TIP
🔥 Help shape the future of Azure Tips and Tricks by telling what you'd like us to write about here (opens new window).
💡 Learn more : Azure Table Storage overview (opens new window).
📺 Watch the video : How to choose between Azure Table Storage and Azure Cosmos DB Table API (opens new window).
# How to choose between Azure Table Storage and Azure Cosmos DB Table API
# Semi-structured data storage
You can use Azure Table Storage (opens new window) to store data in rows and columns, in a semi-structured format. Each row can have different columns, and the data isn't linked. Azure Table Storage is a great way to store massive amounts of information, and it is fast and highly available. Azure Cosmos DB (opens new window) also offers table storage through its Table API (opens new window). You can use the same API to use Azure Table Storage and Azure Cosmos DB Table API.
Azure Table Storage and Azure Cosmos DB Table API are very similar, so how do you know which one to use and when?
This post will help you choose between Azure Table Storage (opens new window) and Azure Cosmos DB Table API (opens new window).
# Prerequisites
If you want to follow along, you'll need the following:
- An Azure subscription (If you don't have an Azure subscription, create a free account (opens new window) before you begin)
- An existing Azure Cosmos DB that works with the Table API. Learn how to create one in this quickstart (opens new window)
- An existing Azure Storage Account. Learn how to create one in this quickstart (opens new window)
- The latest version of Visual Studio (opens new window) or VS Code (opens new window)
# Comparing Azure Table Storage to Azure Cosmos DB Table API
To help you choose between Azure Cosmos DB Table API and Azure Table Storage, we'll use a simple application that tests the speed of write- and read operations for both services. We'll use Visual Studio to create a simple console application, and you can use VS Code if you like.
- Open Visual Studio and click Create a new project
- Select Console Application as the application template
- Enter a Name for the project
- Select a Location
- Click Next
- Select the latest Framework version
- Click Create
- Now that we have a project, create a new file in the project called App.config
- Add the following code to the App.config file and replace the values with the connection strings to your existing Azure Table Storage and Azure Cosmos DB
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<appSettings>
<add key="CosmosStorageConnectionString" value="" />
