpointStore.containerName |
String |
Storage container name. |
12.1.3. Basic Usage
Send messages to Azure Event Hubs
Step 1. Fill the credential configuration options.
-
For credentials as connection string, configure below properties in
application.yml
:
spring: cloud: azure: eventhubs: connection-string: ${AZURE_SERVICE_BUS_CONNECTION_STRING}
-
For credentials as MSI, configure below properties in
application.yml
:
spring: cloud: azure: credential: managed-identity-client-id: ${AZURE_CLIENT_ID} profile: tenant-id: ${AZURE_TENANT_ID} # Uncomment below configurations if you want to enable auto creating resources. # subscription-id: ${AZURE_SUBSCRIPTION_ID} # cloud: Azure # resource: # region: [region] eventhubs: namespace: ${AZURE_SERVICE_BUS_NAMESPACE}
-
For credentials as service principal, configure below properties in application.yml:
spring: cloud: azure: credential: client-id: ${AZURE_CLIENT_ID} client-secret: ${AZURE_CLIENT_SECRET} profile: tenant-id: ${AZURE_TENANT_ID} # Uncomment below configurations if you want to enable auto creating resources. # subscription-id: ${AZURE_SUBSCRIPTION_ID} # cloud: Azure # resource: # region: [region] eventhubs: namespace: ${AZURE_SERVICE_BUS_NAMESPACE}
Step 2. Create DefaultMessageHandler
with the bean of EventHubsTemplate
to send messages to Event Hubs.
private static final String OUTPUT_CHANNEL = "output";
private static final String EVENTHUB_NAME = "eh1";
@Bean
@ServiceActivator(inputChannel = OUTPUT_CHANNEL)
public MessageHandler messageSender(EventHubsTemplate queueOperation) {
DefaultMessageHandler handler = new DefaultMessageHandler(EVENTHUB_NAME, queueOperation);
handler.setSendCallback(new ListenableFutureCallback<Void>() {
@Override
public void onSuccess(Void result) {
LOGGER.info("Message was sent successfully.");
}
@Override
public void onFailure(Throwable ex) {
LOGGER.error("There was an error sending the message.", ex);
}
});
return handler;
}
Step 3. Create a Message gateway binding with the message handler created in the last step via a message channel
@Autowired
EventHubOutboundGateway messagingGateway;
@MessagingGateway(defaultRequestChannel = OUTPUT_CHANNEL)
public interface EventHubOutboundGateway {
void send(String text);
}
Step 4. Send messages using the gateway
this.messagingGateway.send(message);
Receive messages from Azure Event Hubs
Step 1. Fill the credential configuration options.
Step 2. Create a bean of message channel as the input channel.
private static final String INPUT_CHANNEL = "input";
private static final String EVENTHUB_NAME = "eh1";
private static final String CONSUMER_GROUP = "$Default";
@Bean
public MessageChannel input() {
return new DirectChannel();
}
Step 3. Create EventHubsInboundChannelAdapter
with the bean of EventHubsProcessorContainer
to receive messages to Event Hubs.
@Bean
public EventHubsInboundChannelAdapter messageChannelAdapter(
@Qualifier(INPUT_CHANNEL) MessageChannel inputChannel,
EventHubsProcessorContainer processorContainer) {
CheckpointConfig config = new CheckpointConfig(CheckpointMode.MANUAL);
EventHubsInboundChannelAdapter adapter =
new EventHubsInboundChannelAdapter(processorContainer, EVENTHUB_NAME,
CONSUMER_GROUP, config);
adapter.setOutputChannel(inputChannel);
return adapter;
}
Step 4. Create a message receiver binding with EventHubsInboundChannelAdapter created in the last step via the message channel we created before.
@ServiceActivator(inputChannel = INPUT_CHANNEL)
public void messageReceiver(byte[] payload, @Header(AzureHeaders.CHECKPOINTER) Checkpointer checkpointer) {
String message = new String(payload);
LOGGER.info("New message received: '{}'", message);
checkpointer.success()
.doOnSuccess(s -> LOGGER.info("Message '{}' successfully checkpointed", message))
.doOnError(e -> LOGGER.error("Error found", e))
.subscribe();
}
12.1.4. Samples
Please refer to this sample project to learn how to use Event Hubs integration.
12.2. Spring Integration with Azure Service Bus
12.2.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-integration-servicebus</artifactId>
</dependency>
12.2.2. Configuration
Azure Common Configuration Options
Below properties can also be configured with the default Spring Cloud Azure unified properties by changing the prefix from spring.cloud.azure.servicebus. to spring.cloud.azure..
