This page was generated from
docs/examples/driver_examples/Qcodes example with Alazar ATS9360.ipynb.
Interactive online version:
.
QCoDeS Example with Alazar ATS 9360
In this notebook, we want to show how to setup an Alazar ATS9360 card and its acquisition controller in qcodes to acquire data. In this example, we only demonstrate how to setup the card for an acquisition job without physically contected to any other instrument like wave form generators. Although, this notebook is aimed for ATS9360, most of its content can be useful for other models of Alazar cards having their qcodes driver.
Required imports
[1]:
from qcodes.dataset import do1d, plot_by_id
from qcodes.instrument_drivers.AlazarTech import (
AlazarTechATS9360,
DemodulationAcquisitionController,
)
from qcodes.parameters import ManualParameter
Setting up Alazar and performing an acquisition
First, lets list all the Alazar boards connected to this machine. In most cases this will probably only be a single one:
[2]:
AlazarTechATS9360.find_boards()
[2]:
[{'system_id': 1,
'board_id': 1,
'board_kind': 'ATS9360',
'max_samples': 4294967294,
'bits_per_sample': 12}]
Now, we create an instance of alazar:
[3]:
ats_inst = AlazarTechATS9360(name='Alazar1')
This prints all information about this Alazar card:
[4]:
ats_inst.get_idn() # or .IDN()
[4]:
{'firmware': '21.09',
'model': 'ATS9360',
'max_samples': 4294967294,
'bits_per_sample': 12,
'serial': '970396',
'vendor': 'AlazarTech',
'CPLD_version': '25.16',
'driver_version': '6.5.1',
'SDK_version': '6.5.1',
'latest_cal_date': '25-01-17',
'memory_size': '4294967294',
'asopc_type': 1763017568,
'pcie_link_speed': '0.5GB/s',
'pcie_link_width': '8'}
The parameters on the Alazar card are set in a slightly unusual way. As communicating with the card is slow, and sometimes multiple parameters have to be set with a single “command”, we use a context manager that takes care of syncing all the paramters to the card after we set them. Remember to sync parameter values to Alazar card when changing values of those parameters which correspond to Alazar card settings (for example sample_rate parameter is a global “setting” for the card, while for example records_per_buffer is a parameter of the acquisition process and is set to the card only when performing an acquisition):
[5]:
with ats_inst.syncing():
ats_inst.clock_source('INTERNAL_CLOCK')
ats_inst.sample_rate(1_000_000_000)
ats_inst.clock_edge('CLOCK_EDGE_RISING')
ats_inst.decimation(1)
ats_inst.coupling1('DC')
ats_inst.coupling2('DC')
ats_inst.channel_range1(.4)
ats_inst.channel_range2(.4)
ats_inst.impedance1(50)
ats_inst.impedance2(50)
ats_inst.trigger_operation('TRIG_ENGINE_OP_J')
ats_inst.trigger_engine1('TRIG_ENGINE_J')
ats_inst.trigger_source1('EXTERNAL')
ats_inst.trigger_slope1('TRIG_SLOPE_POSITIVE')
ats_inst.trigger_level1(160)
ats_inst.trigger_engine2('TRIG_ENGINE_K')
ats_inst.trigger_source2('DISABLE')
ats_inst.trigger_slope2('TRIG_SLOPE_POSITIVE')
ats_inst.trigger_level2(128)
ats_inst.external_trigger_coupling('DC')
ats_inst.external_trigger_range('ETR_2V5')
ats_inst.trigger_delay(0)
ats_inst.aux_io_mode('AUX_IN_AUXILIARY') # AUX_IN_TRIGGER_ENABLE for seq mode on
ats_inst.aux_io_param('NONE') # TRIG_SLOPE_POSITIVE for seq mode on
# Note that we set this parameter to a non-0 value for demonstration purposes - doing so allows
# to perform an acquisition using just the Alazar card without any additional hardware.
# Read Alazar SDK manual about `AlazarSetTriggerTimeOut` function for more information.
ats_inst.timeout_ticks(0)
The alazar qcodes driver is only for setting and/ or getting parameters defined in the manual of the card. Other acquisition functionalities at the moment are available in the module called ATS_acquisition_controllers. So, we instantiate this module with the already instantiated Alazar driver:
[6]:
acquisition_controller = DemodulationAcquisitionController(name='acquisition_controller',
demodulation_frequency=10e6,
alazar_name='Alazar1')
This following command is specific to this acquisition controller. The kwargs provided here are being forwarded to instrument’s acquire function (ats_inst.acquire()).
