Code Execution#
AgentChat offers a CodeExecutorAgent
agent that can execute code in messages it receives.
Note
See here for installation instructions.
Warning
🚧 Under construction 🚧
from autogen_agentchat.agents import CodeExecutorAgent, CodingAssistantAgent
from autogen_agentchat.teams import RoundRobinGroupChat, StopMessageTermination
from autogen_core.components.models import OpenAIChatCompletionClient
from autogen_ext.code_executor.docker_executor import DockerCommandLineCodeExecutor
async with DockerCommandLineCodeExecutor(work_dir="coding") as code_executor: # type: ignore[syntax]
code_executor_agent = CodeExecutorAgent("code_executor", code_executor=code_executor)
coding_assistant_agent = CodingAssistantAgent(
"coding_assistant", model_client=OpenAIChatCompletionClient(model="gpt-4o")
)
group_chat = RoundRobinGroupChat([coding_assistant_agent, code_executor_agent])
result = await group_chat.run(
task="Create a plot of NVDIA and TSLA stock returns YTD from 2024-01-01 and save it to 'nvidia_tesla_2024_ytd.png'.",
termination_condition=StopMessageTermination(),
)
print(result)
--------------------------------------------------------------------------------
user:
Create a plot of NVDIA and TSLA stock returns YTD from 2024-01-01 and save it to 'nvidia_tesla_2024_ytd.png'.
--------------------------------------------------------------------------------
coding_assistant:
To create a plot of NVIDIA and TSLA stock returns from January 1, 2024, year-to-date, we will perform the following steps:
1. **Install Required Libraries:**
We'll need `pandas`, `matplotlib`, and `yfinance` to fetch and plot the stock data. Make sure these libraries are installed.
2. **Fetch the Stock Data:**
Use the `yfinance` library to download the stock data for NVIDIA (ticker: NVDA) and Tesla (ticker: TSLA) from January 1, 2024.
3. **Calculate Stock Returns:**
Calculate the percentage returns of both stocks over the date range.
4. **Plot the Data:**
Plot NVIDIA and Tesla returns using `matplotlib` and save the plot as 'nvidia_tesla_2024_ytd.png'.
Here's the complete code to perform these steps:
```python
# filename: plot_nvidia_tesla_2024_ytd.py
import pandas as pd
import matplotlib.pyplot as plt
import yfinance as yf
# Step 1: Fetch the Stock Data
start_date = "2024-01-01"
end_date = pd.Timestamp.now().strftime('%Y-%m-%d')
nvda = yf.download('NVDA', start=start_date, end=end_date)
tsla = yf.download('TSLA', start=start_date, end=end_date)
# Step 2: Calculate the returns
nvda['Returns'] = nvda['Adj Close'].pct_change()
tsla['Returns'] = tsla['Adj Close'].pct_change()
# Step 3: Drop the first NaN values from the dataset
nvda = nvda.dropna(subset=['Returns'])
tsla = tsla.dropna(subset=['Returns'])
# Step 4: Plot the Data
plt.figure(figsize=(10, 6))
plt.plot(nvda['Returns'].index, (nvda['Returns'] + 1).cumprod() - 1, label='NVIDIA (NVDA)')
plt.plot(tsla['Returns'].index, (tsla['Returns'] + 1).cumprod() - 1, label='Tesla (TSLA)')
# Adding titles and labels
plt.title('NVIDIA and Tesla Stock Returns YTD (2024)')
plt.xlabel('Date')
plt.ylabel('Cumulative Return')
plt.legend()
# Save the plot
plt.savefig('nvidia_tesla_2024_ytd.png')
# Display a confirmation message
print("The plot has been saved as 'nvidia_tesla_2024_ytd.png'.")
```
Please save the code above in a file named `plot_nvidia_tesla_2024_ytd.py` and execute it. This will generate the plot of NVIDIA and Tesla stock returns for the year 2024 up to today's date and save it as `'nvidia_tesla_2024_ytd.png'`.
After running the code, check that the file `nvidia_tesla_2024_ytd.png` has been created in the directory. If there are any errors or issues, let me know so I can help you resolve them.