<add key="TableStorageConnectionString" value="" />
</appSettings>
</configuration>
2
3
4
5
6
7
- Next, right-click the project file and select "Manage NuGet Packages"
- Install the package Azure.Data.Tables (opens new window) and System.Configuration.ConfigurationManager. The project structure should now look like the image below
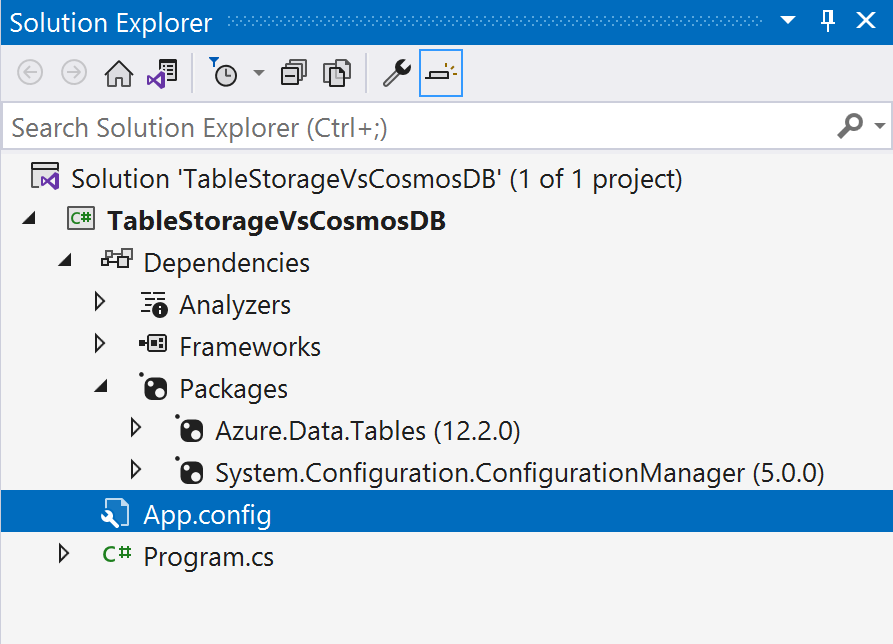
(The project structure in Visual Studio)
- Open Program.cs and replace the code in it with the code below. This connects to Azure Table Storage, creates a Table in it, and creates and reads rows. It does the same for Azure Cosmos DB
namespace TableSBS
{
using Azure;
using Azure.Data.Tables;
using Azure.Data.Tables.Models;
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Diagnostics;
using System.Linq;
/// <summary>
/// This sample program shows how to use the Azure tables SDK to work with tables
/// </summary>
public class Program
{
/// <summary>
/// Run common Table CRUD and query operations using the Azure Cosmos DB endpoints
/// ("premium tables")
/// </summary>
/// <param name="args">Command line arguments</param>
public static void Main(string[] args)
{
string connectionString = ConfigurationManager.AppSettings["CosmosStorageConnectionString"];
int numIterations = 500;
//run against Cosmo DB Table API
TableServiceClient tableClient = new TableServiceClient(connectionString);
Program p = new Program();
p.Run(tableClient, numIterations, "Azure Cosmos DB");
connectionString = ConfigurationManager.AppSettings["TableStorageConnectionString"];
//run against Azure Table Storage
tableClient = new TableServiceClient(connectionString);
p.Run(tableClient, numIterations, "Azure Table Storage");
Console.WriteLine("\n");
Console.WriteLine("Press any key to exit...");
Console.ReadLine();
}
/// <summary>
/// Run a bunch of core Table operations. Each operation is run ~500 times to measure latency.
/// You can swap the endpoint and compare with regular Azure Table storage.
/// </summary>
public void Run(TableServiceClient tableServiceClient, int numIterations, string target)
{
Console.WriteLine("\n");
Console.WriteLine("Creating Table if it doesn't exist, for " + target);
tableServiceClient.DeleteTable("People");
tableServiceClient.CreateTable("People");
List<CustomerEntity> items = new List<CustomerEntity>();
Stopwatch watch = new Stopwatch();
Console.WriteLine("\n");
Console.WriteLine("Running inserts: ");
var tableClient = tableServiceClient.GetTableClient("People");
double latencyInMsnsertLatencyInMs = 0;
for (int i = 0; i < numIterations; i++)
{
watch.Start();
