Create a Coded UI Test using Selenium in Visual Studio
If you performed the previous lab, you will have used a screen recorder to create a coded UI test. While screen recorders are convenient, they often do not provide the level of control needed for more sophisticated testing.
In this lab, you will learn to create a coded UI test based on Selenium. The Selenium automation tools speak a language known as Selenese. Rather than issuing Selenese directly to the different types of browsers, web drivers are used to perform the translation. In this lab, you will install the Chrome, IE, and Firefox drivers, then automate the code from within the C# language in Visual Studio.
Note that there is a Selenium IDE that can be used for recording web actions but using it is not currently recommended. It only works with older insecure versions of FireFox (up to version 5.4). In this lab, we will use the drivers and classes directly from code.
For the purposes of the lab, we will use the PartsUnlimited web application hosted on Azure to run this lab.
There have been reports that the keyword search on the home page is intermittently performing poorly. We will investigate how the keyword search on the home page performs, both by entering values into the box and clicking the search icon, and by directly referencing the search term a URL.
DevOps MPP Course Source
- This lab is used in course DevOps200.5x: DevOps Testing - Module 5.
Lab Video:
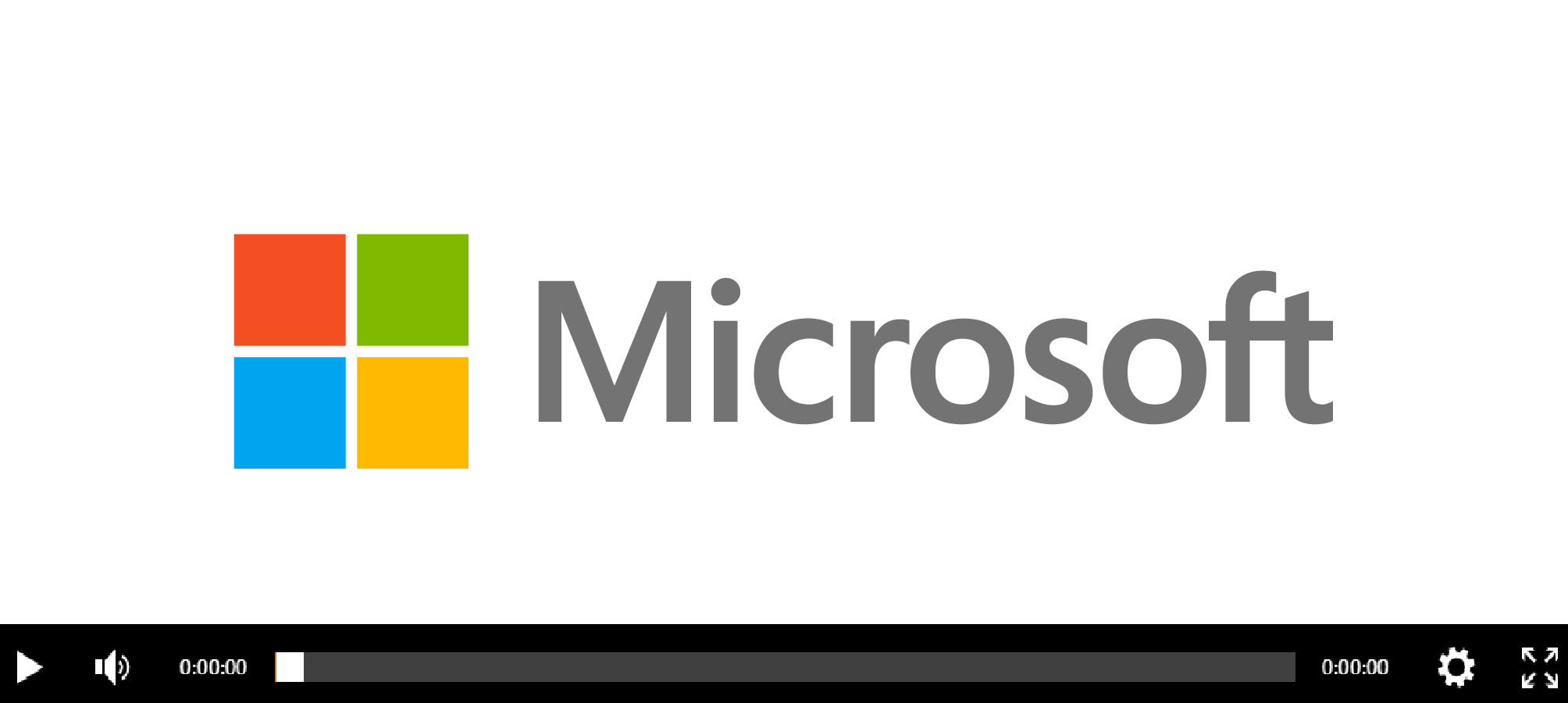
Pre-requisites:
- Visual Studio 2017
- Google Chrome (recent edition) https://www.google.com.au/chrome/browser/features.html
- Access to the PartsUnlimited website deployed at http://cdrm-pu-demo-dev.azurewebsites.net/
Lab Tasks:
- Create a coded UI web test in C#
Estimated Lab Time:
- approx. 30 minutes
Learn to use the Parts Unlimited Search Functionality
- In a Chrome browser, launch the PartsUnlimited web application by going to the URL http://cdrm-pu-demo-dev.azurewebsites.net/
-
This opens the PartsUnlimited application as shown below.