Properties | Type | Description |
---|---|---|
spring.cloud.azure.servicebus.enabled |
boolean |
Whether an Azure Service Bus is enabled. |
spring.cloud.azure.servicebus.credential.* |
NA |
Properties used for getting token credential. |
spring.cloud.azure.servicebus.credential.clientId |
String |
Client id to use when performing service principal authentication with Azure. |
spring.cloud.azure.servicebus.credential.clientSecret |
String |
Client secret to use when performing service principal authentication with Azure. |
spring.cloud.azure.servicebus.credential.clientCertificatePath |
String |
Path of a PEM certificate file to use when performing service principal authentication with Azure. |
spring.cloud.azure.servicebus.credential.clientCertificatePassword |
String |
Password of the certificate file. |
spring.cloud.azure.servicebus.credential.username |
String |
Username to use when performing username/password authentication with Azure. |
spring.cloud.azure.servicebus.credential.password |
String |
Password to use when performing username/password authentication with Azure. |
spring.cloud.azure.servicebus.credential.managedIdentityClientId |
String |
Client id to use when using managed identity to authenticate with Azure. |
spring.cloud.azure.servicebus.profile.* |
String |
Properties related to an Azure subscription. |
spring.cloud.azure.servicebus.profile.tenantId |
String |
Tenant id for Azure resources. |
spring.cloud.azure.servicebus.profile.subscriptionId |
String |
Subscription id to use when connecting to Azure resources. |
spring.cloud.azure.servicebus.profile.cloud |
AzureProfileAware.CloudType |
Name of the Azure cloud to connect to. |
spring.cloud.azure.servicebus.profile.environment.* |
NA |
Properties to Azure services, such as endpoints, resource ids, etc. |
spring.cloud.azure.servicebus.profile.environment.activeDirectoryEndpoint |
String |
The Azure Active Directory endpoint to connect to. |
spring.cloud.azure.servicebus.resource.* |
String |
Metadata defining an Azure resource. |
spring.cloud.azure.servicebus.resource.resourceGroup |
String |
Name of the Azure resource group. |
spring.cloud.azure.servicebus.resource.resourceId |
String |
Id of the Azure resource group. |
spring.cloud.azure.servicebus.resource.region |
String |
Name of region. |
spring.cloud.azure.servicebus.client.transportType |
AmqpTransportType |
Transport type switches available for AMQP protocol. |
spring.cloud.azure.servicebus.retry.* |
NA |
Retry properties. |
spring.cloud.azure.servicebus.retry.backoff.* |
NA |
Backoff properties when a retry fails. |
spring.cloud.azure.servicebus.retry.backoff.delay |
Duration |
Amount of time to wait between retry attempts. |
spring.cloud.azure.servicebus.retry.backoff.maxDelay |
Duration |
Maximum permissible amount of time between retry attempts. |
spring.cloud.azure.servicebus.retry.backoff.multiplier |
Double |
Multiplier used to calculate the next backoff delay. If positive, then used as a multiplier for generating the next delay for backoff. |
spring.cloud.azure.servicebus.retry.maxAttempts |
Integer |
The maximum number of attempts. |
spring.cloud.azure.servicebus.retry.timeout |
Duration |
Amount of time to wait until a timeout. |
spring.cloud.azure.servicebus.proxy.* |
NA |
Common proxy properties. |
spring.cloud.azure.servicebus.proxy.type |
String |
Type of the proxy. |
spring.cloud.azure.servicebus.proxy.hostname |
String |
The host of the proxy. |
spring.cloud.azure.servicebus.proxy.port |
Integer |
The port of the proxy. |
spring.cloud.azure.servicebus.proxy.authenticationType |
String |
Authentication type used against the proxy. |
spring.cloud.azure.servicebus.proxy.username |
String |
Username used to authenticate with the proxy. |
spring.cloud.azure.servicebus.proxy.password |
String |
Password used to authenticate with the proxy. |
Azure Service Bus Client Configuration Options
Below options are used to configure Azure Service Bus SDK Client.
Properties | Type | Description |
---|---|---|
spring.cloud.azure.servicebus.connection-string |
String |
Service Bus Namespace connection string value. |
spring.cloud.azure.servicebus.namespace |
String |
Service Bus Namespace value. |
spring.cloud.azure.servicebus.domainName |
String |
Domain name of an Azure Service Bus Namespace value. |
spring.cloud.azure.servicebus.entityName |
String |
Entity name of Azure Service Bus queue or topic. |
spring.cloud.azure.servicebus.entityType |
ServiceBusEntityType |
Entity type of Azure Service Bus queue or topic. |
spring.cloud.azure.servicebus.crossEntityTransactions |
Boolean |
Enable cross entity transaction on the connection to Service bus. |
12.2.3. Basic Usage
Send messages to Azure Service Bus
Step 1. Fill the credential configuration options.
-
For credentials as connection string, configure below properties in application.yml:
spring: cloud: azure: servicebus: connection-string: ${AZURE_SERVICE_BUS_CONNECTION_STRING}
-
For credentials as MSI, configure below properties in application.yml:
spring: cloud: azure: credential: managed-identity-client-id: ${AZURE_CLIENT_ID} profile: tenant-id: ${AZURE_TENANT_ID} # Uncomment below configurations if you want to enable auto creating resources. # subscription-id: ${AZURE_SUBSCRIPTION_ID} # cloud: Azure # resource: # region: [region] servicebus: namespace: ${AZURE_SERVICE_BUS_NAMESPACE}
-
For credentials as service principal, configure below properties in application.yml:
spring: cloud: azure: credential: client-id: ${AZURE_CLIENT_ID} client-secret: ${AZURE_CLIENT_SECRET} profile: tenant-id: ${AZURE_TENANT_ID} # Uncomment below configurations if you want to enable auto creating resources. # subscription-id: ${AZURE_SUBSCRIPTION_ID} # cloud: Azure # resource: # region: [region] servicebus: namespace: ${AZURE_SERVICE_BUS_NAMESPACE}
Step 2. Create DefaultMessageHandler
with the bean of ServiceBusTemplate
to send messages to Service Bus,
set the entity type for the ServiceBusTemplate.
private static final String OUTPUT_CHANNEL = "queue.output";
@Bean
@ServiceActivator(inputChannel = OUTPUT_CHANNEL)
public MessageHandler queueMessageSender(ServiceBusTemplate serviceBusTemplate) {
serviceBusTemplate.setDefaultEntityType(ServiceBusEntityType.QUEUE);
DefaultMessageHandler handler = new DefaultMessageHandler(QUEUE_NAME, serviceBusTemplate);
handler.setSendCallback(new ListenableFutureCallback<Void>() {
@Override
public void onSuccess(Void result) {
LOGGER.info("Message was sent successfully.");
}
@Override
public void onFailure(Throwable ex) {
LOGGER.info("There was an error sending the message.");
}
});
return handler;
}
Step 3. Create a Message gateway binding with the message handler created in the last stop via a message channel
@Autowired
QueueOutboundGateway messagingGateway;
@MessagingGateway(defaultRequestChannel = OUTPUT_CHANNEL)
public interface QueueOutboundGateway {
void send(String text);
}
Step 4. Send messages using the gateway
this.messagingGateway.send(message);
Receive messages from Azure Service Bus
Step 1. Fill the credential configuration options.
Step 2. Create a bean of message channel as the input channel.
private static final String INPUT_CHANNEL = "input";
@Bean
public MessageChannel input() {
return new DirectChannel();
}
Step 3. Create ServiceBusInboundChannelAdapter
with the bean of ServiceBusProcessorContainer
to receive messages to Service Bus.
private static final String QUEUE_NAME = "queue1";
@Bean
public ServiceBusInboundChannelAdapter queueMessageChannelAdapter(
@Qualifier(INPUT_CHANNEL) MessageChannel inputChannel, ServiceBusProcessorContainer processorContainer) {
ServiceBusInboundChannelAdapter adapter = new ServiceBusInboundChannelAdapter(processorContainer, QUEUE_NAME,
new CheckpointConfig(CheckpointMode.MANUAL));
adapter.setOutputChannel(inputChannel);
return adapter;
}
Step 4. Create a message receiver binding with ServiceBusInboundChannelAdapter created in the last step via the message channel we created before.