CustomerEntity item = new CustomerEntity()
{
PartitionKey = Guid.NewGuid().ToString(),
RowKey = Guid.NewGuid().ToString(),
Email = $"{GetRandomString(6)}@contoso.com",
PhoneNumber = "425-555-0102",
Bio = GetRandomString(1000)
};
tableClient.AddEntity(item);
latencyInMsnsertLatencyInMs += watch.Elapsed.TotalMilliseconds;
Console.Write($"\r\tInsert # {i}");
items.Add(item);
watch.Reset();
}
Console.Write($"\r\tInsert completed in {latencyInMsnsertLatencyInMs / numIterations} ms on average.");
Console.WriteLine("\n");
Console.WriteLine("Running retrieves: ");
double readLatencyInMs = 0;
for (int i = 0; i < numIterations; i++)
{
watch.Start();
tableClient.GetEntity<CustomerEntity>(items[i].PartitionKey, items[i].RowKey);
readLatencyInMs += watch.Elapsed.TotalMilliseconds;
Console.Write($"\r\tRead # {i}");
watch.Reset();
}
Console.Write($"\r\tRetrieve completed in {readLatencyInMs / numIterations} ms on average.");
}
public string GetRandomString(int length)
{
Random random = new Random(System.Environment.TickCount);
string chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
return new string(Enumerable.Repeat(chars, length).Select(s => s[random.Next(s.Length)]).ToArray());
}
public class CustomerEntity : ITableEntity
{
public CustomerEntity(string lastName, string firstName)
{
this.PartitionKey = lastName;
this.RowKey = firstName;
}
public CustomerEntity() { }
public string Email { get; set; }
public string PhoneNumber { get; set; }
public string Bio { get; set; }
public string PartitionKey { get; set; }
public string RowKey { get; set; }
public DateTimeOffset? Timestamp { get; set; }
public ETag ETag { get; set; }
}
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
- Run the application. You'll see that Azure Cosmos DB Table API is faster than Azure Table Storage. This could make a difference in high-performance applications
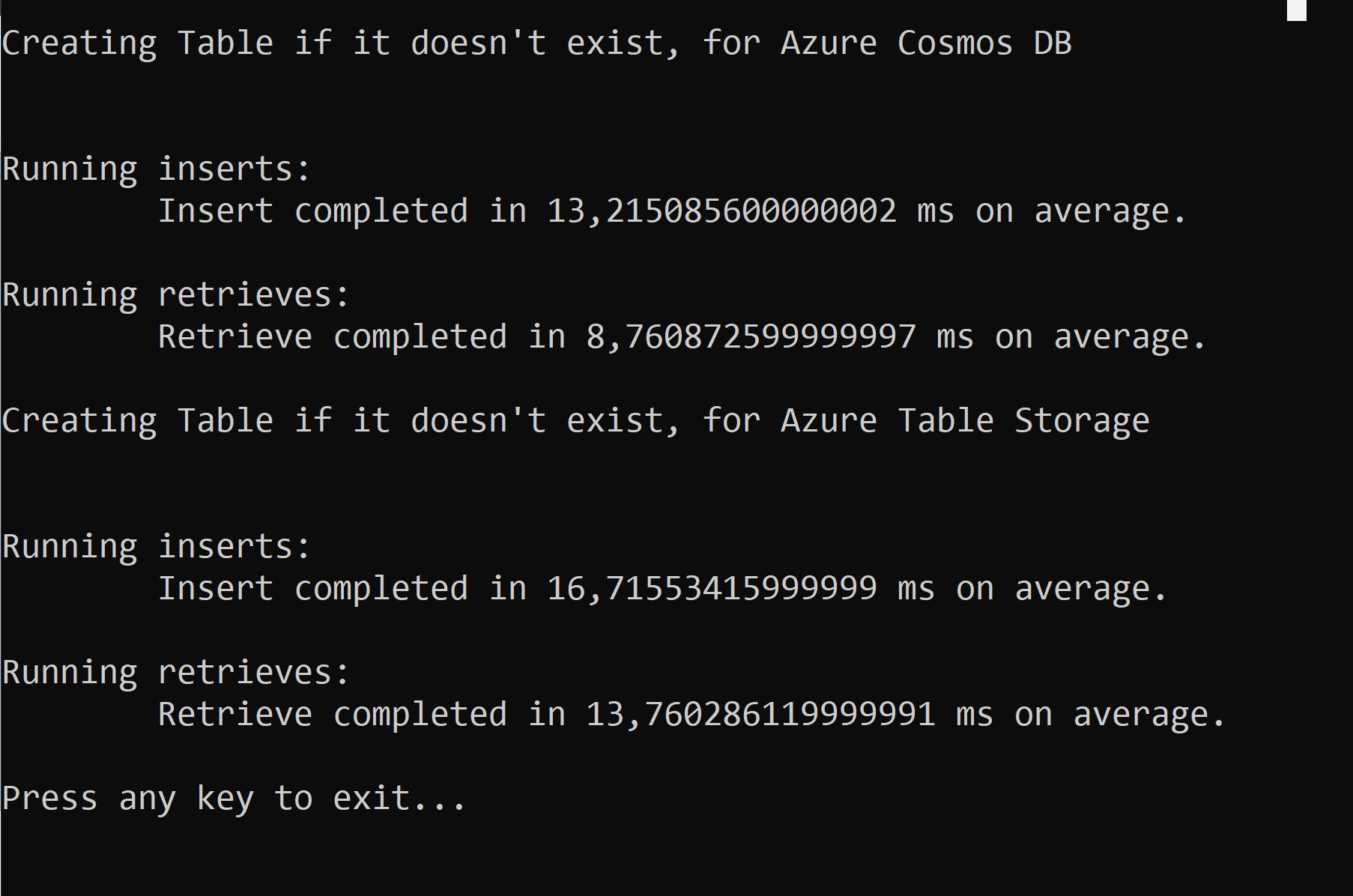
(Azure Cosmos DB Table API vs. Azure Table Storage)
Besides performance, another big difference between Azure Cosmos DB Table API and Azure Table Storage is that Azure Cosmos DB is made for geographic performance and availability (opens new window). You can create writable Azure Cosmos DB nodes all over the world, making your application fast and available everywhere. Also, Azure Cosmos DB has latency for reads and writes included in its SLA (opens new window). It promises to have a read latency below 10 milliseconds, and a write latency below 15 milliseconds. Azure Table Storage doesn't make any latency promises in its SLA (opens new window).
