TIP
🔥 Make sure you star the repo (opens new window) to keep up to date with new tips and tricks.
💡 Learn more : Azure Cosmos DB Overview (opens new window).
📺 Watch the video : How to store unstructured data in Azure Cosmos DB with Azure Functions (opens new window).
# How to store unstructured data in Azure Cosmos DB with Azure Functions
# Serverless scaling of unstructured data
Azure Cosmos DB (opens new window) is a great database for unstructured data. It is designed to work with documents, like JSON documents, and is highly scalable and extremely fast. You can also use Azure Cosmos DB in a serverless (opens new window) mode, where you only pay for it when it you need it.
Azure Functions (opens new window) work well with Azure Cosmos DB, because they have bindings (opens new window) that can directly output to Azure Cosmos DB, without you having to write the plumbing code. In this post, we'll use an Azure Function to write unstructured data into Azure Cosmos DB.
# Prerequisites
If you want to follow along, you'll need the following:
- An Azure subscription (If you don't have an Azure subscription, create a free account (opens new window) before you begin)
# Use an Azure Cosmos DB output binding with Azure Functions
We are going to create an Azure Cosmos DB Account and an Azure Function that writes data into Azure Cosmos DB. We'll start by creating the Azure Cosmos DB account.
- Go to the Azure portal (opens new window)
- Click the Create a resource button (the plus-sign in the top left corner)
- Search for cosmos db, select the "Azure Cosmos DB" result, and click Create
- Select a Resource Group
- Type in an Account Name
- Leave the API and Location settings
- Change the Capacity mode to Serverless
- Leave the Account Type to Non-Production
- Click Review + create and then Create
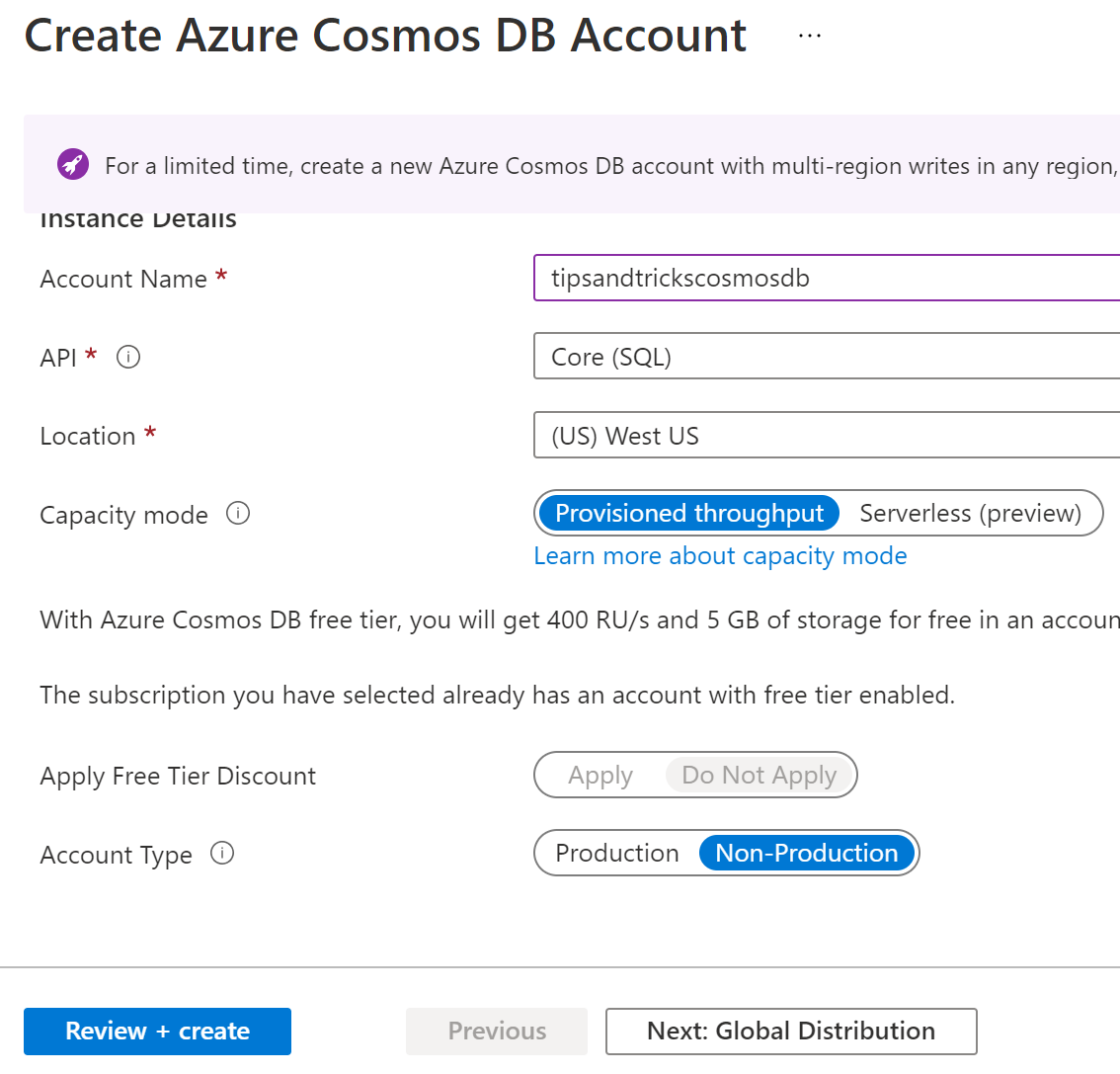
(Create an Azure Cosmos DB account in the Azure portal)
Now that we have an Azure Cosmos DB account, we can create an Azure Function that writes data into it.
