TIP
🔥 Checkout our new Azure Developer page at azure.com/developer (opens new window).
💡 Learn more : Azure Functions Triggers and Bindings (opens new window).
📺 Watch the video : What database should you use in your next Azure Functions app (opens new window).
# What database should you use in your next Azure Functions app
# Azure Functions integration
Azure Functions (opens new window) are great for running background tasks. And Azure Functions can easily connect to other services, like Azure Cosmos DB (opens new window), to read and write data, with minimal plumbing code.
In this post, we'll take a look at using a database with Azure Functions and we'll see which database is best suited for which scenario.
# Prerequisites
If you want to follow along, you'll need the following:
- An Azure subscription (If you don't have an Azure subscription, create a free account (opens new window) before you begin)
# Use a database with Azure Functions
Most applications need some type of data store to work with. This is also true for applications that run as Azure Functions. Connecting to a database from an Azure Function can be extremely simple. For instance, you can store a connection string in the Function App Settings and use a SqlConnection object to connect to Azure SQL, like in the example below:
// Get the connection string from app settings and use it to create a connection.
var str = Environment.GetEnvironmentVariable("sqldb_connection");
using (SqlConnection conn = new SqlConnection(str))
{
conn.Open();
var text = "UPDATE SalesLT.SalesOrderHeader " +
"SET [Status] = 5 WHERE ShipDate < GetDate();";
using (SqlCommand cmd = new SqlCommand(text, conn))
{
// Execute the command and log the # rows affected.
var rows = await cmd.ExecuteNonQueryAsync();
log.LogInformation($"{rows} rows were updated");
}
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
Or, when you want to connect to an Azure Cosmos DB, you can use the Function bindings (opens new window), such as a trigger or input- or output-binding to connect to Cosmos DB, without writing one line of code. The binding creates the plumbing to connect to Cosmos DB, like in this function.json file from a Function with a Cosmos DB Trigger:
{
"bindings": [
{
"type": "cosmosDBTrigger",
"name": "input",
"direction": "in",
"connectionStringSetting": "cosmosdbtips_DOCUMENTDB",
"databaseName": "SampleDB",
"collectionName": "Persons",
"leaseCollectionName": "leases",
"createLeaseCollectionIfNotExists": true
}
]
}
2
3
4
5
6
7
8
9
10
11
12
13
14
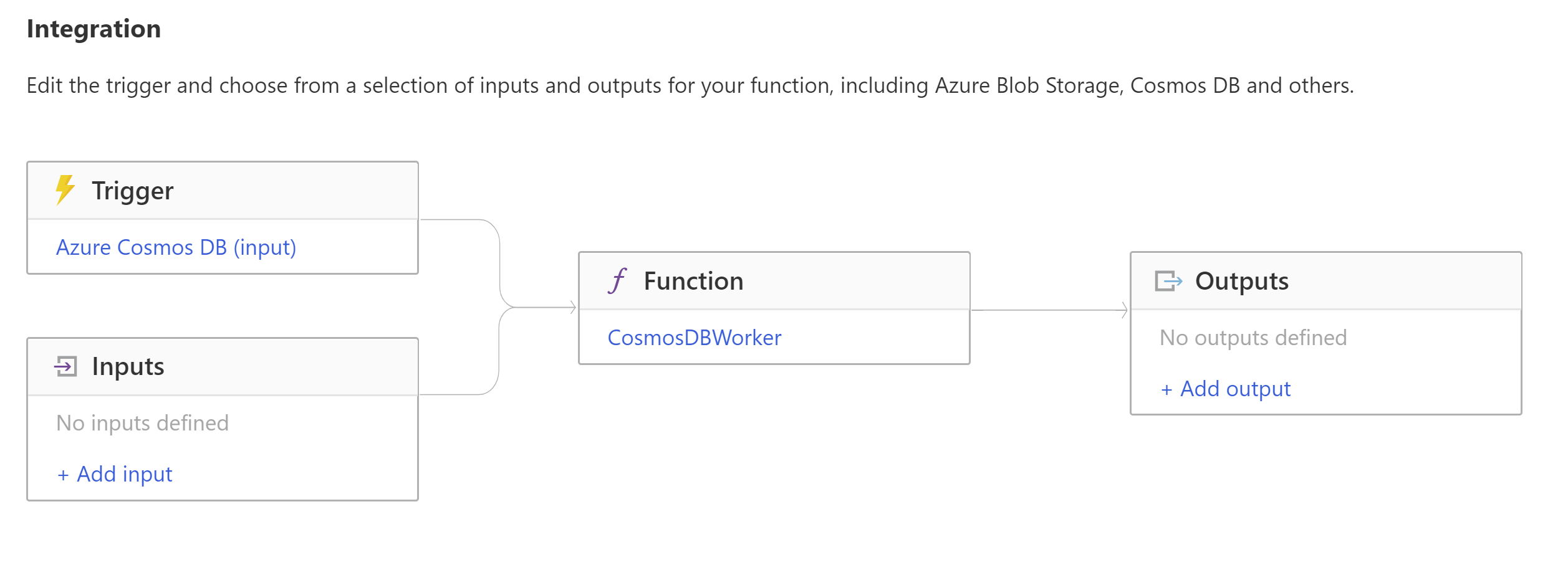
(Using Function bindings to connect to Azure Cosmos DB from an Azure Function)
Connecting to a database is relatively easy. But which database should you use for your Azure Function? In Azure, you can choose from several databases and each has its own characteristics.