@ServiceActivator(inputChannel = INPUT_CHANNEL)
public void messageReceiver(byte[] payload, @Header(AzureHeaders.CHECKPOINTER) Checkpointer checkpointer) {
String message = new String(payload);
LOGGER.info("New message received: '{}'", message);
checkpointer.success()
.doOnSuccess(s -> LOGGER.info("Message '{}' successfully checkpointed", message))
.doOnError(e -> LOGGER.error("Error found", e))
.subscribe();
}
12.2.4. Samples
Please refer to this sample project to learn how to use Service Bus integration.
Example: Manually set the partition key for the message
This example demonstrates how to manually set the partition key for the message in the application.
Recommended: Use ServiceBusMessageHeaders.PARTITION_KEY
as the key of the header.
public class SampleController {
@PostMapping("/messages")
public ResponseEntity<String> sendMessage(@RequestParam String message) {
LOGGER.info("Going to add message {} to Sinks.Many.", message);
many.emitNext(MessageBuilder.withPayload(message)
.setHeader(ServiceBusMessageHeaders.PARTITION_KEY, "Customize partition key")
.build(), Sinks.EmitFailureHandler.FAIL_FAST);
return ResponseEntity.ok("Sent!");
}
}
Not recommended but currently supported: AzureHeaders.PARTITION_KEY
as the key of the header.
public class SampleController {
@PostMapping("/messages")
public ResponseEntity<String> sendMessage(@RequestParam String message) {
LOGGER.info("Going to add message {} to Sinks.Many.", message);
many.emitNext(MessageBuilder.withPayload(message)
.setHeader(AzureHeaders.PARTITION_KEY, "Customize partition key")
.build(), Sinks.EmitFailureHandler.FAIL_FAST);
return ResponseEntity.ok("Sent!");
}
}
When both ServiceBusMessageHeaders.PARTITION_KEY and AzureHeaders.PARTITION_KEY are set in the message headers,
ServiceBusMessageHeaders.PARTITION_KEY is preferred.
|
Example: Set the session id for the message
This example demonstrates how to manually set the session id of a message in the application.
public class SampleController {
@PostMapping("/messages")
public ResponseEntity<String> sendMessage(@RequestParam String message) {
LOGGER.info("Going to add message {} to Sinks.Many.", message);
many.emitNext(MessageBuilder.withPayload(message)
.setHeader(ServiceBusMessageHeaders.SESSION_ID, "Customize session id")
.build(), Sinks.EmitFailureHandler.FAIL_FAST);
return ResponseEntity.ok("Sent!");
}
}
When the ServiceBusMessageHeaders.SESSION_ID is set in the message headers, and a different ServiceBusMessageHeaders.PARTITION_KEY (or AzureHeaders.PARTITION_KEY ) header is also set,
the value of the session id will eventually be used to overwrite the value of the partition key.
|
12.3. Spring Integration with Azure Storage Queue
12.3.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-integration-storage-queue</artifactId>
</dependency>
12.3.2. Configuration
Azure Common Configuration Options
Below properties can also be configured with the default Spring Cloud Azure unified properties, by changing the prefix from spring.cloud.azure.storage.queue. to spring.cloud.azure..
Properties | Type | Description |
---|---|---|
spring.cloud.azure.storage.queue.enabled |
boolean |
Whether an Azure Storage Queue is enabled. |
spring.cloud.azure.storage.queue.credential.* |
NA |
Properties used for getting token credential. |
spring.cloud.azure.storage.queue.credential.clientId |
String |
Client id to use when performing service principal authentication with Azure. |
spring.cloud.azure.storage.queue.credential.clientSecret |
String |
Client secret to use when performing service principal authentication with Azure. |
spring.cloud.azure.storage.queue.credential.clientCertificatePath |
String |
Path of a PEM certificate file to use when performing service principal authentication with Azure. |
spring.cloud.azure.storage.queue.credential.clientCertificatePassword |
String |
Password of the certificate file. |
spring.cloud.azure.storage.queue.credential.username |
String |
Username to use when performing username/password authentication with Azure. |
spring.cloud.azure.storage.queue.credential.password |
String |
Password to use when performing username/password authentication with Azure. |
spring.cloud.azure.storage.queue.credential.managedIdentityClientId |
String |
Client id to use when using managed identity to authenticate with Azure. |
spring.cloud.azure.storage.queue.profile.* |
String |
Properties related to an Azure subscription. |
spring.cloud.azure.storage.queue.profile.tenantId |
String |
Tenant id for Azure resources. |
spring.cloud.azure.storage.queue.profile.subscriptionId |
String |
Subscription id to use when connecting to Azure resources. |
spring.cloud.azure.storage.queue.profile.cloud |
AzureProfileAware.CloudType |
Name of the Azure cloud to connect to. |
spring.cloud.azure.storage.queue.profile.environment.* |
NA |
Properties to Azure services, such as endpoints, resource ids, etc. |
spring.cloud.azure.storage.queue.profile.environment.activeDirectoryEndpoint |
String |
The Azure Active Directory endpoint to connect to. |
spring.cloud.azure.storage.queue.resource.* |
String |
Metadata defining an Azure resource. |
spring.cloud.azure.storage.queue.resource.resourceGroup |
String |
Name of the Azure resource group. |
spring.cloud.azure.storage.queue.resource.resourceId |
String |
Id of the Azure resource group. |
spring.cloud.azure.storage.queue.resource.region |
String |
Name of region. |
spring.cloud.azure.storage.queue.client.transportType |
AmqpTransportType |
Transport type switches available for AMQP protocol. |
spring.cloud.azure.storage.queue.retry.* |
NA |
Retry properties. |
spring.cloud.azure.storage.queue.retry.backoff.* |
NA |
Backoff properties when a retry fails. |
spring.cloud.azure.storage.queue.retry.backoff.delay |
Duration |
Amount of time to wait between retry attempts. |
spring.cloud.azure.storage.queue.retry.backoff.maxDelay |
Duration |
Maximum permissible amount of time between retry attempts. |
spring.cloud.azure.storage.queue.retry.backoff.multiplier |
Double |
Multiplier used to calculate the next backoff delay. If positive, then used as a multiplier for generating the next delay for backoff. |
spring.cloud.azure.storage.queue.retry.maxAttempts |
Integer |
The maximum number of attempts. |
spring.cloud.azure.storage.queue.retry.timeout |
Duration |
Amount of time to wait until a timeout. |
spring.cloud.azure.storage.queue.proxy.* |
NA |
Common proxy properties. |
spring.cloud.azure.storage.queue.proxy.type |
String |
Type of the proxy. |
spring.cloud.azure.storage.queue.proxy.hostname |
String |
The host of the proxy. |
spring.cloud.azure.storage.queue.proxy.port |
Integer |
The port of the proxy. |
spring.cloud.azure.storage.queue.proxy.authenticationType |
String |
Authentication type used against the proxy. |
spring.cloud.azure.storage.queue.proxy.username |
String |
Username used to authenticate with the proxy. |
spring.cloud.azure.storage.queue.proxy.password |
String |
Password used to authenticate with the proxy. |
Azure Storage Queue Client Configuration Options
Below options are used to configure Azure Storage Queue SDK Client.
