TIP
🔥 Make sure you star the repo (opens new window) to keep up to date with new tips and tricks.
💡 Learn more : Azure Event Grid overview (opens new window).
📺 Watch the video : How to receive Azure Event Grid events in an Azure Function (opens new window).
# How to receive Azure Event Grid events in an Azure Function
# Event-driven applications
Many modern applications are distributed and are event-driven. This means that processes in the application execute when an event is received, like an event that gets raised when a new file is uploaded. Event-driven applications promote high availability, resilience, and only run when they need to. Azure Event Grid (opens new window) is the central service in Azure that enables event-driven applications. It provides an event gateway that connects events from Azure and third-party services to event handlers, like your application.
In this post, we'll use an Azure Function (opens new window) to receive an event from Azure Event Grid (opens new window).
# Prerequisites
If you want to follow along, you'll need the following:
- An Azure subscription (If you don't have an Azure subscription, create a free account (opens new window) before you begin)
- An existing Azure Function app (opens new window). Learn how to create one in this quickstart (opens new window)
# Use an Azure Function as event handler
We'll use an existing Azure Function App, and create a new Function in it that will receive events from Azure Event Grid.
- Go to the Azure portal (opens new window) and navigate to an existing Azure Function app
- Navigate to the Functions menu
- Click the "+ Create" button to start creating a new Function
- Leave the Development environment to "Develop in portal"
- Select the "Azure Event Grid trigger" template
- Enter a Name for the Function
- Click Create
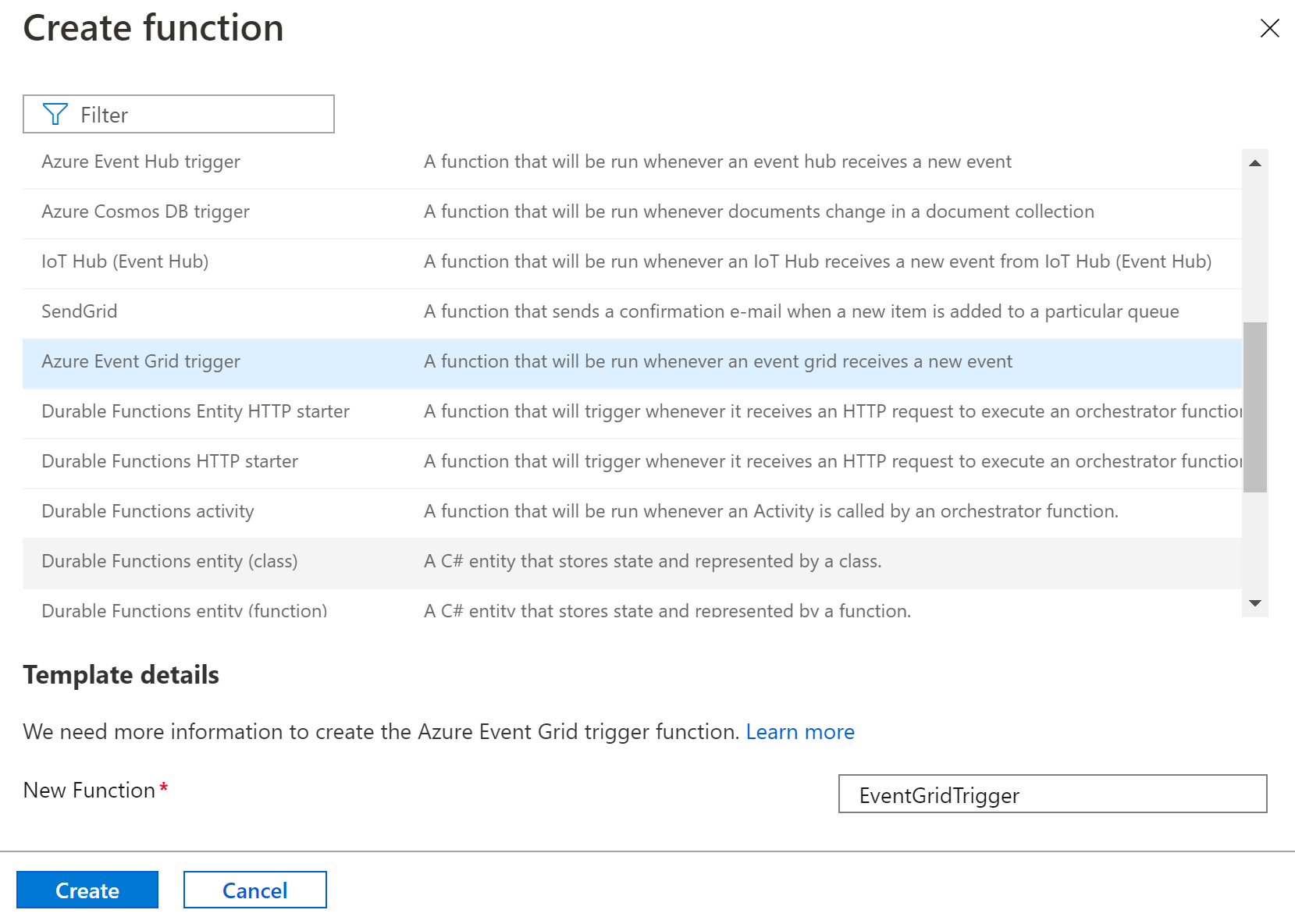
(Create a new Function in the Azure portal)
The Function will act as the event handler. Now, we need to create an Event Grid Topic. We can use this to raise events.