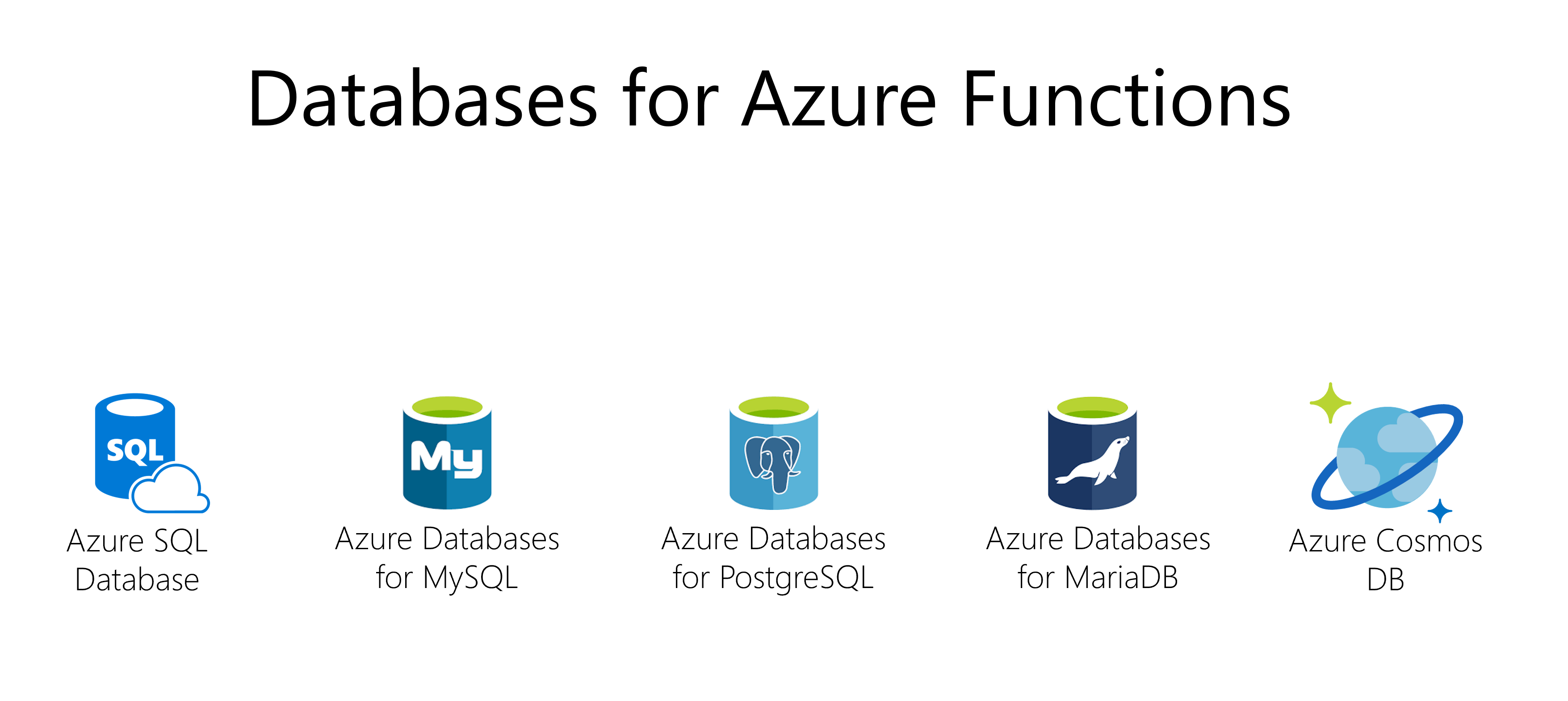
(Database options in Azure)
There is Azure SQL Database (opens new window), which provides scalable relational data storage. And there are Azure Database for MySQL (opens new window), Azure Database for PostgreSQL (opens new window) and Azure Database for MariaDB (opens new window), which also store relational data. And there is also Azure Cosmos DB (opens new window), which stores unstructured- and document data and can interact with data through multiple APIs, including a SQL API (opens new window), a MongoDB API (opens new window) and a Graph API (opens new window).