Properties | Type | Description |
---|---|---|
spring.cloud.azure.storage.queue.connection-string |
String |
Storage Queue Namespace connection string value. |
spring.cloud.azure.storage.queue.accountName |
String |
Storage Queue account name. |
spring.cloud.azure.storage.queue.accountKey |
String |
Storage Queue account key. |
spring.cloud.azure.storage.queue.endpoint |
String |
Storage Queue service endpoint. |
spring.cloud.azure.storage.queue.sasToken |
String |
Sas token credential |
spring.cloud.azure.storage.queue.serviceVersion |
QueueServiceVersion |
QueueServiceVersion that is used when making API requests. |
spring.cloud.azure.storage.queue.messageEncoding |
String |
Queue message encoding. |
12.3.3. Basic Usage
Send messages to Azure Storage Queue
Step 1. Fill the credential configuration options.
-
For credentials as connection string, configure below properties in application.yml:
spring: cloud: azure: storage: queue: connection-string: ${AZURE_SERVICE_BUS_CONNECTION_STRING}
-
For credentials as MSI, configure below properties in application.yml:
spring: cloud: azure: credential: managed-identity-client-id: ${AZURE_CLIENT_ID} profile: tenant-id: ${AZURE_TENANT_ID} # Uncomment below configurations if you want to enable auto creating resources. # subscription-id: ${AZURE_SUBSCRIPTION_ID} # cloud: Azure # resource: # region: [region] storage: queue: namespace: ${AZURE_SERVICE_BUS_NAMESPACE}
-
For credentials as service principal, configure below properties in application.yml:
spring: cloud: azure: credential: client-id: ${AZURE_CLIENT_ID} client-secret: ${AZURE_CLIENT_SECRET} profile: tenant-id: ${AZURE_TENANT_ID} # Uncomment below configurations if you want to enable auto creating resources. # subscription-id: ${AZURE_SUBSCRIPTION_ID} # cloud: Azure # resource: # region: [region] storage: queue: namespace: ${AZURE_SERVICE_BUS_NAMESPACE}
Step 2. Create DefaultMessageHandler
with the bean of StorageQueueOperation
to send messages to Storage Queue.
private static final String STORAGE_QUEUE_NAME = "example";
private static final String OUTPUT_CHANNEL = "output";
@Bean
@ServiceActivator(inputChannel = OUTPUT_CHANNEL)
public MessageHandler messageSender(StorageQueueOperation storageQueueOperation) {
DefaultMessageHandler handler = new DefaultMessageHandler(STORAGE_QUEUE_NAME, storageQueueOperation);
handler.setSendCallback(new ListenableFutureCallback<Void>() {
@Override
public void onSuccess(Void result) {
LOGGER.info("Message was sent successfully.");
}
@Override
public void onFailure(Throwable ex) {
LOGGER.info("There was an error sending the message.");
}
});
return handler;
}
Step 3. Create a Message gateway binding with the message handler created in the last stop via a message channel
@Autowired
StorageQueueOutboundGateway storageQueueOutboundGateway;
@MessagingGateway(defaultRequestChannel = OUTPUT_CHANNEL)
public interface StorageQueueOutboundGateway {
void send(String text);
}
Step 4. Send messages using the gateway
this.storageQueueOutboundGateway.send(message);
Receive messages from Azure Storage Queue
Step 1. Fill the credential configuration options.
Step 2. Create a bean of message channel as the input channel.
private static final String INPUT_CHANNEL = "input";
@Bean
public MessageChannel input() {
return new DirectChannel();
}
Step 3. Create StorageQueueMessageSource
with the bean of StorageQueueOperation
to receive messages to Storage Queue.
private static final String STORAGE_QUEUE_NAME = "example";
@Bean
@InboundChannelAdapter(channel = INPUT_CHANNEL, poller = @Poller(fixedDelay = "1000"))
public StorageQueueMessageSource storageQueueMessageSource(StorageQueueOperation storageQueueOperation) {
storageQueueOperation.setCheckpointMode(CheckpointMode.MANUAL);
storageQueueOperation.setVisibilityTimeoutInSeconds(10);
return new StorageQueueMessageSource(STORAGE_QUEUE_NAME, storageQueueOperation);
}
Step 4. Create a message receiver binding with StorageQueueMessageSource created in the last step via the message channel we created before.
@ServiceActivator(inputChannel = INPUT_CHANNEL)
public void messageReceiver(byte[] payload, @Header(AzureHeaders.CHECKPOINTER) Checkpointer checkpointer) {
String message = new String(payload);
LOGGER.info("New message received: '{}'", message);
checkpointer.success()
.doOnError(Throwable::printStackTrace)
.doOnSuccess(t -> LOGGER.info("Message '{}' successfully checkpointed", message))
.subscribe();
}
12.3.4. Samples
Please refer to this sample project illustrating how to use Storage Queue integration.
13. Spring Cloud Stream Support
Spring Cloud Stream is a framework for building highly scalable event-driven microservices connected with shared messaging systems.
The framework provides a flexible programming model built on already established and familiar Spring idioms and best practices, including support for persistent pub/sub semantics, consumer groups, and stateful partitions.
Current binder implementations include:
-
spring-cloud-azure-stream-binder-eventhubs
-
spring-cloud-azure-stream-binder-servicebus
13.1. Spring Cloud Stream Binder for Azure Event Hubs
13.1.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-stream-binder-eventhubs</artifactId>
</dependency>
13.1.2. Configuration
The binder provides the following configuration options in application.properties
.