- In the Azure portal, click the Create a resource button (the plus-sign in the top left corner)
- Search for function, select the "Function App" result, and click Create
- Select a Resource Group
- Type in a Function App Name
- Pick .NET for the Runtime stack
- Select a .NET Version
- Pick a Region
- Click Review + create and then Create. This creates a Function App that runs in consumption mode
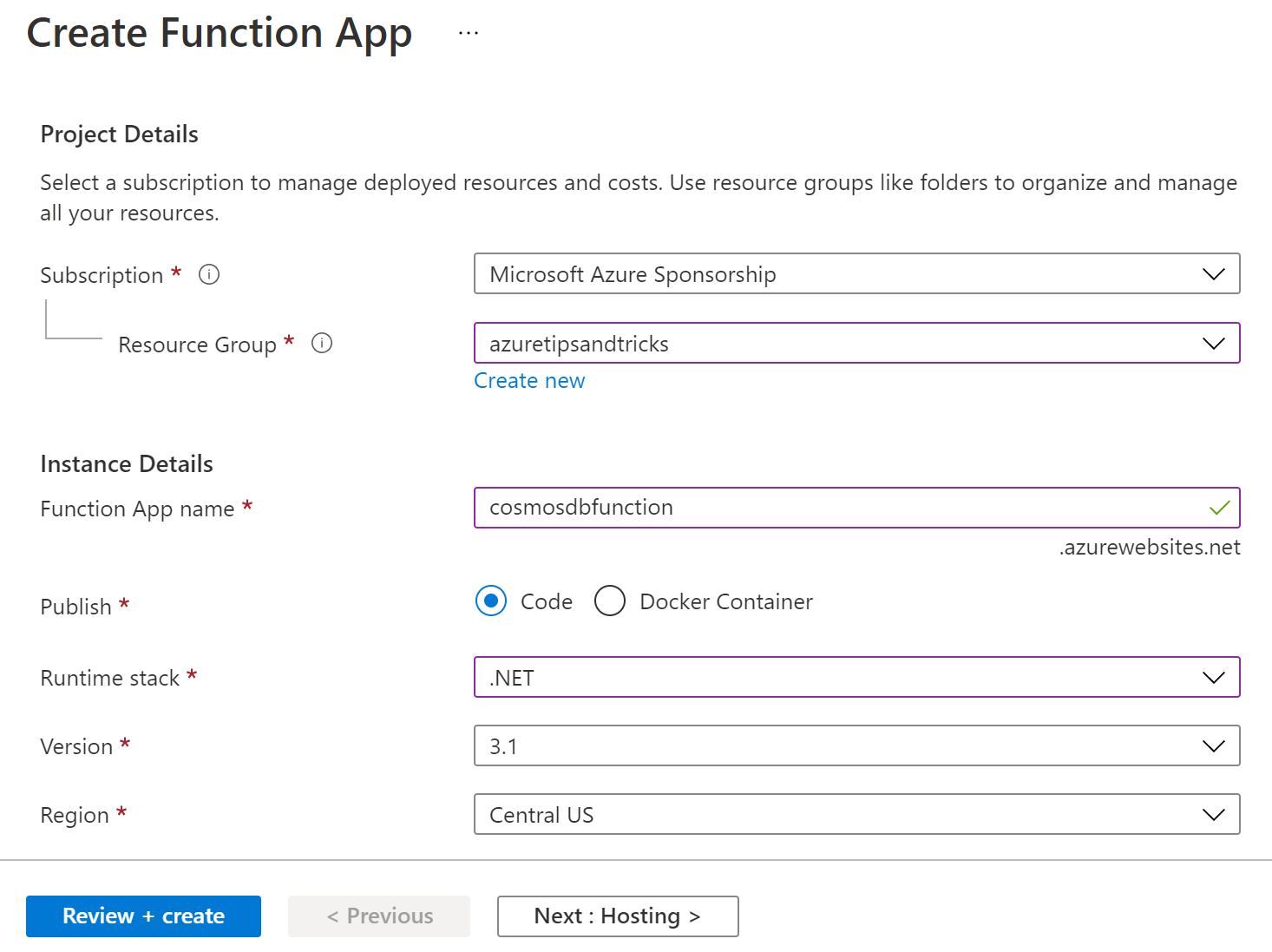
(Create an Azure Function in the Azure portal)
When the Function App is deployed, navigate to it in the Azure portal.