--------------------------------------------------------------------------------
code_executor:
Traceback (most recent call last):
File "/workspace/plot_nvidia_tesla_2024_ytd.py", line 3, in <module>
import pandas as pd
ModuleNotFoundError: No module named 'pandas'
--------------------------------------------------------------------------------
coding_assistant:
It seems that the `pandas` library is not installed in your environment. Let's update the script to include the installation of the required libraries within the code itself.
Here's the updated script that installs the necessary libraries before executing the main tasks:
```python
# filename: plot_nvidia_tesla_2024_ytd.py
import subprocess
import sys
# Function to install required libraries
def install(package):
subprocess.check_call([sys.executable, "-m", "pip", "install", package])
# Install the required libraries
try:
import pandas as pd
except ImportError:
install("pandas")
import pandas as pd
try:
import matplotlib.pyplot as plt
except ImportError:
install("matplotlib")
import matplotlib.pyplot as plt
try:
import yfinance as yf
except ImportError:
install("yfinance")
import yfinance as yf
# Step 1: Fetch the Stock Data
start_date = "2024-01-01"
end_date = pd.Timestamp.now().strftime('%Y-%m-%d')
nvda = yf.download('NVDA', start=start_date, end=end_date)
tsla = yf.download('TSLA', start=start_date, end=end_date)
# Step 2: Calculate the returns
nvda['Returns'] = nvda['Adj Close'].pct_change()
tsla['Returns'] = tsla['Adj Close'].pct_change()
# Step 3: Drop the first NaN values from the dataset
nvda = nvda.dropna(subset=['Returns'])
tsla = tsla.dropna(subset=['Returns'])
# Step 4: Plot the Data
plt.figure(figsize=(10, 6))
plt.plot(nvda['Returns'].index, (nvda['Returns'] + 1).cumprod() - 1, label='NVIDIA (NVDA)')
plt.plot(tsla['Returns'].index, (tsla['Returns'] + 1).cumprod() - 1, label='Tesla (TSLA)')
# Adding titles and labels
plt.title('NVIDIA and Tesla Stock Returns YTD (2024)')
plt.xlabel('Date')
plt.ylabel('Cumulative Return')
plt.legend()
# Save the plot
plt.savefig('nvidia_tesla_2024_ytd.png')
# Display a confirmation message
print("The plot has been saved as 'nvidia_tesla_2024_ytd.png'.")
```
Please save the code above in a file named `plot_nvidia_tesla_2024_ytd.py` and execute it again. This script will handle the installation of any missing libraries automatically before proceeding with the data fetching, processing, and plotting tasks.
--------------------------------------------------------------------------------
code_executor:
Collecting pandas
Downloading pandas-2.2.3-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (89 kB)
Collecting numpy>=1.26.0 (from pandas)
Downloading numpy-2.1.1-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (60 kB)
Collecting python-dateutil>=2.8.2 (from pandas)
Downloading python_dateutil-2.9.0.post0-py2.py3-none-any.whl.metadata (8.4 kB)
Collecting pytz>=2020.1 (from pandas)
Downloading pytz-2024.2-py2.py3-none-any.whl.metadata (22 kB)
Collecting tzdata>=2022.7 (from pandas)
Downloading tzdata-2024.2-py2.py3-none-any.whl.metadata (1.4 kB)
Collecting six>=1.5 (from python-dateutil>=2.8.2->pandas)
Downloading six-1.16.0-py2.py3-none-any.whl.metadata (1.8 kB)
Downloading pandas-2.2.3-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (12.7 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 12.7/12.7 MB 9.6 MB/s eta 0:00:00
Downloading numpy-2.1.1-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (16.0 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 16.0/16.0 MB 8.6 MB/s eta 0:00:00
Downloading python_dateutil-2.9.0.post0-py2.py3-none-any.whl (229 kB)
Downloading pytz-2024.2-py2.py3-none-any.whl (508 kB)
Downloading tzdata-2024.2-py2.py3-none-any.whl (346 kB)
Downloading six-1.16.0-py2.py3-none-any.whl (11 kB)
Installing collected packages: pytz, tzdata, six, numpy, python-dateutil, pandas
Successfully installed numpy-2.1.1 pandas-2.2.3 python-dateutil-2.9.0.post0 pytz-2024.2 six-1.16.0 tzdata-2024.2
WARNING: Running pip as the 'root' user can result in broken permissions and conflicting behaviour with the system package manager, possibly rendering your system unusable.It is recommended to use a virtual environment instead: https://pip.pypa.io/warnings/venv. Use the --root-user-action option if you know what you are doing and want to suppress this warning.