Spring Cloud Azure Properties
Name | Description | Required | Default |
---|---|---|---|
spring.cloud.azure.auto-create-resources |
If enable auto-creation for Azure resources |
false |
|
spring.cloud.azure.region |
Region name of the Azure resource group, e.g. westus |
Yes if spring.cloud.azure.auto-create-resources is enabled. |
spring.cloud.azure.environment |
Azure Cloud name for Azure resources, supported values are |
azure |
spring.cloud.azure.client-id |
|
Client (application) id of a service principal or Managed Service Identity (MSI) |
Yes if service principal or MSI is used as credential configuration. |
spring.cloud.azure.client-secret |
Client secret of a service principal |
Yes if service principal is used as credential configuration. |
spring.cloud.azure.msi-enabled |
If enable MSI as credential configuration |
Yes if MSI is used as credential configuration. |
false |
spring.cloud.azure.resource-group |
Name of Azure resource group |
Yes if service principal or MSI is used as credential configuration. |
spring.cloud.azure.subscription-id |
Subscription id of an MSI |
Yes if MSI is used as credential configuration. |
spring.cloud.azure.tenant-id |
Tenant id of a service principal |
Yes if service principal is used as credential configuration. |
spring.cloud.azure.eventhub.connection-string |
Event Hubs Namespace connection string |
Yes if connection string is used as Event Hubs credential configuration |
spring.cloud.azure.eventhub.checkpoint-storage-account |
StorageAccount name for message checkpoint |
Yes |
spring.cloud.azure.eventhub.checkpoint-access-key |
StorageAccount access key for message checkpoint |
Yes if StorageAccount access key is used as StorageAccount credential configuration |
spring.cloud.azure.eventhub.checkpoint-container |
StorageAccount container name for message checkpoint |
Yes |
spring.cloud.azure.eventhub.namespace |
Event Hub Namespace. Auto creating if missing |
Common Producer Properties
You can use the producer configurations of Spring Cloud Stream,
it uses the configuration with the format of spring.cloud.stream.bindings.<channelName>.producer
.
Partition configuration
The system will obtain the parameter PartitionSupply
to send the message,
the following is the process of obtaining the priority of the partition ID and key:
The following are configuration items related to the producer:
PropertyName | Default Value | Description |
---|---|---|
partition-count |
1 |
The number of target partitions for the data, if partitioning is enabled. |
partition-key-extractor-name |
null |
The name of the bean that implements |
partition-key-expression |
null |
A SpEL expression that determines how to partition outbound data.
When interface |
For more information about setting partition for the producer properties, please refer to the Producer Properties of Spring Cloud Stream.
Event Hub Producer Properties
It supports the following configurations with the format of spring.cloud.stream.eventhubs.bindings.<channelName>.producer
.
PropertyName | Default Value | Description |
---|---|---|
sync |
false |
Whether the producer should act in a synchronous manner with respect to writing messages into a stream. If true, the producer will wait for a response from Event Hub after a send operation. |
send-timeout |
10000 |
Effective only if |
Common Consumer Properties
You can use the below consumer configurations of Spring Cloud Stream,
it uses the configuration with the format of spring.cloud.stream.bindings.<channelName>.consumer
.
Batch Consumer
When spring.cloud.stream.bindings.<binding-name>.consumer.batch-mode
is set to true
, all of the received events
will be presented as a List<?>
to the consumer function. Otherwise, the function will be called with one event at a time.
The size of the batch is controlled by Event Hubs consumer properties max-size
(required) and max-wait-time
(optional); refer to the below section for more information.
batch-mode
Whether to enable the entire batch of messages to be passed to the consumer function in a List
.
Default: False
Event Hub Consumer Properties
It supports the following configurations with the format of spring.cloud.stream.eventhubs.bindings.<channelName>.consumer
.
start-position
Whether the consumer receives messages from the beginning or end of event hub. if EARLIEST
, from beginning. If
LATEST
, from end.
Default: LATEST
checkpoint-mode
The mode in which checkpoints are updated.
RECORD
, default
mode. Checkpoints occur after each record is successfully processed by user-defined message
handler without any exception. If you use StorageAccount
as checkpoint store, this might become bottleneck.
BATCH
, checkpoints occur after each batch of messages successfully processed by user-defined message handler
without any exception. Be aware that batch size could be any value and BATCH
mode is only supported when consume
batch
mode is set true.
MANUAL
, checkpoints occur on demand by the user via the Checkpointer
. You can do checkpoints after the message has been successfully processed. Message.getHeaders.get(AzureHeaders.CHECKPOINTER)`callback can get you the `Checkpointer
you need. Please be aware all messages in the corresponding Event Hub partition before this message will be considered as successfully processed.
PARTITION_COUNT
, checkpoints occur after the count of messages defined by checkpoint_count
successfully processed for each partition. You may experience reprocessing at most checkpoint_count
of when message processing fails.
Time
, checkpoints occur at fixed time interval specified by checkpoint_interval
. You may experience reprocessing of messages during this time interval when message processing fails.
Default: RECORD
when consume batch mode is false(default value), BATCH checkpoint mode is not invalid.
|
checkpoint-count
Effectively only when checkpoint-mode
is PARTITION_COUNT
. Decides the amount of message for each partition to do one checkpoint.
Default: 10
checkpoint-interval
Effectively only when checkpoint-mode
is Time
. Decides The time interval to do one checkpoint.
Default: 5s
max-size
The maximum number of events that will be in the list of a message payload when the consumer callback is invoked.
Default: 10
max-wait-time
The max time Duration
to wait to receive a batch of events up to the max batch size before invoking the consumer callback.
Default: null
To see the list of all Spring Cloud Azure related configuration properties please check the Appendix page.
13.1.5. Error Channels
consumer error channel
this channel is open by default, you can handle the error message in this way:
// Replace destination with spring.cloud.stream.bindings.input.destination // Replace group with spring.cloud.stream.bindings.input.group @ServiceActivator(inputChannel == "{destination}.{group}.errors") public void consumerError(Message<?> message) { LOGGER.error("Handling customer ERROR: " + message); }
producer error channel
this channel is not open by default, if you want to open it. You need to add a configuration in your application.properties, like this:
spring.cloud.stream.default.producer.errorChannelEnabled=true
you can handle the error message in this way:
// Replace destination with spring.cloud.stream.bindings.output.destination @ServiceActivator(inputChannel == "{destination}.errors") public void producerError(Message<?> message) { LOGGER.error("Handling Producer ERROR: " + message); }
Batch Consumer Sample
Configuration Options
To enable the batch consumer mode, you should add below configuration
spring:
cloud:
stream:
bindings:
consume-in-0:
destination: ${AZURE_EVENTHUB_NAME}
group: ${AZURE_EVENTHUB_CONSUMER_GROUP}
consumer:
batch-mode: true
eventhubs:
bindings:
consume-in-0:
consumer:
checkpoint:
mode: BATCH # or MANUAL as needed
batch:
max-size: 2 # The default value is 10
max-wait-time: 1m # Optional, the default value is null
Consume messages in batches
For checkpointing mode as BATCH, you can use below code to send messages and consume in batches.