This way, it becomes (arguably) easy to change acquisition specific settings from the ipython notebook.
[7]:
acquisition_controller.update_acquisitionkwargs(#mode='NPT',
samples_per_record=1024,
records_per_buffer=74,
buffers_per_acquisition=1,
#channel_selection='AB',
#transfer_offset=0,
#external_startcapture='ENABLED',
#enable_record_headers='DISABLED',
#alloc_buffers='DISABLED',
#fifo_only_streaming='DISABLED',
#interleave_samples='DISABLED',
#get_processed_data='DISABLED',
allocated_buffers=1,
#buffer_timeout=1000
)
Getting the value of the parameter acquisition of the instrument acquisition_controller performes the entire acquisition protocol. The type/shape/kind of the returned value depends on the specific implementation of the acquisition controller in use. In this particular example, the output will be a single value (see the docstring of Demodulation_AcquisitionController).
[8]:
acquisition_controller.acquisition()
[8]:
4.430443717981646
Let’s have look at the snapshot of the Alazar instance:
[9]:
ats_inst.snapshot()
[9]:
{'functions': {},
'submodules': {},
'__class__': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'parameters': {'IDN': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_IDN',
'value': None,
'raw_value': None,
'ts': None,
'post_delay': 0,
'name': 'IDN',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'IDN',
'inter_delay': 0,
'vals': '<Anything>'},
'clock_source': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_clock_source',
'value': 'INTERNAL_CLOCK',
'raw_value': 1,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'val_mapping': {'INTERNAL_CLOCK': 1,
'FAST_EXTERNAL_CLOCK': 2,
'EXTERNAL_CLOCK_10MHz_REF': 7},
'name': 'clock_source',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Clock Source',
'inter_delay': 0,
'vals': "<Enum: {'EXTERNAL_CLOCK_10MHz_REF', 'FAST_EXTERNAL_CLOCK', 'INTERNAL_CLOCK'}>"},
'external_sample_rate': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_external_sample_rate',
'value': 'UNDEFINED',
'raw_value': 'UNDEFINED',
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'name': 'external_sample_rate',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'S/s',
'label': 'External Sample Rate',
'inter_delay': 0,
'vals': "<MultiType: Ints 300000000<=v<=1800000000, Enum: {'UNDEFINED'}>"},
'sample_rate': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_sample_rate',
'value': 1000000000,
'raw_value': 53,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'val_mapping': {1000: 1,
2000: 2,
5000: 4,
10000: 8,
20000: 10,
50000: 12,
100000: 14,
200000: 16,
500000: 18,
1000000: 20,
2000000: 24,
5000000: 26,
10000000: 28,
20000000: 30,
50000000: 34,
100000000: 36,
200000000: 40,
500000000: 48,
800000000: 50,
1000000000: 53,
1200000000: 55,
1500000000: 58,
1800000000: 61,
'EXTERNAL_CLOCK': 64,
'UNDEFINED': 'UNDEFINED'},
'name': 'sample_rate',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'S/s',
'label': 'Internal Sample Rate',
'inter_delay': 0,
'vals': "<Enum: {2000000, 10000000, 20000000, 50000000, 100000000, 500000000, 800000000, 200000000, 5000, 1000000000, 10000, 1800000000, 20000, 100000, 500000, 'UNDEFINED', 200000, 1000000, 5000000, 2000, 50000, 1200000000, 1000, 'EXTERNAL_CLOCK', 1500000000}>"},
'clock_edge': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_clock_edge',
'value': 'CLOCK_EDGE_RISING',
'raw_value': 0,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'CLOCK_EDGE_RISING': 0, 'CLOCK_EDGE_FALLING': 1},
'name': 'clock_edge',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Clock Edge',
'inter_delay': 0,
'vals': "<Enum: {'CLOCK_EDGE_RISING', 'CLOCK_EDGE_FALLING'}>"},
'decimation': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_decimation',
'value': 1,
'raw_value': 1,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'name': 'decimation',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Decimation',
'inter_delay': 0,
'vals': '<Ints 0<=v<=100000>'},
'coupling1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_coupling1',
'value': 'DC',
'raw_value': 2,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'AC': 1, 'DC': 2},
'name': 'coupling1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Coupling channel 1',
'inter_delay': 0,
'vals': "<Enum: {'DC', 'AC'}>"},
'channel_range1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_channel_range1',
'value': 0.4,
'raw_value': 7,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'val_mapping': {0.4: 7},
'name': 'channel_range1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'V',
'label': 'Range channel 1',
'inter_delay': 0,
'vals': '<Enum: {0.4}>'},
'impedance1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_impedance1',