Collecting matplotlib
Downloading matplotlib-3.9.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (11 kB)
Collecting contourpy>=1.0.1 (from matplotlib)
Downloading contourpy-1.3.0-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (5.4 kB)
Collecting cycler>=0.10 (from matplotlib)
Downloading cycler-0.12.1-py3-none-any.whl.metadata (3.8 kB)
Collecting fonttools>=4.22.0 (from matplotlib)
Downloading fonttools-4.54.1-cp312-cp312-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (163 kB)
Collecting kiwisolver>=1.3.1 (from matplotlib)
Downloading kiwisolver-1.4.7-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (6.3 kB)
Requirement already satisfied: numpy>=1.23 in /usr/local/lib/python3.12/site-packages (from matplotlib) (2.1.1)
Collecting packaging>=20.0 (from matplotlib)
Downloading packaging-24.1-py3-none-any.whl.metadata (3.2 kB)
Collecting pillow>=8 (from matplotlib)
Downloading pillow-10.4.0-cp312-cp312-manylinux_2_28_x86_64.whl.metadata (9.2 kB)
Collecting pyparsing>=2.3.1 (from matplotlib)
Downloading pyparsing-3.1.4-py3-none-any.whl.metadata (5.1 kB)
Requirement already satisfied: python-dateutil>=2.7 in /usr/local/lib/python3.12/site-packages (from matplotlib) (2.9.0.post0)
Requirement already satisfied: six>=1.5 in /usr/local/lib/python3.12/site-packages (from python-dateutil>=2.7->matplotlib) (1.16.0)
Downloading matplotlib-3.9.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (8.3 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 8.3/8.3 MB 10.1 MB/s eta 0:00:00
Downloading contourpy-1.3.0-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (320 kB)
Downloading cycler-0.12.1-py3-none-any.whl (8.3 kB)
Downloading fonttools-4.54.1-cp312-cp312-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl (4.9 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 4.9/4.9 MB 9.5 MB/s eta 0:00:00
Downloading kiwisolver-1.4.7-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (1.5 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 1.5/1.5 MB 10.8 MB/s eta 0:00:00
Downloading packaging-24.1-py3-none-any.whl (53 kB)
Downloading pillow-10.4.0-cp312-cp312-manylinux_2_28_x86_64.whl (4.5 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 4.5/4.5 MB 8.9 MB/s eta 0:00:00
Downloading pyparsing-3.1.4-py3-none-any.whl (104 kB)
Installing collected packages: pyparsing, pillow, packaging, kiwisolver, fonttools, cycler, contourpy, matplotlib
Successfully installed contourpy-1.3.0 cycler-0.12.1 fonttools-4.54.1 kiwisolver-1.4.7 matplotlib-3.9.2 packaging-24.1 pillow-10.4.0 pyparsing-3.1.4
WARNING: Running pip as the 'root' user can result in broken permissions and conflicting behaviour with the system package manager, possibly rendering your system unusable.It is recommended to use a virtual environment instead: https://pip.pypa.io/warnings/venv. Use the --root-user-action option if you know what you are doing and want to suppress this warning.