@Bean
public Consumer<List<String>> consume() {
return list -> list.forEach(event -> LOGGER.info("New event received: '{}'",event));
}
@Bean
public Supplier<Message<String>> supply() {
return () -> {
LOGGER.info("Sending message, sequence " + i);
return MessageBuilder.withPayload("\"test"+ i++ +"\"").build();
};
}
For checkpointing mode as MANUAL, you can use below code to send messages and consume/checkpoint in batches.
@Bean
public Consumer<Message<List<String>>> consume() {
return message -> {
for (int i == 0; i < message.getPayload().size(); i++) {
LOGGER.info("New message received: '{}', partition key: {}, sequence number: {}, offset: {}, enqueued time: {}",
message.getPayload().get(i),
((List<Object>) message.getHeaders().get(EventHubsHeaders.PARTITION_KEY)).get(i),
((List<Object>) message.getHeaders().get(EventHubsHeaders.SEQUENCE_NUMBER)).get(i),
((List<Object>) message.getHeaders().get(EventHubsHeaders.OFFSET)).get(i),
((List<Object>) message.getHeaders().get(EventHubsHeaders.ENQUEUED_TIME)).get(i));
}
Checkpointer checkpointer == (Checkpointer) message.getHeaders().get(CHECKPOINTER);
checkpointer.success()
.doOnSuccess(success -> LOGGER.info("Message '{}' successfully checkpointed", message.getPayload()))
.doOnError(error -> LOGGER.error("Exception found", error))
.subscribe();
};
}
@Bean
public Supplier<Message<String>> supply() {
return () -> {
LOGGER.info("Sending message, sequence " + i);
return MessageBuilder.withPayload("\"test"+ i++ +"\"").build();
};
}
13.2. Spring Cloud Stream Binder for Azure Service Bus
13.2.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-stream-binder-servicebus</artifactId>
</dependency>
13.2.2. Configuration
The binder provides the following configuration options:
Spring Cloud Azure Properties
Name | Description | Required | Default |
---|---|---|---|
spring.cloud.azure.auto-create-resources |
If enable auto-creation for Azure resources |
false |
|
spring.cloud.azure.region |
Region name of the Azure resource group, e.g. westus |
Yes if spring.cloud.azure.auto-create-resources is enabled. |
spring.cloud.azure.environment |
Azure Cloud name for Azure resources, supported values are |
azure |
spring.cloud.azure.client-id |
|
Client (application) id of a service principal or Managed Service Identity (MSI) |
Yes if service principal or MSI is used as credential configuration. |
spring.cloud.azure.client-secret |
Client secret of a service principal |
Yes if service principal is used as credential configuration. |
spring.cloud.azure.msi-enabled |
If enable MSI as credential configuration |
Yes if MSI is used as credential configuration. |
false |
spring.cloud.azure.resource-group |
Name of Azure resource group |
Yes if service principal or MSI is used as credential configuration. |
spring.cloud.azure.subscription-id |
Subscription id of an MSI |
Yes if MSI is used as credential configuration. |
spring.cloud.azure.tenant-id |
Tenant id of a service principal |
Yes if service principal is used as credential configuration. |
spring.cloud.azure.servicebus.connection-string |
Service Bus Namespace connection string |
Yes if connection string is used as credential configuration |
spring.cloud.azure.servicebus.namespace |
Service Bus Namespace. Auto creating if missing |
Yes if service principal or MSI is used as credential configuration. |
spring.cloud.azure.servicebus.transportType |
Service Bus transportType, supported value of |
No |
|
spring.cloud.azure.servicebus.retry-Options |
Service Bus retry options |
No |
Default value of AmqpRetryOptions |
Partition configuration
The system will obtain the parameter PartitionSupply
to send the message.
The following are configuration items related to the producer:
partition-count
The number of target partitions for the data, if partitioning is enabled.
Default: 1
partition-key-extractor-name
The name of the bean that implements PartitionKeyExtractorStrategy
.
The partition handler will first use the PartitionKeyExtractorStrategy#extractKey
method to obtain the partition key value.
Default: null
partition-key-expression
A SpEL expression that determines how to partition outbound data.
When interface PartitionKeyExtractorStrategy
is not implemented, it will be called in the method PartitionHandler#extractKey
.
Default: null
For more information about setting partition for the producer properties, please refer to the Producer Properties of Spring Cloud Stream.
Serivce Bus Queue Producer Properties
It supports the following configurations with the format of spring.cloud.stream.servicebus.queue.bindings.<channelName>.producer
.
sync
Whether the producer should act in a synchronous manner with respect to writing messages into a stream. If true, the producer will wait for a response after a send operation.
Default: false
send-timeout
Effective only if sync
is set to true. The amount of time to wait for a response after a send operation, in milliseconds.
Default: 10000
Service Bus Queue Consumer Properties
It supports the following configurations with the format of spring.cloud.stream.servicebus.queue.bindings.<channelName>.consumer
.
checkpoint-mode
The mode in which checkpoints are updated.
RECORD
, checkpoints occur after each record successfully processed by user-defined message handler without any exception.
MANUAL
, checkpoints occur on demand by the user via the Checkpointer
. You can get Checkpointer
by `Message.getHeaders.get(AzureHeaders.CHECKPOINTER)`callback.
Default: RECORD
prefetch-count
Prefetch count of underlying service bus client.
Default: 1
maxConcurrentCalls
Controls the max concurrent calls of service bus message handler and the size of fixed thread pool that handles user’s business logic
Default: 1
maxConcurrentSessions
Controls the maximum number of concurrent sessions to process at any given time.
Default: 1
concurrency
When sessionsEnabled
is true, controls the maximum number of concurrent sessions to process at any given time.
