TIP
🔥 Make sure you star the repo (opens new window) to keep up to date with new tips and tricks.
💡 Learn more : Azure Maps overview (opens new window).
📺 Watch the video : How to get started with Azure Maps (opens new window).
# How to get started with Azure Maps
# Realtime maps services
Displaying a map and interacting with it is an often used feature for mobile and web applications. Azure Maps (opens new window) enables that usecase, and more.
Azure Maps offers many geospatial services that you can use through REST APIs and SDKs, including:
- The rendering of map data, including satellite imagery
- Creator services that enable you to create custom maps, e.g. for indoor maps
- Elevation services
- Weather services
- Traffic services
- Routing services
- Mobility services that you can use to plan public transport routes
- Time zone and geolocation services, and more
Azure Maps (opens new window) is a comprehensive offering. In this post, we'll create an Azure Maps Account and use that to create a simple map in an HTML page.
# Prerequisites
If you want to follow along, you'll need the following:
- An Azure subscription (If you don't have an Azure subscription, create a free account (opens new window) before you begin)
# Rendering a map on an HTML page
We'll start by creating an Azure Map Account through the Azure portal.
- Go to the Azure portal (opens new window)
- Click the Create a resource button (the plus-sign in the top left corner)
- Search for Azure Maps, select the "Azure Maps" result and click Create
- Select a Resource Group
- Fill in a Name
- Select a Pricing tier. The Gen2 tier is fine for this exercise
- Check the box to confirm that you agree to the license and privacy statement
- Click Create to create the Azure Maps Account
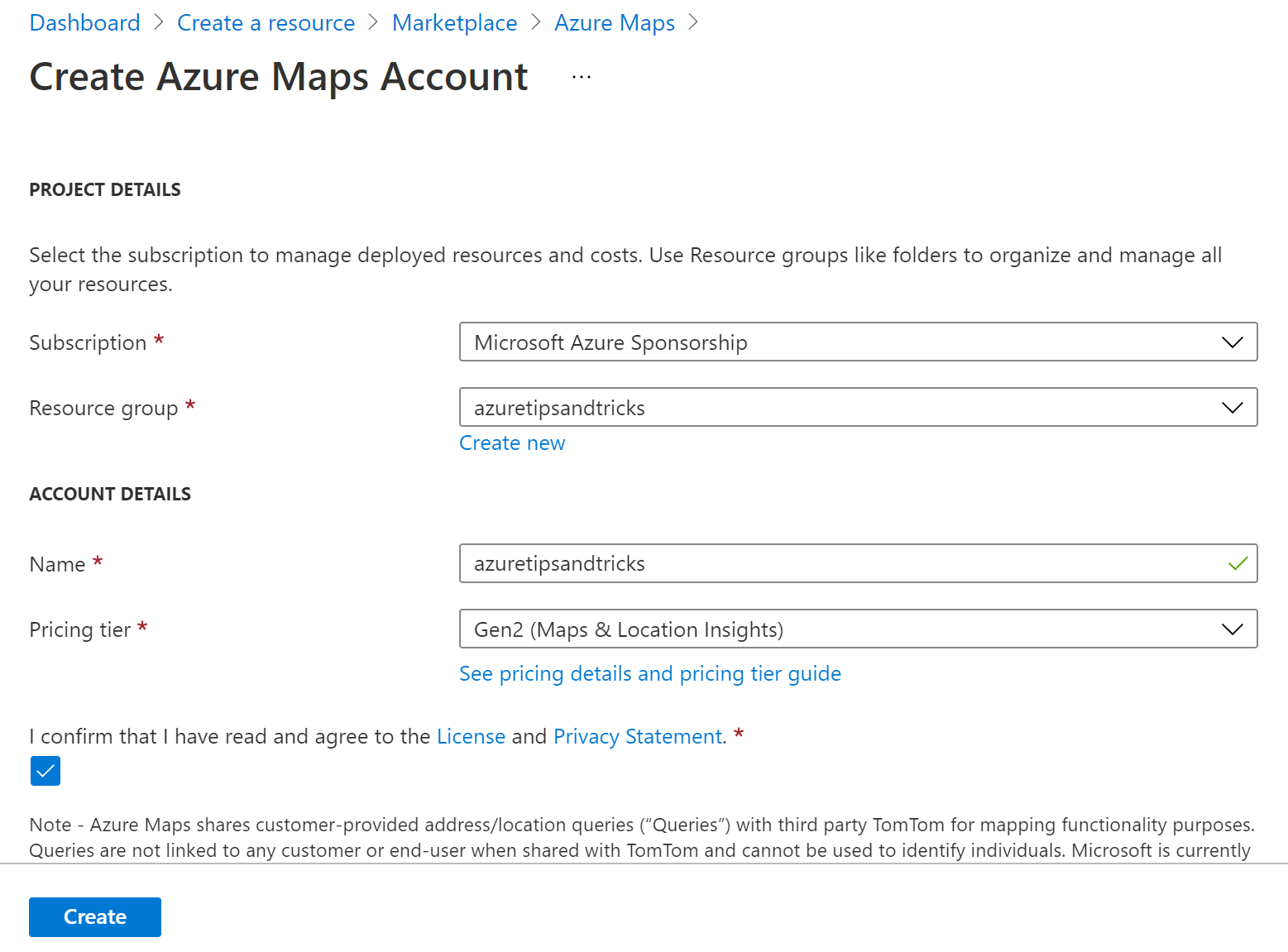
(Create an Azure Maps Account in the Azure portal)
When the Azure Maps Account is created, navigate to it in the Azure portal. We need the shared authentication key to be able to use it in an HTML page. Navigate to the Authentication menu, and copy the Primary Key.
