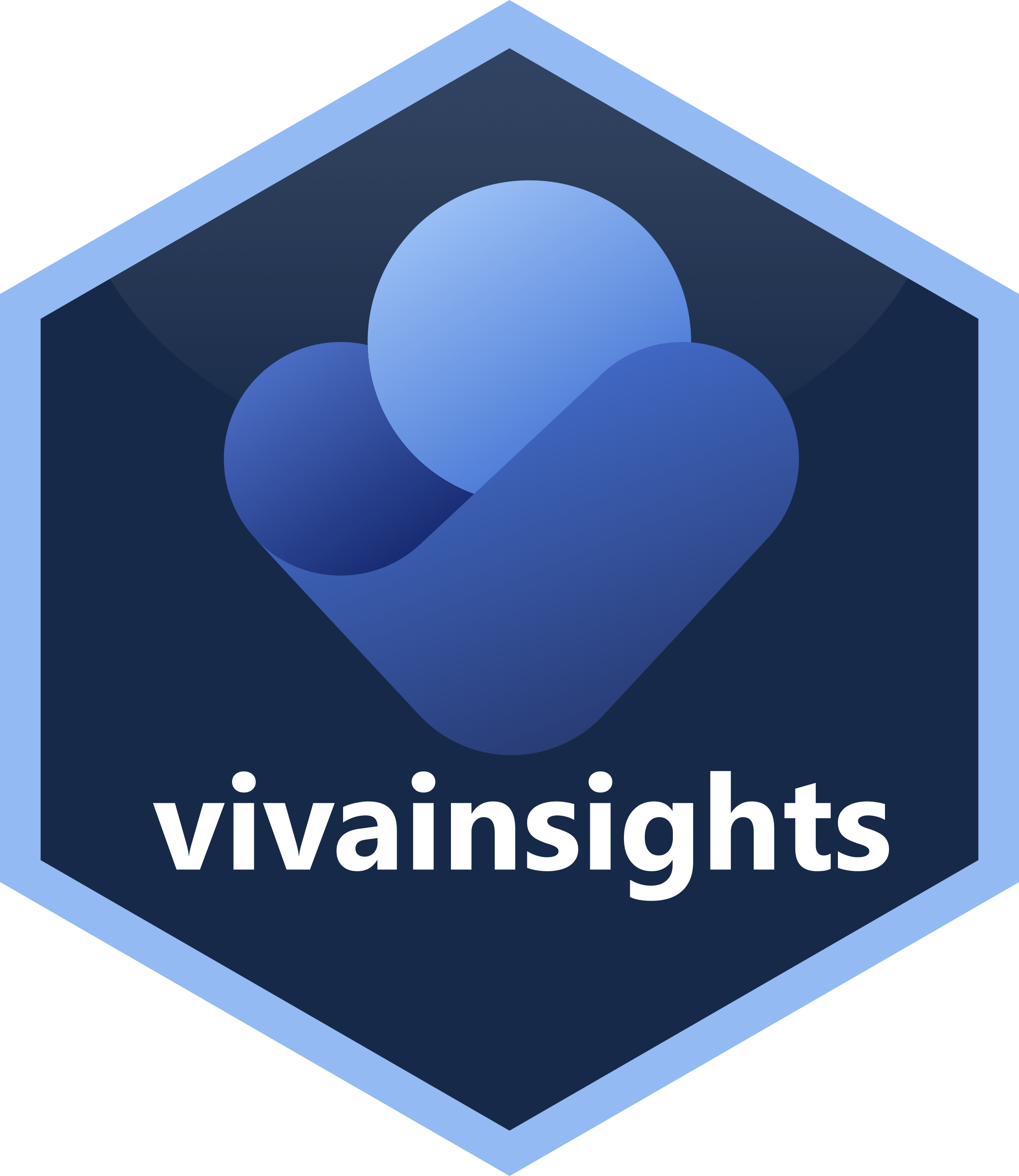
Tenure calculation based on different input dates, returns data summary table or histogram
Source:R/identify_tenure.R
identify_tenure.Rd
This function calculates employee tenure based on different input dates.
identify_tenure
uses the latest Date available if user selects "MetricDate",
but also have flexibility to select a specific date, e.g. "1/1/2020".
Usage
identify_tenure(
data,
end_date = "MetricDate",
beg_date = "HireDate",
maxten = 40,
return = "message"
)
Arguments
- data
A Standard Person Query dataset in the form of a data frame.
- end_date
A string specifying the name of the date variable representing the latest date. Defaults to "MetricDate".
- beg_date
A string specifying the name of the date variable representing the hire date. Defaults to "HireDate".
- maxten
A numeric value representing the maximum tenure. If the tenure exceeds this threshold, it would be accounted for in the flag message.
- return
String specifying what to return. This must be one of the following strings:
"message"
"text"
"plot"
"data_cleaned"
"data_dirty"
"data"
See
Value
for more information.
Value
A different output is returned depending on the value passed to the return
argument:
"message"
: message on console with a diagnostic message."text"
: string containing a diagnostic message."plot"
: 'ggplot' object. A line plot showing tenure."data_cleaned"
: data frame filtered only by rows with tenure values lying within the threshold."data_dirty"
: data frame filtered only by rows with tenure values lying outside the threshold."data"
: data frame with thePersonId
and a calculated variable calledTenureYear
is returned.
See also
Other Data Validation:
check_query()
,
extract_hr()
,
flag_ch_ratio()
,
flag_em_ratio()
,
flag_extreme()
,
flag_outlooktime()
,
hr_trend()
,
hrvar_count()
,
hrvar_count_all()
,
hrvar_trend()
,
identify_churn()
,
identify_holidayweeks()
,
identify_inactiveweeks()
,
identify_nkw()
,
identify_outlier()
,
identify_privacythreshold()
,
identify_shifts()
,
track_HR_change()
,
validation_report()