- Navigate to the Functions menu
- Click Add to create a new function. This opens the Add function blade
- Leave the Development environment to Develop in portal
- Select HTTP trigger as the template
- Give the function a Name
- Leave the Authorization level to Function
- Click Add to create the function
- When the function is created, click on it to see its details
- Navigate to the Integration menu of the function
- Next, click + Add output in the Outputs box. We are going to create an output binding that connects to the Azure Cosmos DB
- Change the Binding type to Azure Cosmos DB
- Leave the document, database and collection names as they are
- Set "If true, creates the Aure Cosmos DB database and collection" to True
- For Azure Cosmos DB account connection, click New to create a new connection
- Now select the Azure Cosmos DB that we've created earlier and click OK
- Click OK to create the output binding
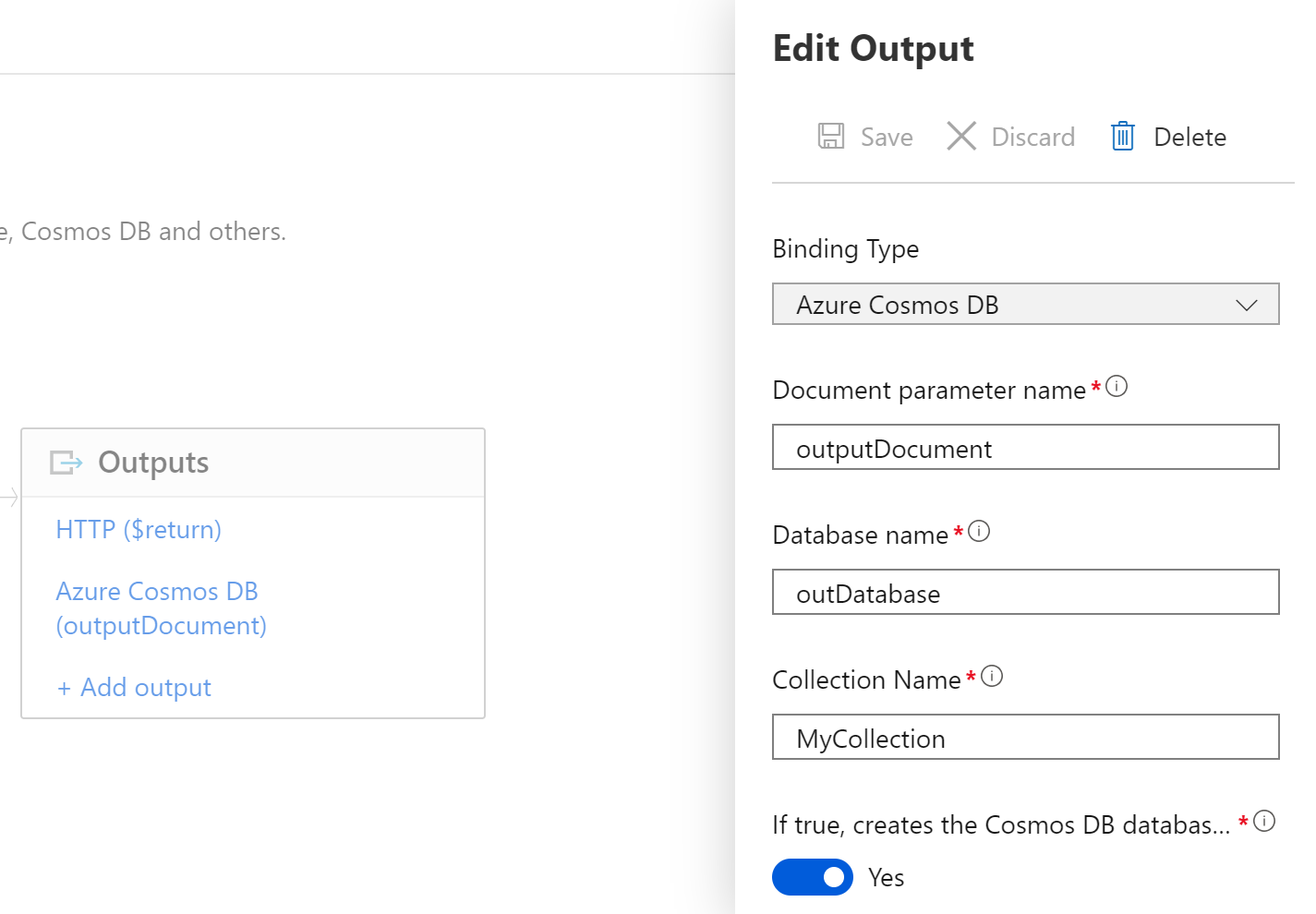