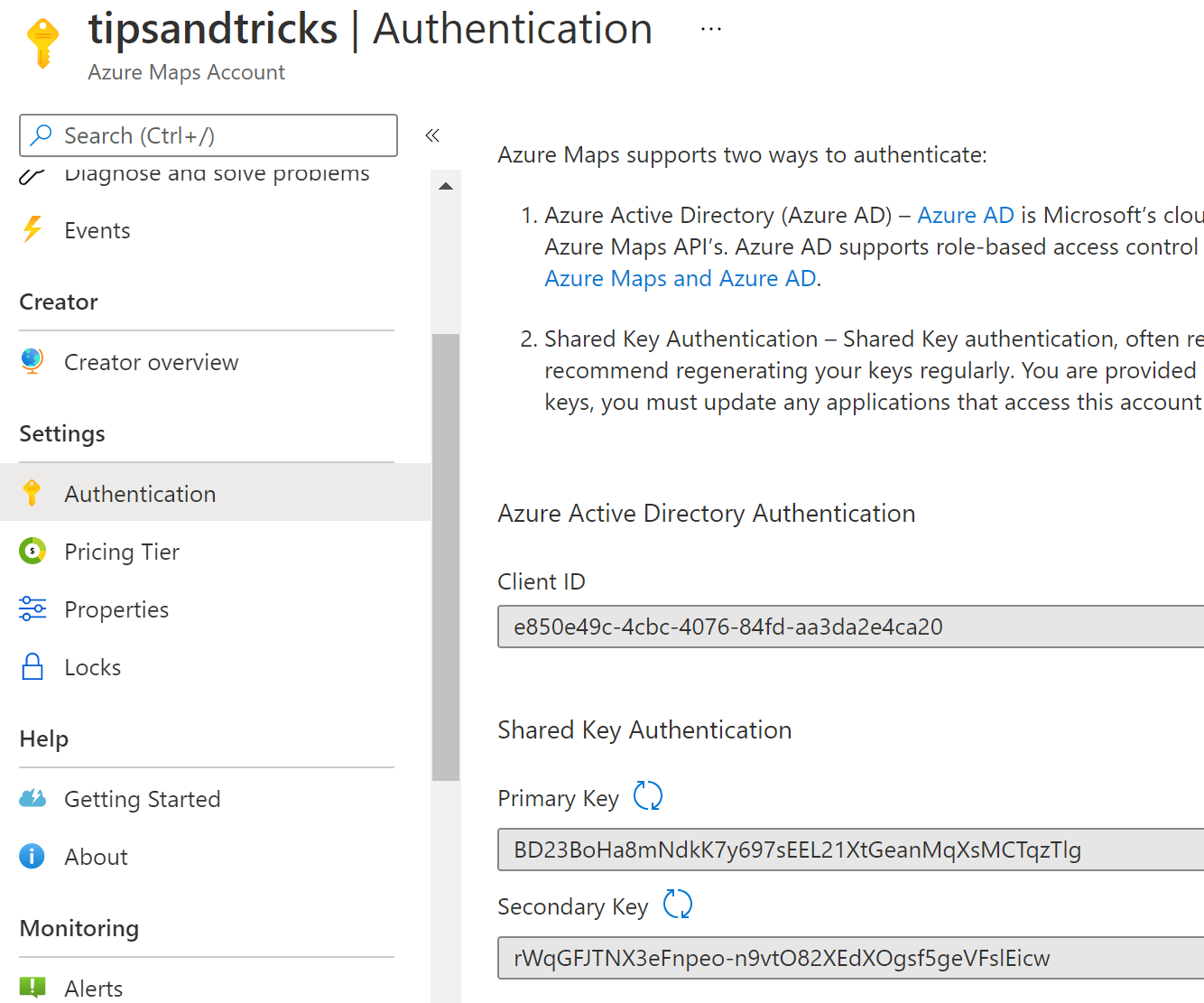
(Azure Maps authentication keys)
We'll use a sample HTML page that renders a map based on search results.