- In the Azure portal, use the search bar to search for "Event Grid Topics" and select the result
- Click the "+ Create" button to Create a new Topic
- Select a Resource group
- Enter a Name for the Topic
- Pick a Region
- Click Review + create and then Create
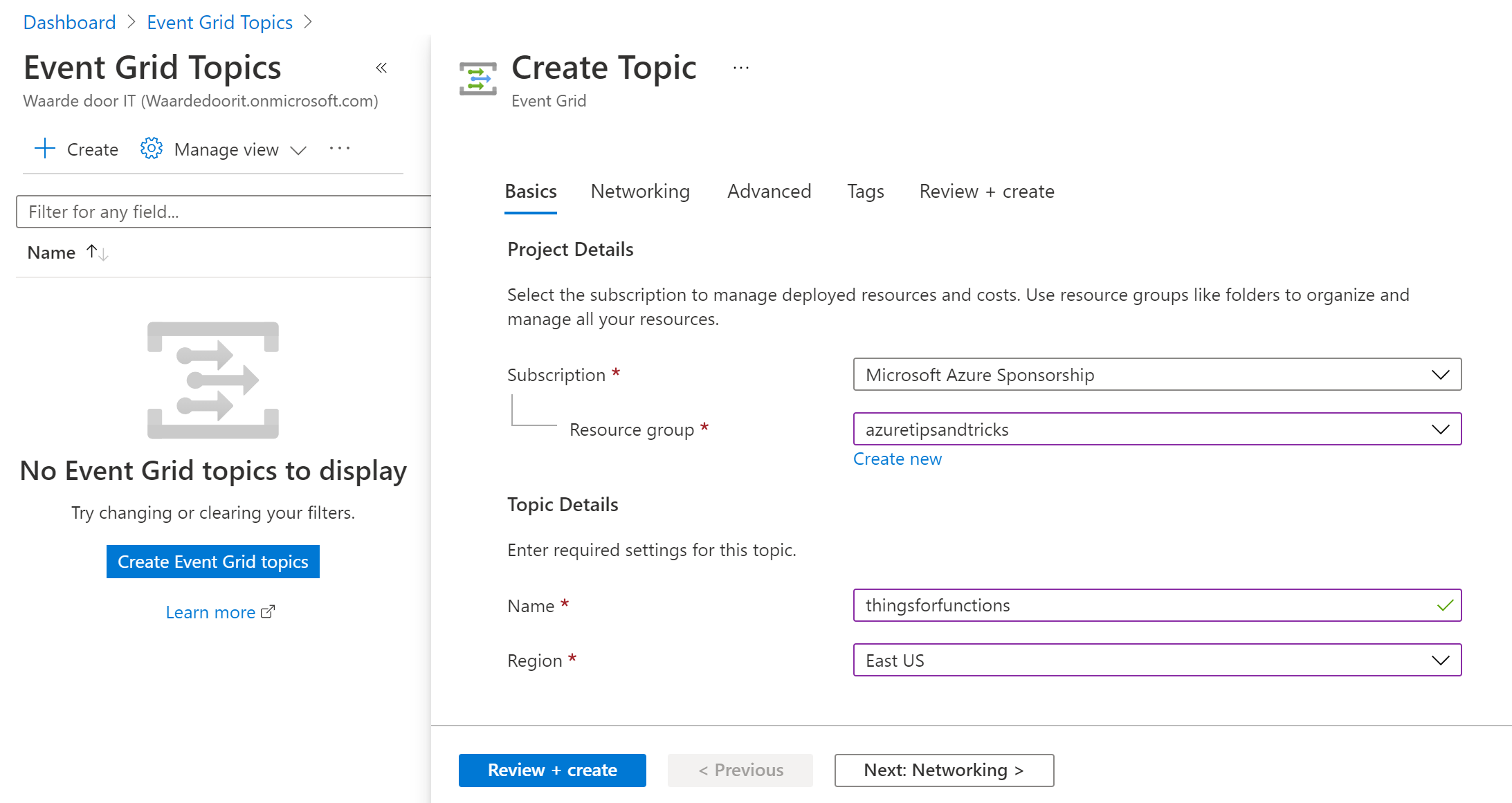
(Create a new Event Grid Topic in the Azure portal)
- When the Topic is created, you'll automatically navigate to its overview blade. We need to create a subscription for this topic, so that the event handler (the Function) is