TIP
💡 Learn more : Azure storage account overview (opens new window).
# Create an Azure Storage Blob Container through C#
Azure Storage is described as a service that provides storages that is available, secure, durable, scalable, and redundant. Azure Storage consists of 1) Blob storage, 2) File Storage, and 3) Queue storage. In this post, we'll take a look at creating an Azure Storage Blob container with C#. Yesterday (opens new window), I described how to do it through the Azure Portal.
Go ahead and open the Azure Portal and navigate to the Azure Storage account that we worked with yesterday (opens new window).
Look under Settings, then Access Keys and copy the connection string.
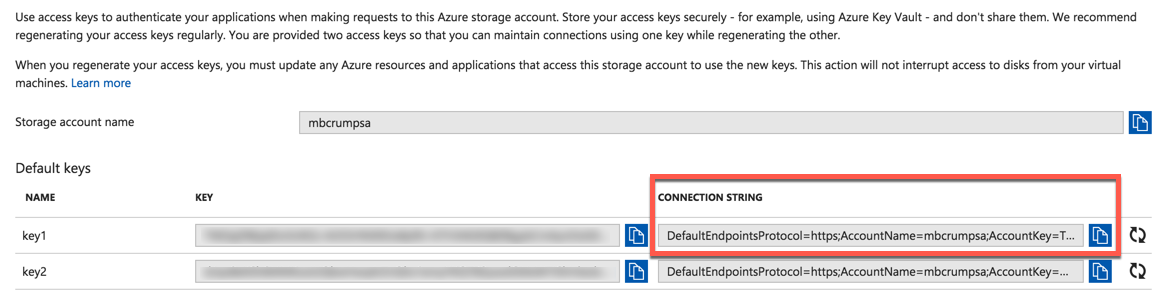
Create a C# Console Application, and use NuGet to pull in references to :
- Azure.Storage.Blob
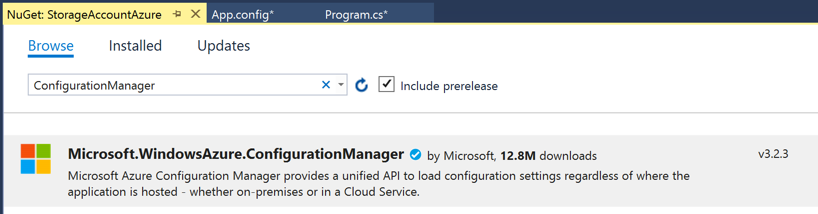
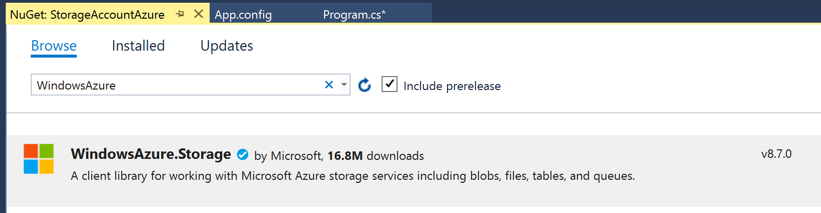
Inside of your Console app, you will see App.config, now add the following section:
<appSettings>
<add key="StorageConnection" value="YOUR-CONNECTION-STRING-COPIED-FROM-EARLIER"/>
</appSettings>
2
3
Copy the following code into your Main method:
static void Main(string[] args)
{
string connectionString = ConfigurationManager.AppSettings("StorageConnection");
BlobContainerClient container = new BlobContainerClient(connectionString, "images-backup");
container.CreateIfNotExists(PublicAccessType.Blob);
Console.ReadLine();
}
2
3
4
5
6
7
This code will get our connection string from the App.config, create our client and a container named images-backup if it doesn't exist. We can go back inside of the portal to see if executed correctly.
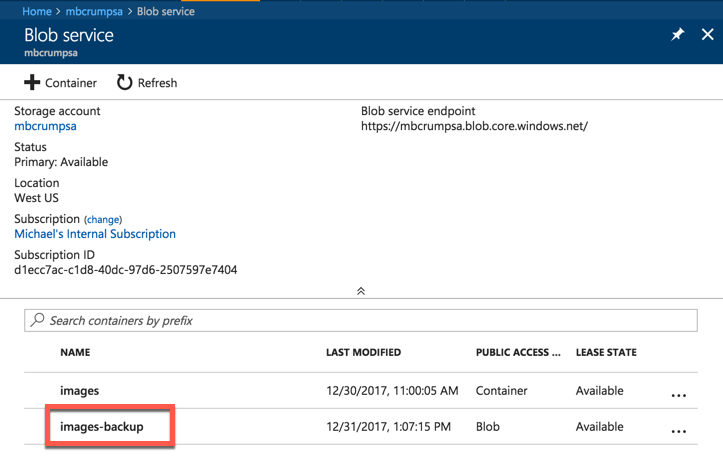