- Go to the HTML sample page on GitHub (opens new window) and copy the code
- Create a local file called index.html
- Paste the HTML code into the index.html file
- Replace the GetMap function with the code below, and fill in your primary authentication key. This code initializes a map inside the <div> element with the id myMap
function GetMap() {
//Initialize a map instance.
map = new atlas.Map('myMap', {
center: [-118.270293, 34.039737],
zoom: 14,
view: 'Auto',
//Add authentication details for connecting to Azure Maps.
authOptions: {
//Use an Azure Maps key. Get an Azure Maps key at https://azure.com/maps. NOTE: The primary key should be used as the key.
authType: 'subscriptionKey',
subscriptionKey: 'BD23BoHa8mNdkK7y697sEEL21XtGeanMqXsMCTqzTlg'
}
});
2
3
4
5
6
7
8
9
10
11
12
13
14
15
- Save the HTML file and open it in a browser. You should see a map being rendered, and a search box. Search for a place or thing and click on the result
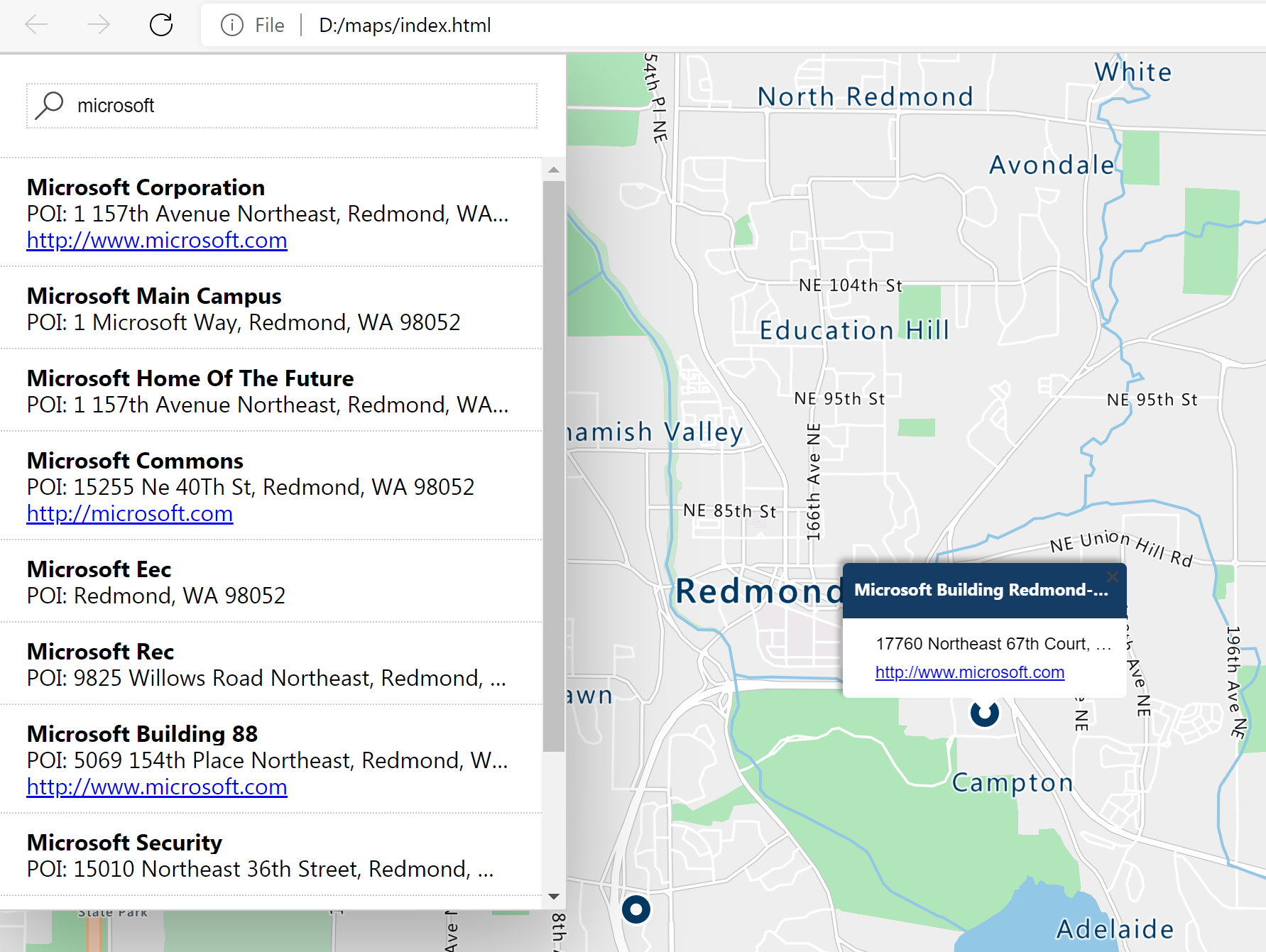
(Map and search result in the HTML page)
In the JavaScript Search function in the HTML file, it calls the Azure Maps APIs with the Azure Maps JavaScript Web SDK, which it gets from the references below. It also comes with a CSS file to help with map rendering and support images:
<!-- Add references to the Azure Maps Map control JavaScript and CSS files. -->
<link rel="stylesheet" href="https://atlas.microsoft.com/sdk/javascript/mapcontrol/2/atlas.min.css" type="text/css" />
<script src="https://atlas.microsoft.com/sdk/javascript/mapcontrol/2/atlas.min.js"></script>
<!-- Add a reference to the Azure Maps Services Module JavaScript file. -->
<script src="https://atlas.microsoft.com/sdk/javascript/service/2/atlas-service.min.js"></script>
2
3
4
5
6
The search function works by calling the searchPOI (opens new window) method of the Azure Maps search API (opens new window), and displaying the results as an HTML list.
function search() {
//Remove any previous results from the map.
datasource.clear();
popup.close();
resultsPanel.innerHTML = '';