When sessionsEnabled
is false, controls the max concurrent calls of service bus message handler and the size of fixed thread pool that handles user’s business logic.
Deprecated, replaced with maxConcurrentSessions
when sessionsEnabled
is true and maxConcurrentCalls
when sessionsEnabled
is false
Default: 1
sessionsEnabled
Controls if is a session aware consumer. Set it to true
if is a queue with sessions enabled.
Default: false
requeueRejected
Controls if is a message that trigger any exception in consumer will be force to DLQ.
Set it to true
if a message that trigger any exception in consumer will be force to DLQ.
Set it to false
if a message that trigger any exception in consumer will be re-queued.
Default: false
receiveMode
The modes for receiving messages.
PEEK_LOCK
, received message is not deleted from the queue or subscription, instead it is temporarily locked to the receiver, making it invisible to other receivers.
RECEIVE_AND_DELETE
, received message is removed from the queue or subscription and immediately deleted.
Default: PEEK_LOCK
enableAutoComplete
Enable auto-complete and auto-abandon of received messages. 'enableAutoComplete' is not needed in for RECEIVE_AND_DELETE mode.
Default: false
Support for Service Bus Message Headers and Properties
The following table illustrates how Spring message headers are mapped to Service Bus message headers and properties. When create a message, developers can specify the header or property of a Service Bus message by below constants.
For some Service Bus headers that can be mapped to multiple Spring header constants, the priority of different Spring headers is listed.
Service Bus Message Headers and Properties |
Spring Message Header Constants |
Type |
Priority Number (Descending priority) |
MessageId |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.MESSAGE_ID |
String |
1 |
MessageId |
com.azure.spring.integration.core.AzureHeaders.RAW_ID |
String |
2 |
MessageId |
org.springframework.messaging.MessageHeaders.ID |
UUID |
3 |
ContentType |
org.springframework.messaging.MessageHeaders.CONTENT_TYPE |
String |
N/A |
ReplyTo |
org.springframework.messaging.MessageHeaders.REPLY_CHANNEL |
String |
N/A |
ScheduledEnqueueTimeUtc |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.SCHEDULED_ENQUEUE_TIME |
OffsetDateTime |
1 |
ScheduledEnqueueTimeUtc |
com.azure.spring.integration.core.AzureHeaders.SCHEDULED_ENQUEUE_MESSAGE |
Integer |
2 |
TimeToLive |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.TIME_TO_LIVE |
Duration |
N/A |
SessionID |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.SESSION_ID |
String |
N/A |
CorrelationId |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.CORRELATION_ID |
String |
N/A |
To |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.TO |
String |
N/A |
ReplyToSessionId |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.REPLY_TO_SESSION_ID |
String |
N/A |
PartitionKey |
com.azure.spring.integration.servicebus.converter.ServiceBusMessageHeaders.PARTITION_KEY |
String |
1 |
PartitionKey |
com.azure.spring.integration.core.AzureHeaders.PARTITION_KEY |
String |
2 |
For full configurations, please check appendix
13.2.4. Samples
Example: Manually set the partition key for the message
This example demonstrates how to manually set the partition key for the message in the application.
Approach 1: Set partition key expression.
This example requires that spring.cloud.stream.default.producer.partitionKeyExpression
be set "'partitionKey-' + headers[<message-header-key>]"
.
spring:
cloud:
azure:
servicebus:
connection-string: [servicebus-namespace-connection-string]
stream:
default:
producer:
partitionKeyExpression: "'partitionKey-' + headers[<message-header-key>]"
@PostMapping("/messages")
public ResponseEntity<String> sendMessage(@RequestParam String message) {
LOGGER.info("Going to add message {} to Sinks.Many.", message);
many.emitNext(MessageBuilder.withPayload(message)
.setHeader("<message-header-key>", "Customize partirion key")
.build(), Sinks.EmitFailureHandler.FAIL_FAST);
return ResponseEntity.ok("Sent!");
}
When using application.yml to configure the partition key, its priority will be the lowest.
It will take effect only when the ServiceBusMessageHeaders.SESSION_ID , ServiceBusMessageHeaders.PARTITION_KEY , AzureHeaders.PARTITION_KEY are not configured.
|
Approach 2: Manually add the partition Key in the message header by code.
Recommended: Use ServiceBusMessageHeaders.PARTITION_KEY
as the key of the header.
@PostMapping("/messages")
public ResponseEntity<String> sendMessage(@RequestParam String message) {
LOGGER.info("Going to add message {} to Sinks.Many.", message);
many.emitNext(MessageBuilder.withPayload(message)
.setHeader(ServiceBusMessageHeaders.PARTITION_KEY, "Customize partirion key")
.build(), Sinks.EmitFailureHandler.FAIL_FAST);
return ResponseEntity.ok("Sent!");
}
Not recommended but currently supported: AzureHeaders.PARTITION_KEY
as the key of the header.
@PostMapping("/messages")
public ResponseEntity<String> sendMessage(@RequestParam String message) {
LOGGER.info("Going to add message {} to Sinks.Many.", message);
many.emitNext(MessageBuilder.withPayload(message)
.setHeader(AzureHeaders.PARTITION_KEY, "Customize partirion key")
.build(), Sinks.EmitFailureHandler.FAIL_FAST);
return ResponseEntity.ok("Sent!");
}
When both ServiceBusMessageHeaders.PARTITION_KEY and AzureHeaders.PARTITION_KEY are set in the message headers,
ServiceBusMessageHeaders.PARTITION_KEY is preferred.
|
Example: Set the session id for the message
This example demonstrates how to manually set the session id of a message in the application.
@PostMapping("/messages")
public ResponseEntity<String> sendMessage(@RequestParam String message) {
LOGGER.info("Going to add message {} to Sinks.Many.", message);
many.emitNext(MessageBuilder.withPayload(message)
.setHeader(ServiceBusMessageHeaders.SESSION_ID, "Customize session id")
.build(), Sinks.EmitFailureHandler.FAIL_FAST);
return ResponseEntity.ok("Sent!");
}
When the ServiceBusMessageHeaders.SESSION_ID is set in the message headers, and a different ServiceBusMessageHeaders.PARTITION_KEY (or AzureHeaders.PARTITION_KEY ) header is also set,
the value of the session id will eventually be used to overwrite the value of the partition key.
Please use this sample as a reference to learn more about how to use this binder in your project.