'value': 50,
'raw_value': 2,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {50: 2},
'name': 'impedance1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'Ohm',
'label': 'Impedance channel 1',
'inter_delay': 0,
'vals': '<Enum: {50}>'},
'bwlimit1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_bwlimit1',
'value': 'DISABLED',
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 1},
'name': 'bwlimit1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Bandwidth limit channel 1',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'coupling2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_coupling2',
'value': 'DC',
'raw_value': 2,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'AC': 1, 'DC': 2},
'name': 'coupling2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Coupling channel 2',
'inter_delay': 0,
'vals': "<Enum: {'DC', 'AC'}>"},
'channel_range2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_channel_range2',
'value': 0.4,
'raw_value': 7,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {0.4: 7},
'name': 'channel_range2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'V',
'label': 'Range channel 2',
'inter_delay': 0,
'vals': '<Enum: {0.4}>'},
'impedance2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_impedance2',
'value': 50,
'raw_value': 2,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {50: 2},
'name': 'impedance2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'Ohm',
'label': 'Impedance channel 2',
'inter_delay': 0,
'vals': '<Enum: {50}>'},
'bwlimit2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_bwlimit2',
'value': 'DISABLED',
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 1},
'name': 'bwlimit2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Bandwidth limit channel 2',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'trigger_operation': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_operation',
'value': 'TRIG_ENGINE_OP_J',
'raw_value': 0,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'TRIG_ENGINE_OP_J': 0,
'TRIG_ENGINE_OP_K': 1,
'TRIG_ENGINE_OP_J_OR_K': 2,
'TRIG_ENGINE_OP_J_AND_K': 3,
'TRIG_ENGINE_OP_J_XOR_K': 4,
'TRIG_ENGINE_OP_J_AND_NOT_K': 5,
'TRIG_ENGINE_OP_NOT_J_AND_K': 6},
'name': 'trigger_operation',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Operation',
'inter_delay': 0,
'vals': "<Enum: {'TRIG_ENGINE_OP_J', 'TRIG_ENGINE_OP_J_OR_K', 'TRIG_ENGINE_OP_J_XOR_K', 'TRIG_ENGINE_OP_NOT_J_AND_K', 'TRIG_ENGINE_OP_J_AND_K', 'TRIG_ENGINE_OP_J_AND_NOT_K', 'TRIG_ENGINE_OP_K'}>"},
'trigger_engine1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_engine1',
'value': 'TRIG_ENGINE_J',
'raw_value': 0,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'TRIG_ENGINE_J': 0, 'TRIG_ENGINE_K': 1},
'name': 'trigger_engine1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Engine 1',
'inter_delay': 0,
'vals': "<Enum: {'TRIG_ENGINE_K', 'TRIG_ENGINE_J'}>"},
'trigger_source1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_source1',
'value': 'EXTERNAL',
'raw_value': 2,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'CHANNEL_A': 0,
'CHANNEL_B': 1,
'EXTERNAL': 2,
'DISABLE': 3},
'name': 'trigger_source1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Source 1',
'inter_delay': 0,
'vals': "<Enum: {'DISABLE', 'EXTERNAL', 'CHANNEL_A', 'CHANNEL_B'}>"},
'trigger_slope1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_slope1',
'value': 'TRIG_SLOPE_POSITIVE',
'raw_value': 1,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'TRIG_SLOPE_POSITIVE': 1, 'TRIG_SLOPE_NEGATIVE': 2},
'name': 'trigger_slope1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Slope 1',
'inter_delay': 0,
'vals': "<Enum: {'TRIG_SLOPE_NEGATIVE', 'TRIG_SLOPE_POSITIVE'}>"},
'trigger_level1': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_level1',
'value': 160,
'raw_value': 160,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'name': 'trigger_level1',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Level 1',
'inter_delay': 0,
'vals': '<Ints 0<=v<=255>'},
'trigger_engine2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_engine2',
'value': 'TRIG_ENGINE_K',
'raw_value': 1,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'TRIG_ENGINE_J': 0, 'TRIG_ENGINE_K': 1},
'name': 'trigger_engine2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Engine 2',
'inter_delay': 0,
'vals': "<Enum: {'TRIG_ENGINE_K', 'TRIG_ENGINE_J'}>"},
'trigger_source2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_source2',
'value': 'DISABLE',
'raw_value': 3,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'CHANNEL_A': 0,
'CHANNEL_B': 1,
'EXTERNAL': 2,
'DISABLE': 3},
'name': 'trigger_source2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Source 2',