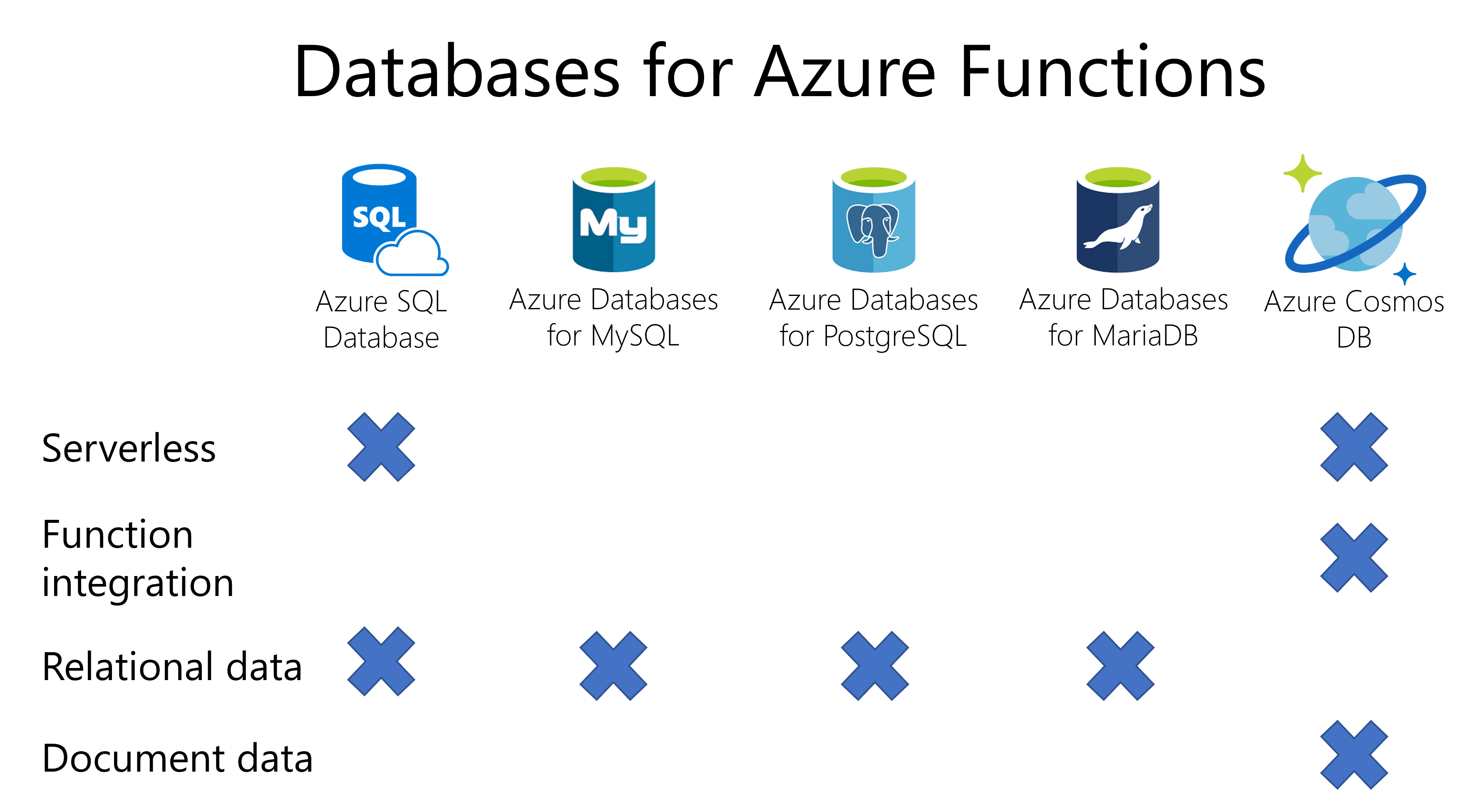
(Comparison of Database options in Azure)
Besides storing relational or non-relational data, these databases are different in that they can run serverless, like an Azure Function and that only Cosmos DB currently has a native integration with Azure Functions through the Function Bindings. The database that you choose, depends on your needs.
# Conclusion
If you need to use a database for your Azure Function (opens new window), you can choose from many different database options, which all have different characteristics. Now, you have the information to make the right choice for your situation. Go and check it out!