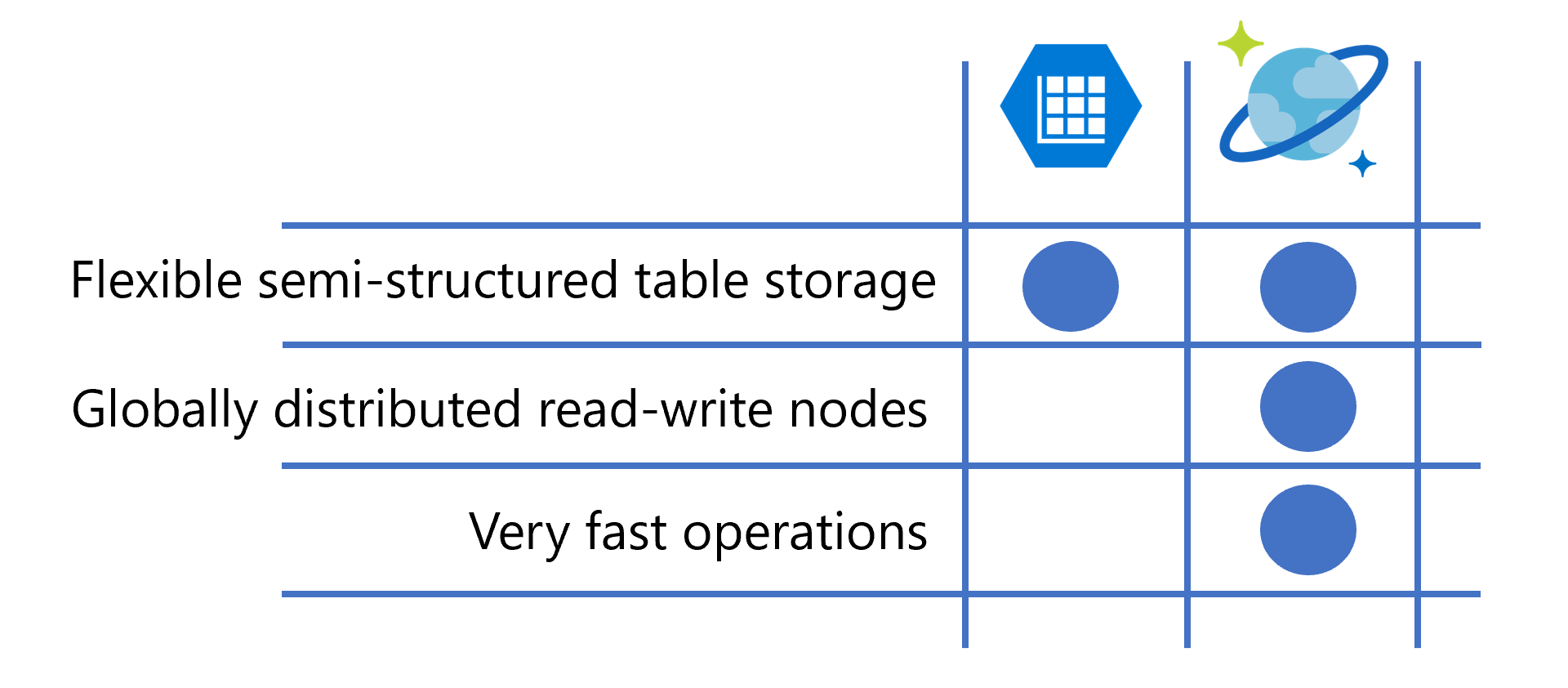
(The biggest differences between Azure Cosmos DB Table API and Azure Table Storage)
# Conclusion
You can use Azure Cosmos DB Table API (opens new window) and Azure Table Storage (opens new window) with the same API and SDK (opens new window). When you need extremely high global performance, you can use Azure Cosmos DB for your applications. And if you need fast and reliable semi-structured table storage, you can use Azure Table Storage. Go and check it out!