'inter_delay': 0,
'vals': "<Enum: {'DISABLE', 'EXTERNAL', 'CHANNEL_A', 'CHANNEL_B'}>"},
'trigger_slope2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_slope2',
'value': 'TRIG_SLOPE_POSITIVE',
'raw_value': 1,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'TRIG_SLOPE_POSITIVE': 1, 'TRIG_SLOPE_NEGATIVE': 2},
'name': 'trigger_slope2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Slope 2',
'inter_delay': 0,
'vals': "<Enum: {'TRIG_SLOPE_NEGATIVE', 'TRIG_SLOPE_POSITIVE'}>"},
'trigger_level2': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_level2',
'value': 128,
'raw_value': 128,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'name': 'trigger_level2',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Level 2',
'inter_delay': 0,
'vals': '<Ints 0<=v<=255>'},
'external_trigger_coupling': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_external_trigger_coupling',
'value': 'DC',
'raw_value': 2,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'AC': 1, 'DC': 2},
'name': 'external_trigger_coupling',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'External Trigger Coupling',
'inter_delay': 0,
'vals': "<Enum: {'DC', 'AC'}>"},
'external_trigger_range': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_external_trigger_range',
'value': 'ETR_2V5',
'raw_value': 3,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'ETR_TTL': 2, 'ETR_2V5': 3},
'name': 'external_trigger_range',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'External Trigger Range',
'inter_delay': 0,
'vals': "<Enum: {'ETR_TTL', 'ETR_2V5'}>"},
'trigger_delay': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_trigger_delay',
'value': 0,
'raw_value': 0,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'name': 'trigger_delay',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'Sample clock cycles',
'label': 'Trigger Delay',
'inter_delay': 0,
'vals': '<Ints v>=0, Multiples of 8>'},
'timeout_ticks': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_timeout_ticks',
'value': 0,
'raw_value': 0,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'name': 'timeout_ticks',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '10 us',
'label': 'Timeout Ticks',
'inter_delay': 0,
'vals': '<Ints v>=0>'},
'aux_io_mode': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_aux_io_mode',
'value': 'AUX_IN_AUXILIARY',
'raw_value': 13,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'AUX_OUT_TRIGGER': 0,
'AUX_IN_TRIGGER_ENABLE': 1,
'AUX_IN_AUXILIARY': 13},
'name': 'aux_io_mode',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'AUX I/O Mode',
'inter_delay': 0,
'vals': "<Enum: {'AUX_OUT_TRIGGER', 'AUX_IN_TRIGGER_ENABLE', 'AUX_IN_AUXILIARY'}>"},
'aux_io_param': {'__class__': 'qcodes.instrument_drivers.AlazarTech.utils.TraceParameter',
'full_name': 'Alazar1_aux_io_param',
'value': 'NONE',
'raw_value': 0,
'ts': '2020-11-26 13:52:50',
'post_delay': 0,
'val_mapping': {'NONE': 0,
'TRIG_SLOPE_POSITIVE': 1,
'TRIG_SLOPE_NEGATIVE': 2},
'name': 'aux_io_param',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'AUX I/O Param',
'inter_delay': 0,
'vals': "<Enum: {'TRIG_SLOPE_NEGATIVE', 'TRIG_SLOPE_POSITIVE', 'NONE'}>"},
'mode': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_mode',
'value': 'NPT',
'raw_value': 512,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'val_mapping': {'NPT': 512, 'TS': 1024},
'name': 'mode',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Acquisition mode',
'inter_delay': 0,
'vals': "<Enum: {'TS', 'NPT'}>"},
'samples_per_record': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_samples_per_record',
'value': 1024,
'raw_value': 1024,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'name': 'samples_per_record',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Samples per Record',
'inter_delay': 0,
'vals': '<Ints v>=256, Multiples of 128>'},
'records_per_buffer': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_records_per_buffer',
'value': 74,
'raw_value': 74,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'name': 'records_per_buffer',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Records per Buffer',
'inter_delay': 0,
'vals': '<Ints v>=0>'},
'buffers_per_acquisition': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_buffers_per_acquisition',
'value': 1,
'raw_value': 1,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'name': 'buffers_per_acquisition',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Buffers per Acquisition',
'inter_delay': 0,
'vals': '<Ints v>=0>'},
'channel_selection': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_channel_selection',
'value': 'AB',