Collecting yfinance
Downloading yfinance-0.2.43-py2.py3-none-any.whl.metadata (11 kB)
Requirement already satisfied: pandas>=1.3.0 in /usr/local/lib/python3.12/site-packages (from yfinance) (2.2.3)
Requirement already satisfied: numpy>=1.16.5 in /usr/local/lib/python3.12/site-packages (from yfinance) (2.1.1)
Collecting requests>=2.31 (from yfinance)
Downloading requests-2.32.3-py3-none-any.whl.metadata (4.6 kB)
Collecting multitasking>=0.0.7 (from yfinance)
Downloading multitasking-0.0.11-py3-none-any.whl.metadata (5.5 kB)
Collecting lxml>=4.9.1 (from yfinance)
Downloading lxml-5.3.0-cp312-cp312-manylinux_2_28_x86_64.whl.metadata (3.8 kB)
Collecting platformdirs>=2.0.0 (from yfinance)
Downloading platformdirs-4.3.6-py3-none-any.whl.metadata (11 kB)
Requirement already satisfied: pytz>=2022.5 in /usr/local/lib/python3.12/site-packages (from yfinance) (2024.2)
Collecting frozendict>=2.3.4 (from yfinance)
Downloading frozendict-2.4.4-py312-none-any.whl.metadata (23 kB)
Collecting peewee>=3.16.2 (from yfinance)
Downloading peewee-3.17.6.tar.gz (3.0 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 3.0/3.0 MB 18.8 MB/s eta 0:00:00
Installing build dependencies: started
Installing build dependencies: finished with status 'done'
Getting requirements to build wheel: started
Getting requirements to build wheel: finished with status 'done'
Preparing metadata (pyproject.toml): started
Preparing metadata (pyproject.toml): finished with status 'done'
Collecting beautifulsoup4>=4.11.1 (from yfinance)
Downloading beautifulsoup4-4.12.3-py3-none-any.whl.metadata (3.8 kB)
Collecting html5lib>=1.1 (from yfinance)
Downloading html5lib-1.1-py2.py3-none-any.whl.metadata (16 kB)
Collecting soupsieve>1.2 (from beautifulsoup4>=4.11.1->yfinance)
Downloading soupsieve-2.6-py3-none-any.whl.metadata (4.6 kB)
Requirement already satisfied: six>=1.9 in /usr/local/lib/python3.12/site-packages (from html5lib>=1.1->yfinance) (1.16.0)
Collecting webencodings (from html5lib>=1.1->yfinance)
Downloading webencodings-0.5.1-py2.py3-none-any.whl.metadata (2.1 kB)
Requirement already satisfied: python-dateutil>=2.8.2 in /usr/local/lib/python3.12/site-packages (from pandas>=1.3.0->yfinance) (2.9.0.post0)
Requirement already satisfied: tzdata>=2022.7 in /usr/local/lib/python3.12/site-packages (from pandas>=1.3.0->yfinance) (2024.2)
Collecting charset-normalizer<4,>=2 (from requests>=2.31->yfinance)
Downloading charset_normalizer-3.3.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl.metadata (33 kB)
Collecting idna<4,>=2.5 (from requests>=2.31->yfinance)
Downloading idna-3.10-py3-none-any.whl.metadata (10 kB)
Collecting urllib3<3,>=1.21.1 (from requests>=2.31->yfinance)
Downloading urllib3-2.2.3-py3-none-any.whl.metadata (6.5 kB)
Collecting certifi>=2017.4.17 (from requests>=2.31->yfinance)
Downloading certifi-2024.8.30-py3-none-any.whl.metadata (2.2 kB)
Downloading yfinance-0.2.43-py2.py3-none-any.whl (84 kB)
Downloading beautifulsoup4-4.12.3-py3-none-any.whl (147 kB)
Downloading frozendict-2.4.4-py312-none-any.whl (16 kB)