//Use MapControlCredential to share authentication between a map control and the service module.
var pipeline = atlas.service.MapsURL.newPipeline(new atlas.service.MapControlCredential(map));
//Construct the SearchURL object
var searchURL = new atlas.service.SearchURL(pipeline);
var query = document.getElementById("search-input").value;
searchURL.searchPOI(atlas.service.Aborter.timeout(10000), query, {
lon: map.getCamera().center[0],
lat: map.getCamera().center[1],
maxFuzzyLevel: 4,
view: 'Auto'
}).then((results) => {
//Extract GeoJSON feature collection from the response and add it to the datasource
var data = results.geojson.getFeatures();
datasource.add(data);
if (centerMapOnResults) {
map.setCamera({
bounds: data.bbox
});
}
console.log(data);
//Create the HTML for the results list.
var html = [];
for (var i = 0; i < data.features.length; i++) {
var r = data.features[i];
html.push('<li onclick="itemClicked(\'', r.id, '\')" onmouseover="itemHovered(\'', r.id, '\')">')
html.push('<div class="title">');
if (r.properties.poi && r.properties.poi.name) {
html.push(r.properties.poi.name);
} else {
html.push(r.properties.address.freeformAddress);
}
html.push('</div><div class="info">', r.properties.type, ': ', r.properties.address.freeformAddress, '</div>');
if (r.properties.poi) {
if (r.properties.phone) {
html.push('<div class="info">phone: ', r.properties.poi.phone, '</div>');
}
if (r.properties.poi.url) {
html.push('<div class="info"><a href="http://', r.properties.poi.url, '">http://', r.properties.poi.url, '</a></div>');
}
}
html.push('</li>');
resultsPanel.innerHTML = html.join('');
}
});
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
# Conclusion
Azure Maps (opens new window) is a powerful set of geospatial services that enable you to render maps, traffic, weather, public transport information, geofencing, and more. You can use Azure Maps services through Web (opens new window) and Android SDKs (opens new window), and by calling REST APIs (opens new window). Go and check it out!