- Service Bus Queue
|
14. Kafka Support
Connect to Azure Event Hubs using Spring Kafka libraries. There’re two approaches to connect to Azure Event Hubs for Kafka, the first one is to provide the Azure Event Hubs connection string directly, the other is to use Azure Resource Manager to retrieve the connection string.
14.1. Dependency Setup
Adding below dependencies if you want to migrate your Apache Kafka application to use Azure Event Hubs for Kafka.
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter</artifactId>
</dependency>
If you want to retrieve the connection string using Azure Resource Manager, please also add below dependency
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-resourcemanager</artifactId>
</dependency>
14.2. Configuration
Below properties could be configured when using Kafka support:
Properties | Description |
---|---|
spring.cloud.azure.eventhubs.kafka.enabled |
Whether to enable the Azure Event Hubs Kafka support, defult to true. |
spring.cloud.azure.eventhubs.connection-string |
Azure Event Hubs connection string. Should be provided when want to provide the connection string directly. |
spring.cloud.azure.eventhubs.namespace |
Azure Event Hubs namespace. Should be provided when want to retrieve the connection information through Azure Resource Manager. |
spring.cloud.azure.eventhubs.resource.resource-group |
The resource group of Azure Event Hubs namespace. Should be provided when want to retrieve the connection information through Azure Resource Manager. |
spring.cloud.azure.profile.subscription-id |
The subscription id. Should be provided when want to retrieve the connection information through Azure Resource Manager. |
Authentication information is also required for authenticating for Azure Resource Manager. The credential related configurations of Resource Manager should be configured under prefix spring.cloud.azure . Please refer to Authentication for more details.
|
14.3. Basic Usage
14.3.1. Use Event Hubs connection string
The simplest way to connect to Event Hubs for Kafka is with the connection string.
Add below properties and you are good to go.
spring:
cloud:
azure:
eventhubs:
connection-string: ${AZURE_EVENTHUBS_CONNECTION_STRING}
14.3.2. Use Azure Resource Manager to retrieve connection string
If you don’t want to configure connection string in your application, it’s also possible to use Azure Resource Manager to retrieve the connection string. And you could use credentials stored in Azure CLI or other local development tool, like Visual Studio Code or Intellij IDEA to authenticate with Azure Resource Manager. Or Managed Identity if your application is deployed to Azure Cloud. Just make sure the principal have sufficient permission to read resource metadata.
Add below properties and you are good to go.
spring:
cloud:
azure:
profile:
subscription-id: ${AZURE_SUBSCRIPTION_ID}
eventhubs:
namespace: ${AZURE_EVENTHUBS_NAMESPACE}
resource:
resource-group: ${AZURE_EVENTHUBS_RESOURCE_GROUP}
14.4. Samples
Please refer to samples for Azure Event Hus for Kafka for more details.
15. Redis Support
Connect to Azure Cache for Redis using Spring Redis libraries. With adding spring-cloud-azure-starter
and spring-cloud-azure-resourcemanager
to your application, it’s possible to read the Azure Cache for Redis connection information through Azure Resource Manager and auto-configure the Redis properties.
15.1. Dependency Setup
Adding below dependencies if you want to use the Spring Cloud Azure Redis support to your Spring Boot application using Redis.
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter</artifactId>
</dependency>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-resourcemanager</artifactId>
</dependency>
15.2. Configuration
Below properties could be configured when using Redis support:
Properties | Description | Default Value | Required |
---|---|---|---|
spring.cloud.azure.redis.enabled |
Azure Cache for Redis instance name. |
true |
No |
spring.cloud.azure.redis.name |
Azure Cache for Redis instance name. |
Yes |
|
spring.cloud.azure.redis.resource.resource-group |
The resource group of Azure Cache for Redis. |
Yes |
|
spring.cloud.azure.profile.subscription-id |
The subscription id. |
Yes |
Authentication information is also required for authenticating for Azure Resource Manager. The credential related configurations of Resource Manager should be configured under prefix spring.cloud.azure . Please refer to Authentication for more details.
|
15.3. Basic Usage
Add below properties and you are good to go.
spring:
cloud:
azure:
redis:
name: ${AZURE_CACHE_REDIS_NAME}
resource:
resource-group: ${AZURE_CACHE_REDIS_RESOURCE_GROUP}
15.4. Samples
Please refer to samples for Azure Cache for Redis for more details.
16. Resource Manager
Connect to Azure Resources for All Azure SDKs service, which Spring Cloud used.
Construct TokenCredential
by using various credential information, and then construct AzureResourceManager
to help Azure SDKs Client to authenticate and authorize.
16.1. Dependency Setup
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-resourcemanager</artifactId>
</dependency>
16.2. Configuration
This Spring Cloud Azure Resource Manager provides the following properties:
Properties | Description |
---|---|
spring.cloud.azure.resource-manager.enabled |
Whether the Resource Manager is enabled. Default is true. |
spring.cloud.azure.credential.client-certificate-password |
Password of the certificate file. |
spring.cloud.azure.credential.client-certificate-path |
Path of a PEM certificate file to use when performing service principal authentication with Azure. |
spring.cloud.azure.credential.client-id |
Client id to use when performing service principal authentication with Azure. |
spring.cloud.azure.credential.client-secret |
Client secret to use when performing service principal authentication with Azure. |
spring.cloud.azure.credential.managed-identity-client-id |
Client id to use when using managed identity to authenticate with Azure. |
spring.cloud.azure.credential.username |
Username to use when performing username/password authentication with Azure. |
spring.cloud.azure.credential.password |
Password to use when performing username/password authentication. |
spring.cloud.azure.profile.cloud |
Name of the Azure cloud to connect to. |
spring.cloud.azure.profile.environment.active-directory-endpoint |
The Azure Active Directory endpoint to connect to for authentication. |
spring.cloud.azure.profile.subscription-id |
Subscription id to use when connecting to Azure resources. |
spring.cloud.azure.profile.tenant-id |
Tenant id for Azure resources. |
16.3. Basic Usage
Azure Resource Manger helps the Azure SDK client to complete authentication and authorization. It can be integrated into a specific Spring Cloud Azure Starter and work together, or it can be used with Spring Cloud Azure auto-configuration modules and third-party libraries to complete authentication, such as: Kafka Support, Redis Support.
16.4. Samples
Please see Kafka Support, Redis Support for more information about usage.
17. Configuration Properties
To see the list of all Spring Cloud Azure related configuration properties please check the Appendix page.