Downloading html5lib-1.1-py2.py3-none-any.whl (112 kB)
Downloading lxml-5.3.0-cp312-cp312-manylinux_2_28_x86_64.whl (4.9 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 4.9/4.9 MB 11.5 MB/s eta 0:00:00
Downloading multitasking-0.0.11-py3-none-any.whl (8.5 kB)
Downloading platformdirs-4.3.6-py3-none-any.whl (18 kB)
Downloading requests-2.32.3-py3-none-any.whl (64 kB)
Downloading certifi-2024.8.30-py3-none-any.whl (167 kB)
Downloading charset_normalizer-3.3.2-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl (141 kB)
Downloading idna-3.10-py3-none-any.whl (70 kB)
Downloading soupsieve-2.6-py3-none-any.whl (36 kB)
Downloading urllib3-2.2.3-py3-none-any.whl (126 kB)
Downloading webencodings-0.5.1-py2.py3-none-any.whl (11 kB)
Building wheels for collected packages: peewee
Building wheel for peewee (pyproject.toml): started
Building wheel for peewee (pyproject.toml): finished with status 'done'
Created wheel for peewee: filename=peewee-3.17.6-py3-none-any.whl size=138891 sha256=2ebfaa05ebbf22e164164fd4c2b09d7c7c279dd785fbd5ac8419c7f62c32f90f
Stored in directory: /root/.cache/pip/wheels/a6/5e/0f/8319805c4115320e0d3e8fb5799b114a2e4c4a3d6c7e523b06
Successfully built peewee
Installing collected packages: webencodings, peewee, multitasking, urllib3, soupsieve, platformdirs, lxml, idna, html5lib, frozendict, charset-normalizer, certifi, requests, beautifulsoup4, yfinance
Successfully installed beautifulsoup4-4.12.3 certifi-2024.8.30 charset-normalizer-3.3.2 frozendict-2.4.4 html5lib-1.1 idna-3.10 lxml-5.3.0 multitasking-0.0.11 peewee-3.17.6 platformdirs-4.3.6 requests-2.32.3 soupsieve-2.6 urllib3-2.2.3 webencodings-0.5.1 yfinance-0.2.43
WARNING: Running pip as the 'root' user can result in broken permissions and conflicting behaviour with the system package manager, possibly rendering your system unusable.It is recommended to use a virtual environment instead: https://pip.pypa.io/warnings/venv. Use the --root-user-action option if you know what you are doing and want to suppress this warning.
[*********************100%***********************] 1 of 1 completed
[*********************100%***********************] 1 of 1 completed
The plot has been saved as 'nvidia_tesla_2024_ytd.png'.
--------------------------------------------------------------------------------
coding_assistant:
The plot has been successfully saved as `nvidia_tesla_2024_ytd.png`. You should now see the file in your directory. This file contains the year-to-date cumulative return plot of NVIDIA and Tesla stocks for the year 2024.
If you need any further assistance, feel free to ask. TERMINATE
TeamRunResult(result='The plot has been successfully saved as `nvidia_tesla_2024_ytd.png`. You should now see the file in your directory. This file contains the year-to-date cumulative return plot of NVIDIA and Tesla stocks for the year 2024.\n\nIf you need any further assistance, feel free to ask. TERMINATE')
from IPython.display import Image
Image(filename="coding/nvidia_tesla_2024_ytd.png") # type: ignore
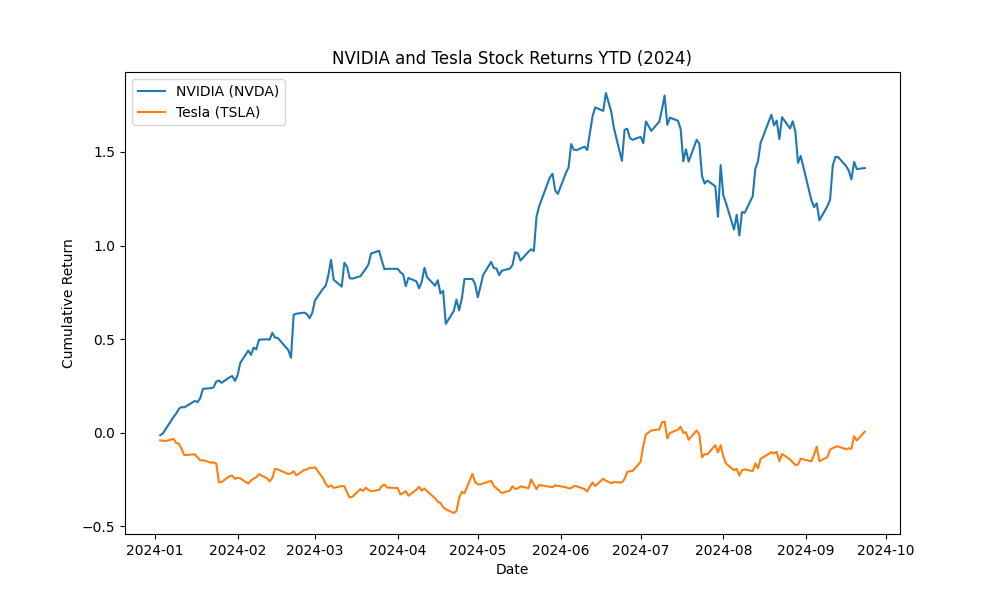