TIP
🔥 Checkout our new Azure Developer page at azure.com/developer (opens new window).
💡 Learn more : The official GraphQL website (opens new window).
📺 Watch the video : GraphQL on Azure (opens new window).
# How to use a GraphQL on Azure
# Evolve your APIs with GraphQL
GraphQL (opens new window) is a great way of creating and querying APIs. A GraphQL API is different from a REST API in that it allows the client application to query for certain fields of resources, like the name of a user, and only receive that data. The client controls what data it receives, not the server. Also, GraphQL provides an abstraction layer to the client, which means that clients don't need to query multiple URLs to access different data. GraphQL APIs get all the data a client needs in a single request when you use multiple GraphQL queries in one request.
GraphQL itself is not a framework or runtime that you can use. It is a specification of how to implement an API server-side and how to query it from a client application.
In this post, we'll create a GraphQL API in ASP.NET Core and run that on an Azure App Service Web App (opens new window).
# Prerequisites
If you want to follow along, you'll need the following:
- An Azure subscription (If you don't have an Azure subscription, create a free account (opens new window) before you begin)
- .NET Core latest version SDK (opens new window)
- You'll need the latest version of Visual Studio (opens new window) with the Azure workload installed. Alternatively, you can use Visual Studio Code (opens new window)
# Run a GraphQL API in ASP.NET Core on Azure
Because GraphQL is a specification and not a framework, we can implement it by either creating our own, custom implementation, or by using an existing implementation. In this example, we'll use the existing implementation of the Hot Chocolate library (opens new window). This is a set of libraries that we can use as NuGet packages and support using GraphQL in ASP.NET Core. In fact, the sample application that we will use is the ASP.NET Core Star Wars example application that you can find here (opens new window). It is a simple GraphQL API that exposes information about Star Wars. Let's get started.
First, we will create the sample application on our local machine.
- Open a command prompt
- Create a new directory for the sample application and navigate to it, like this
mkdir starwars
cd starwars
2
- Create a new .NET Core application from the star wars example template with these commands:
dotnet new -i HotChocolate.Templates.StarWars
dotnet new starwars
2
- That's it! Open the folder of the sample application and open the solution file with Visual Studio.
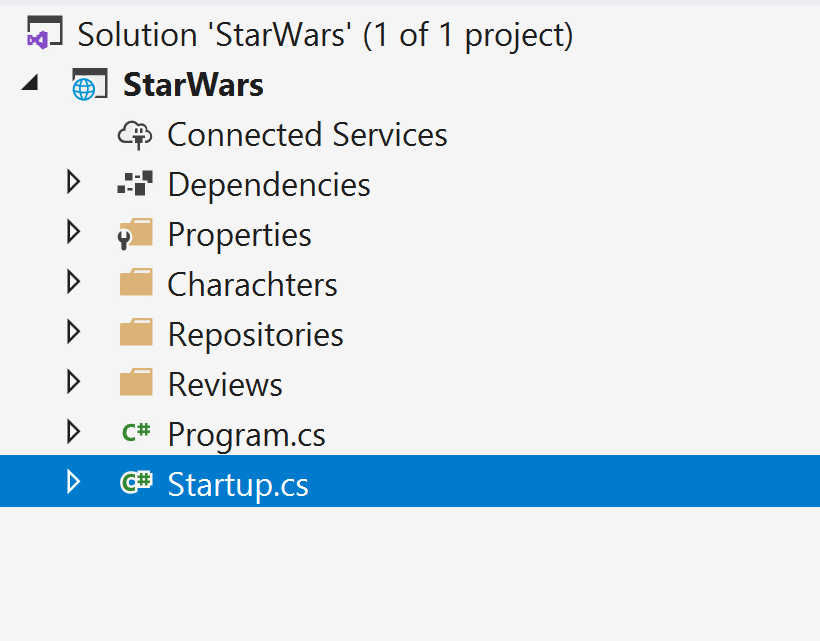
(Star Wars example GraphQL application in Visual Studio)
The example application doesn't have any controllers and contains models and repositories and loads its data in-memory. We can explore what happens in the application by opening the Startup.cs file. In Startup.cs, we see that the file uses the HotChocolate libraries, which provide the magic ingredients to work with GraphQL.
using HotChocolate.AspNetCore;
And in the ConfigureServices method, we can see that the repositories are loaded and that we use an in-memory subscription provider, which loads the data for the GraphQL API in-memory. In a real-world scenario, you would use another data store that retrieves data from, let's say an Azure SQL Database (opens new window). Also, it uses the Hot Chocolate GraphQL ASP.NET Core middleware by using the AddGraphQL method and declaring a new GraphQL schema in it, using queries, mutations, subscriptions and types, like Human and Droid. This makes up the GraphQL API as it lays out which resources are available and which queries can access them. Drill down into any of these types to get more information.
public void ConfigureServices(IServiceCollection services)
{
// Add the custom services like repositories etc ...