Ensure that the website opens succesfully.
-
On the home page, in the Search box, enter light and click the Search icon.
-
Note that three products are returned as expected.
-
Also note in the browser search bar that the search item has been added to the URL. We also want to test that direct access to the search works.
-
Click the Parts Unlimited heading to return to the home page.
Locate the IDs of the required elements
-
While in the home page, click F12 to open the developer tools.
-
In the top section of the developer tools, click on the tool to select screen elements.
-
Hover over the search box and note its ID.
Note that the name is “search-box”.
-
Hover over the search icon and note its ID.
Note that the name is “search-link”.
These are the elements that we will automate.
Create the test in Visual Studio
-
Launch Visual Studio Enterprise.
-
From the File menu, click New then Project. In the New Project window, choose a Unit Test Project from the Test category.
-
Add a name for the project, and solution, and a location for the project.
-
In Solution Explorer, right-click References and click Manage NuGet Packages.
-
In the NuGet manager, click Browse, enter selenium as the search term and hit Enter to start the search.
- Install the following packages, then close the NuGet manager window:
- Selenium.WebDriver
- Selenium.WebDriver.ChromeDriver
- Selenium.WebDriver.IEDriver
- Selenium.Firefox.WebDriver
- Selenium.WebDriver.PhantomJS.Xplatform
-
In Solution Explorer, right-click UnitTest1.cs and rename it to PU_SearchTests.cs.
-
When prompted to rename all references, click Yes.
-
Add the following using statements after the ones that are already there:
using OpenQA.Selenium; using OpenQA.Selenium.Chrome; using OpenQA.Selenium.Firefox; using OpenQA.Selenium.IE; using OpenQA.Selenium.Remote; using OpenQA.Selenium.PhantomJS;
-
Your code should look like this:
-
Add the following declarations to the beginning of the class. Note that the websiteURL and the required browser should really be parameters but this will be ok for our test:
private string websiteURL = @"http://cdrm-pu-demo-dev.azurewebsites.net"; private RemoteWebDriver browserDriver; public TestContext TestContext { get; set; }
-
Your code should look like this:
-
Add the following initialize and cleanup methods:
[TestInitialize()] public void PU_SearchTests_Initialize() { // nothing for now } [TestCleanup()] public void PU_SearchTests_Cleanup() { browserDriver.Quit(); }
-
Your code should look this this:
-
Replace the test method with the following code:
[TestMethod] [TestCategory("Selenium")] public void SearchForLights() { browserDriver = new ChromeDriver(); browserDriver.Manage().Window.Maximize(); browserDriver.Manage().Timeouts().ImplicitlyWait(TimeSpan.FromSeconds(20)); browserDriver.Navigate().GoToUrl(this.websiteURL); }
-
Your code should look this this:
At this point, we have a test that should just start the browser (a Chrome browser), maximize the window, set the timeout, and go to the Parts Unlimited website. We can test it up to this point.
-
From the Build menu, click Build Solution and make sure the solution builds correctly.
-
In Test Explorer (if not visible, from the View menu, click to open it), right-click the SearchForLights test, and click Run Selected Tests to execute the test.
-
You should see the browser open, maximize (if not already), then go to the Parts Unlimited home page.
-
Add the following statements to the test method:
browserDriver.FindElementById("search-box").Clear(); browserDriver.FindElementById("search-box").SendKeys("light"); browserDriver.FindElementById("search-link").Click();
-
Your code should look like this:
-
This will now clear the search box, type the value “light” and search for it.
-
From the Build menu, click Build Solution and make sure the solution builds correctly.
-
In Test Explorer, right-click the SearchForLights test, and click Run Selected Tests to execute the test.
You should see the browser open, maximize (if not already), then go to the Parts Unlimited home page, then search for the lights. You will briefly see the search results page appear before the browser closes.
We also needed to check a direct URL search.
-
Add another test method below the current one:
[TestMethod] [TestCategory("Selenium")] public void DirectSearchForLights() { string lightSearchQuery = this.websiteURL + @"/Search?q=light"; browserDriver = new ChromeDriver(); browserDriver.Manage().Window.Maximize(); browserDriver.Manage().Timeouts().ImplicitlyWait(TimeSpan.FromSeconds(20)); browserDriver.Navigate().GoToUrl(lightSearchQuery); }
-
Your code should look like this:
-
From the Build menu, click Build Solution and make sure the solution still builds correctly.
-
In Test Explorer, right-click the DirectSearchForLights test, and click Run Selected Tests to execute the test.
Your new test should also run as expected.
Congratulations! You’ve now completed this lab.
For more information , you can see: Visual Studio: https://aka.ms/edx-devops200.5x-vs01 Web Apps: https://aka.ms/edx-devops200.5x-az03