Image Alt Text
It is a best practice to provide an alt
attribute for images.
This attribute is used to describe the image to users who are unable to see it.
It is also used by search engines to understand the content of the image.
<img src="..." alt="describe the image here" />
However, this task can be tedious and developers are often tempted to skip it, or provide a generic alt
text like “image”.
<img src="..." alt="image" />
The script
To solve this issue, we created a script that uses the OpenAI Vision model to analyze the documentation images and generate a description alt text.
To start, we assume that the script is run on a single image file and we use defImage to add it to the prompt context.
const file = env.files[0]defImages(file)
Then we give a task to the LLM to generate a good alt text.
...$`You are an expert in assistive technology. You will analyze each imageand generate a description alt text for the image.`
finally, we use defFileOutput to define a file output route.
...defFileOutput(file.filename + ".txt", `Alt text for image ${file.filename}`)
Usage in Astro
The GenAIScript documentation uses Astro, which allows to author pages in MDX. The code below shows how the generated alt text, stored in a separate text file, is injected in the final HTML.
import { Image } from "astro:assets"import src from "../../../assets/debugger.png"import alt from "../../../assets/debugger.png.txt?raw"
<Image src={src} alt={alt} />
The debugger.png
image shows the screenshot of a debugging session and the generated alt text file contents.
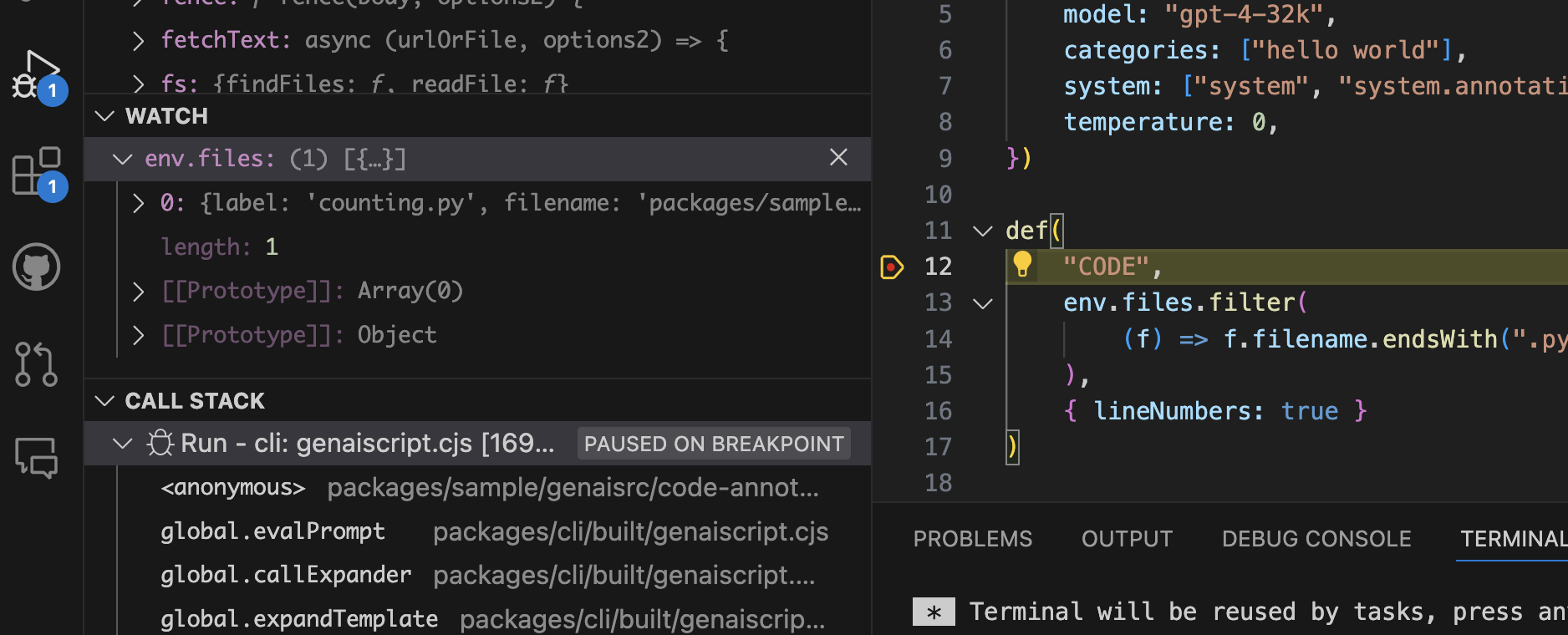
A screenshot of a debugging session in a code editor with a breakpoint set on a line of code. The editor is displaying several panels including the watch variables, call stack, and a terminal output. The code is partially visible with a function definition and JSON configuration data.
Automation
Using the run command, we can apply the script to each image in the docs.
for file in assets/**.png; do npx --yes genaiscript run image-alt-text "$file"
To avoid regenerating the alt text, we also detect if a file exists in the script and cancel accordingly.
for file in assets/**.png; do if [ ! -f "$file" ]; then npx --yes genaiscript run image-alt-text "$file" fidone
Full source
The full source looks like this:
script({ title: "Image Alt Text generator", description: "Generate alt text for images", model: "large", group: "docs", maxTokens: 4000, temperature: 0,})
// inputconst file = env.files[0]// contextdefImages(file)// task$`You are an expert in assistive technology. You will analyze each imageand generate a description alt text for the image and save it to a file.- Do not include Alt text in the description.`// outputdefFileOutput(file.filename + ".txt", `Alt text for image ${file.filename}`)