subscribed to the events of the topic. Click the "+ Event Subscription" button to start creating a new subscription
- Enter a Name for the subscription
- For Endpoint Type, select Azure Function
- Select the Function that we created earlier for the Endpoint
- Click Create to create the subscription
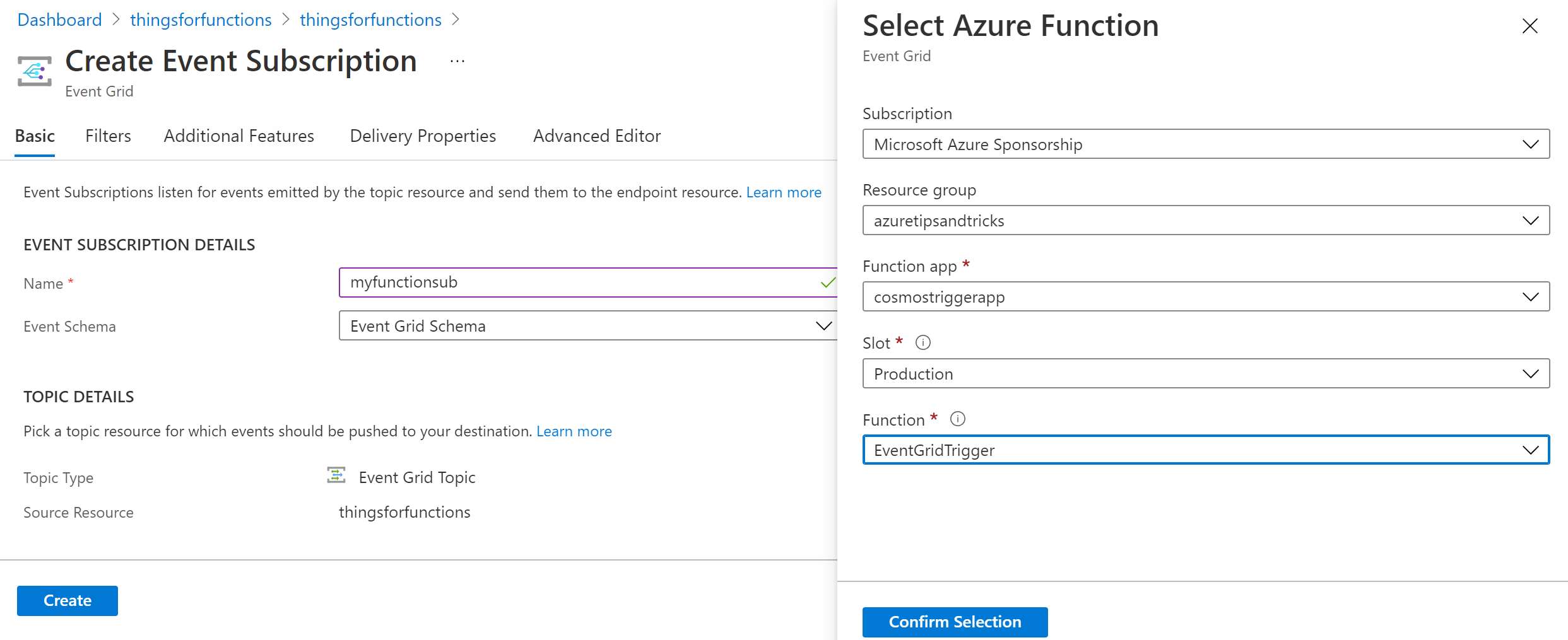
(Create an Event subscription in the Azure portal)
The Function is now subscribed to events that are raised for the Event Grid Topic. Let's create an event manually to trigger the Function.