'raw_value': 3,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'A': 1, 'B': 2, 'AB': 3},
'name': 'channel_selection',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Channel Selection',
'inter_delay': 0,
'vals': "<Enum: {'B', 'A', 'AB'}>"},
'transfer_offset': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_transfer_offset',
'value': 0,
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'name': 'transfer_offset',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'Samples',
'label': 'Transfer Offset',
'inter_delay': 0,
'vals': '<Ints v>=0>'},
'external_startcapture': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_external_startcapture',
'value': 'ENABLED',
'raw_value': 1,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 1},
'name': 'external_startcapture',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'External Startcapture',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'enable_record_headers': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_enable_record_headers',
'value': 'DISABLED',
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 8},
'name': 'enable_record_headers',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Enable Record Headers',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'alloc_buffers': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_alloc_buffers',
'value': 'DISABLED',
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 32},
'name': 'alloc_buffers',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Alloc Buffers',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'fifo_only_streaming': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_fifo_only_streaming',
'value': 'DISABLED',
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 2048},
'name': 'fifo_only_streaming',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Fifo Only Streaming',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'interleave_samples': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_interleave_samples',
'value': 'DISABLED',
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 4096},
'name': 'interleave_samples',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Interleave Samples',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'get_processed_data': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_get_processed_data',
'value': 'DISABLED',
'raw_value': 0,
'ts': '2020-11-26 13:52:42',
'post_delay': 0,
'val_mapping': {'DISABLED': 0, 'ENABLED': 8192},
'name': 'get_processed_data',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Get Processed Data',
'inter_delay': 0,
'vals': "<Enum: {'ENABLED', 'DISABLED'}>"},
'allocated_buffers': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_allocated_buffers',
'value': 1,
'raw_value': 1,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'name': 'allocated_buffers',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Allocated Buffers',
'inter_delay': 0,
'vals': '<Ints v>=0>'},
'buffer_timeout': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_buffer_timeout',
'value': 1000,
'raw_value': 1000,
'ts': '2020-11-26 13:53:42',
'post_delay': 0,
'name': 'buffer_timeout',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': 'ms',
'label': 'Buffer Timeout',
'inter_delay': 0,
'vals': '<Ints v>=0>'},
'trigger_holdoff': {'__class__': 'qcodes.instrument.parameter.Parameter',
'full_name': 'Alazar1_trigger_holdoff',
'value': None,
'raw_value': None,
'ts': None,
'post_delay': 0,
'name': 'trigger_holdoff',
'instrument': 'qcodes.instrument_drivers.AlazarTech.ATS9360.AlazarTech_ATS9360',
'instrument_name': 'Alazar1',
'unit': '',
'label': 'Trigger Holdoff',
'inter_delay': 0,
'vals': '<Boolean>'}},
'name': 'Alazar1'}
Finally, the following shows that Alazar driver and its acquisition controller work well within a the QCoDeS measurement using a dummy parameter and do1d function:
[10]:
dummy = ManualParameter(name="dummy")
do1d(dummy, 0, 50, 51, 0.5, acquisition_controller.acquisition, do_plot=False)
Starting experimental run with id: 20.
[10]:
(results #20@C:\Users\Farzad\experiments.db
------------------------------------------
dummy - numeric
acquisition_controller_acquisition - numeric,
[None],
[None])
Let’s have a quick look at the acquisition result:
[11]:
plot_by_id(20)
[11]:
([<AxesSubplot:title={'center':'Run #20, Experiment exp (no sample1)'}, xlabel='dummy', ylabel='acquisition'>],
[None])
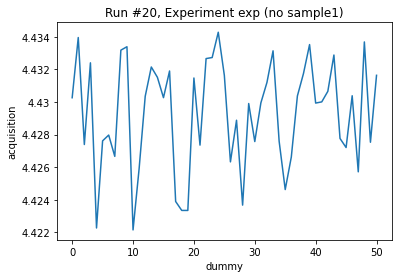
Looking for extra functionalities?
It is expected that the users will implement acquisitions to fit their needs. One can visit this link, where other controller examples for Alazar can be found. Also, it might be useful to check out this notebook to get to know how to use these controllers.