(Create an output binding for the Azure Function)
- Navigate to the Code + Test menu of the function
- Change the code into the following code. This uses the Azure Cosmos DB output binding to write a document to it, when the Function is triggered
#r "Newtonsoft.Json"
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
public static IActionResult Run(HttpRequest req, out object outputDocument, ILogger log)
{
string name = req.Query["name"];
string task = req.Query["task"];
string duedate = req.Query["duedate"];
// We need both name and task parameters.
if (!string.IsNullOrEmpty(name) && !string.IsNullOrEmpty(task))
{
outputDocument = new
{
name,
duedate,
task
};
return (ActionResult)new OkResult();
}
else
{
outputDocument = null;
return (ActionResult)new BadRequestResult();
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
- Click Save to save the code changes and compile the code
- Now Click Test/Run
- In the Input tab of Test/Run, add 3 query parameters with values, like this:
- "name" - "John"
- "duedate" - "03/04/2029"
- "task" - "shopping"
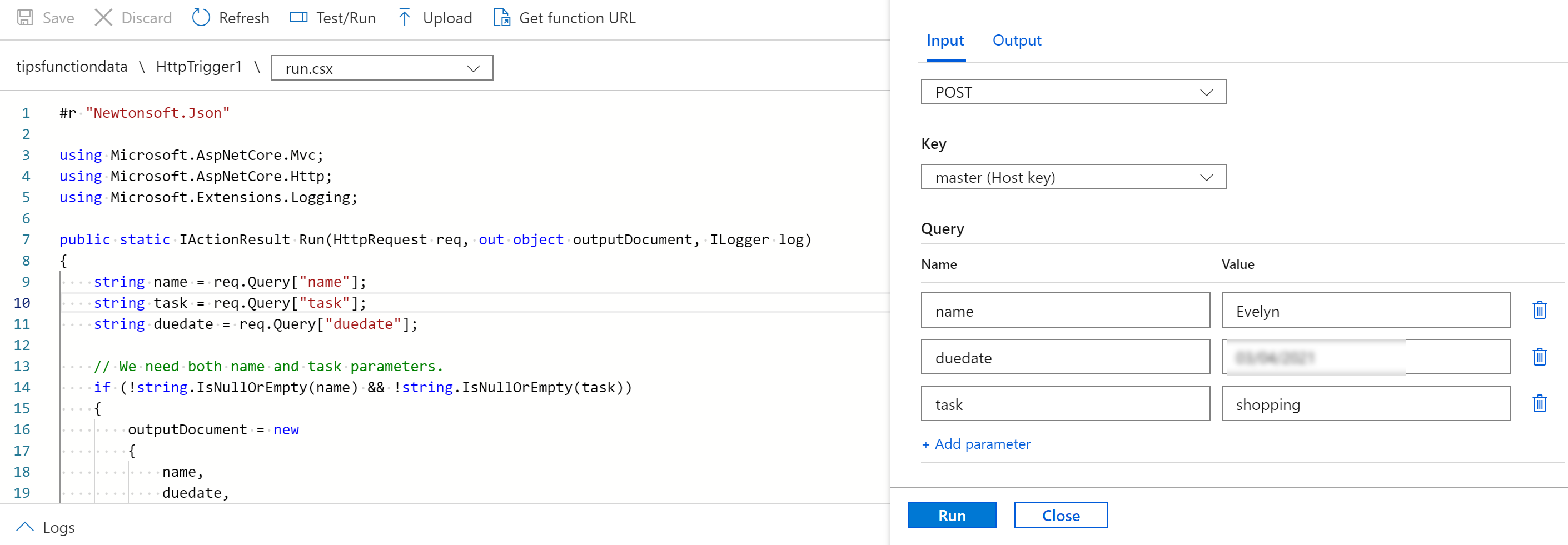
(Test the Azure Function in the Azure portal)
- Click Run. You'll see the HTTP response code 200 OK
- Next, navigate to the Azure Cosmos DB in the Azure portal
- Go to the Data Explorer menu
- Navigate to the items node of the account. Here, you'll see one item, which is the document with unstructured data that the Azure Function just created
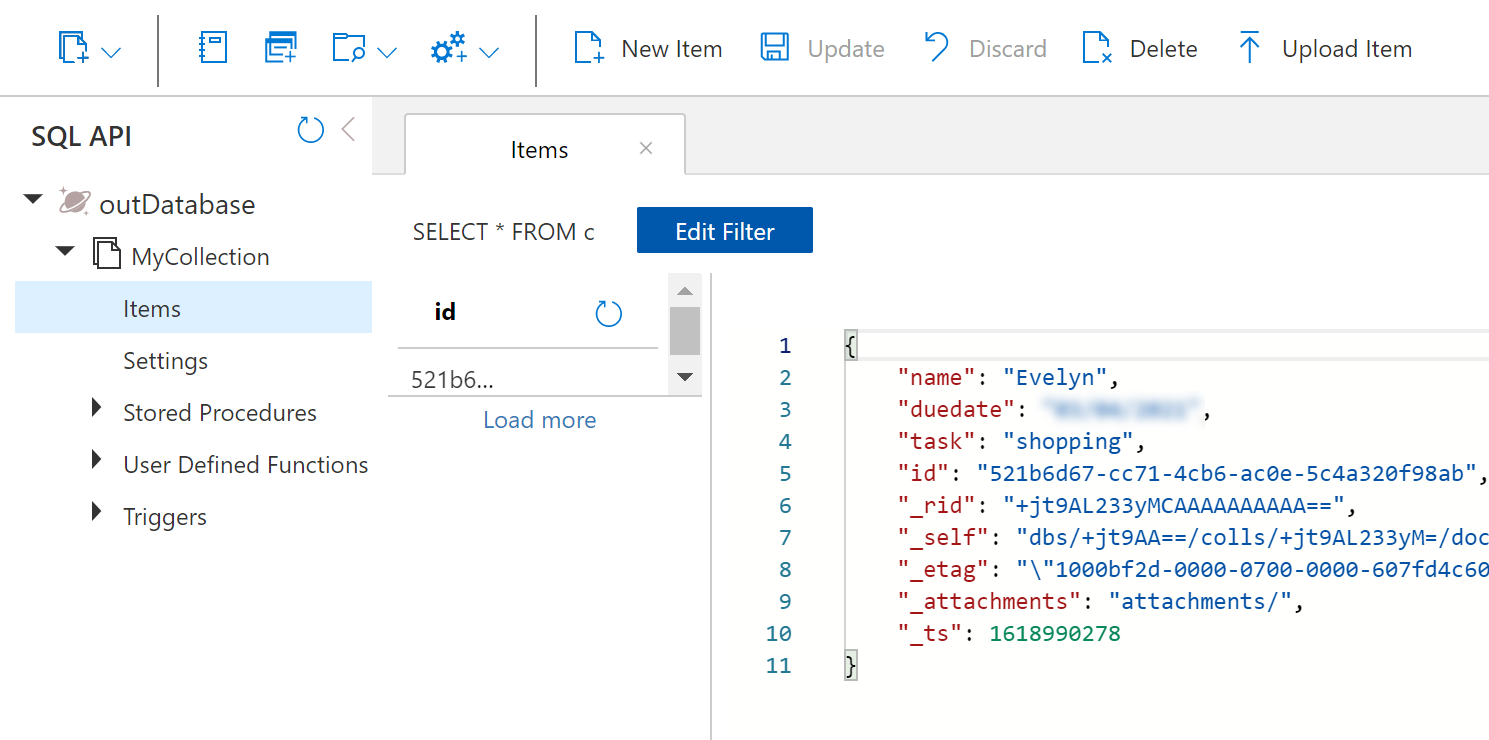
(Document created by Azure Function)
# Conclusion
Azure Functions (opens new window) offer bindings (opens new window) that read and write to other services, without you having to create the plumbing. When you run Azure Functions in consumption mode (opens new window) and use them with Azure Cosmos DB (opens new window) in serverless (opens new window) mode, you have a scalable and affordable solution that works with unstructured data. Go and check it out!