- In the Azure portal, open the Azure Cloud Shell (or go to https://shell.azure.com/ (opens new window))
- Select PowerShell as the language
- Execute the following script to set parameters for the resource group and Event Grid Topic name. Enter the values of the Event Grid Topic
$resourceGroupName = <resource group name>
$topicName = <topic name>
2
- Now execute the following command. This creates an event for the Event Grid Topic
$endpoint = (Get-AzEventGridTopic -ResourceGroupName $resourceGroupName -Name $topicName).Endpoint
$keys = Get-AzEventGridTopicKey -ResourceGroupName $resourceGroupName -Name $topicName
$eventID = Get-Random 99999
#Date format should be SortableDateTimePattern (ISO 8601)
$eventDate = Get-Date -Format s
#Construct body using Hashtable
$htbody = @{
id= $eventID
eventType="recordInserted"
subject="myapp/vehicles/motorcycles"
eventTime= $eventDate
data= @{
make="Ducati"
model="Monster"
}
dataVersion="1.0"
}
#Use ConvertTo-Json to convert event body from Hashtable to JSON Object
#Append square brackets to the converted JSON payload since they are expected in the event's JSON payload syntax
$body = "["+(ConvertTo-Json $htbody)+"]"
Invoke-WebRequest -Uri $endpoint -Method POST -Body $body -Headers @{"aeg-sas-key" = $keys.Key1}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
- Go back to the Azure Function and click on the Monitor menu, or look at the Log in the Code blade. You'll see that the Function was triggered when the event was raised, and that it received the data in the event
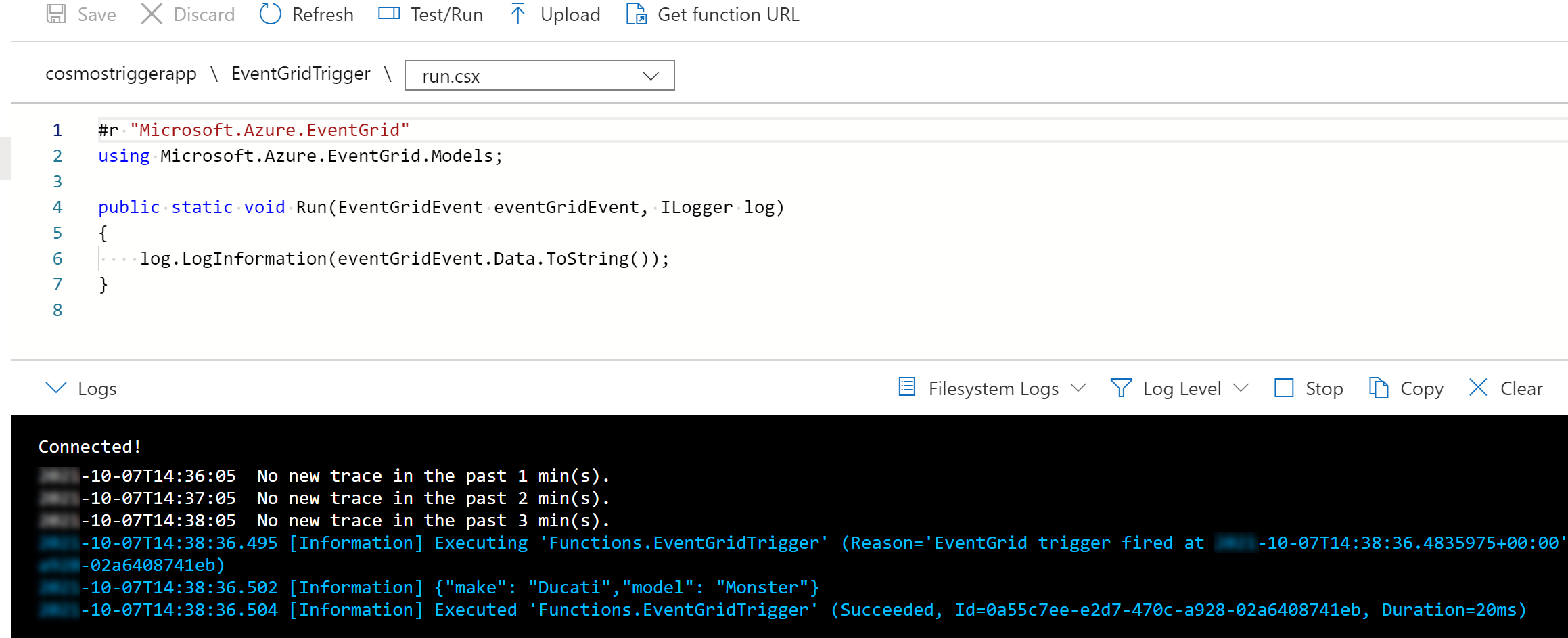
(Azure Function triggered by the event)
# Conclusion
Azure Event Grid (opens new window) is the glue for event-driven, serverless applications that are highly available and only run when they are needed. Go and check it out!