services.AddSingleton<ICharacterRepository, CharacterRepository>();
services.AddSingleton<IReviewRepository, ReviewRepository>();
// Add in-memory event provider
services.AddInMemorySubscriptionProvider();
// Add GraphQL Services
services.AddGraphQL(sp => SchemaBuilder.New()
.AddServices(sp)
.AddQueryType(d => d.Name("Query"))
.AddMutationType(d => d.Name("Mutation"))
.AddSubscriptionType(d => d.Name("Subscription"))
.AddType<CharacterQueries>()
.AddType<ReviewQueries>()
.AddType<ReviewMutations>()
.AddType<ReviewSubscriptions>()
.AddType<Human>()
.AddType<Droid>()
.AddType<Starship>()
.Create());
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
Finally, in the Configure method, the application sets up a route for the GraphQL endpoint and includes a playground, which we'll use later to test the API.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app
.UseRouting()
.UseWebSockets()
.UseGraphQL("/graphql")
.UsePlayground("/graphql")
.UseVoyager("/graphql");
}
2
3
4
5
6
7
8
9
10
11
12
13
14
Let's see if we can get this application to run in Azure
- Right-click the project file in Visual Studio and select Publish
- In the wizard that opens, select Azure as the publish target and select Next
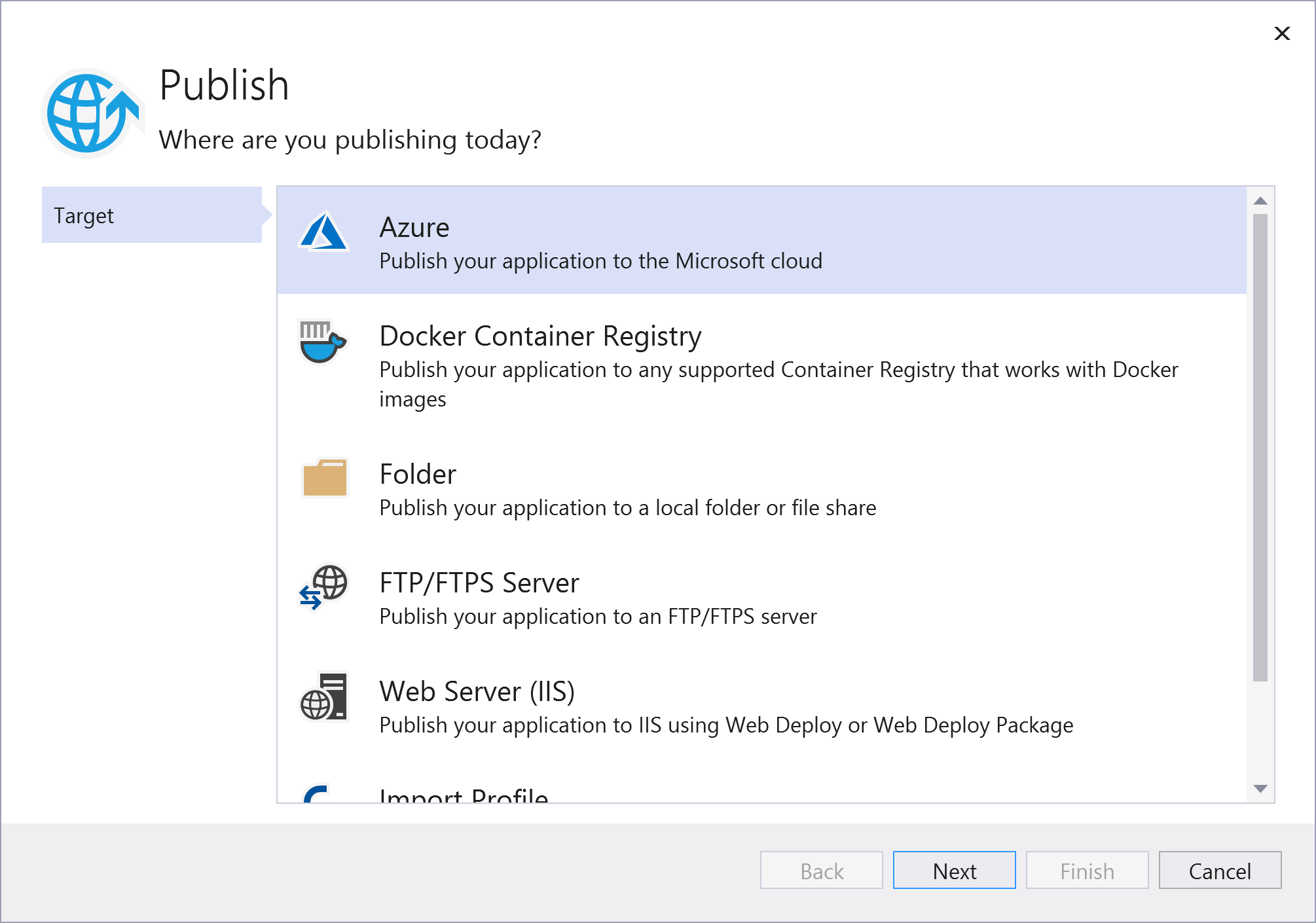
(Publish application window in Visual Studio)
- Next, select Azure App Service (Windows) and select Next
- Now, select "Create a new Azure App Service" as we are going to publish the app to a new App Service Web App
- In the Create new App Service window
- Leave the Name as it is
- Select a Resource group
- Create a new Hosting Plan
- Select Create. This can take a minute as it will create the App Service in Azure
- Once the App Service is created, select it and select Finish
- Now select Publish to publish the application to Azure. Once it is done, it will open the App Service URL in a browser
When the App Service URL is opened in a browser, it will show an error as there is nothing to display. Remember that we can use the GraphQL API with the route /graphql. So let's try that and let's go to the playground by adding /graphql/playground to the URL. This will open the playground, which is a visual GraphQL editor that you can use to test the API. In a real-world scenario, you would consume the API in an application by simply creating an HTTP request and firing it at the GraphQL endpoint.
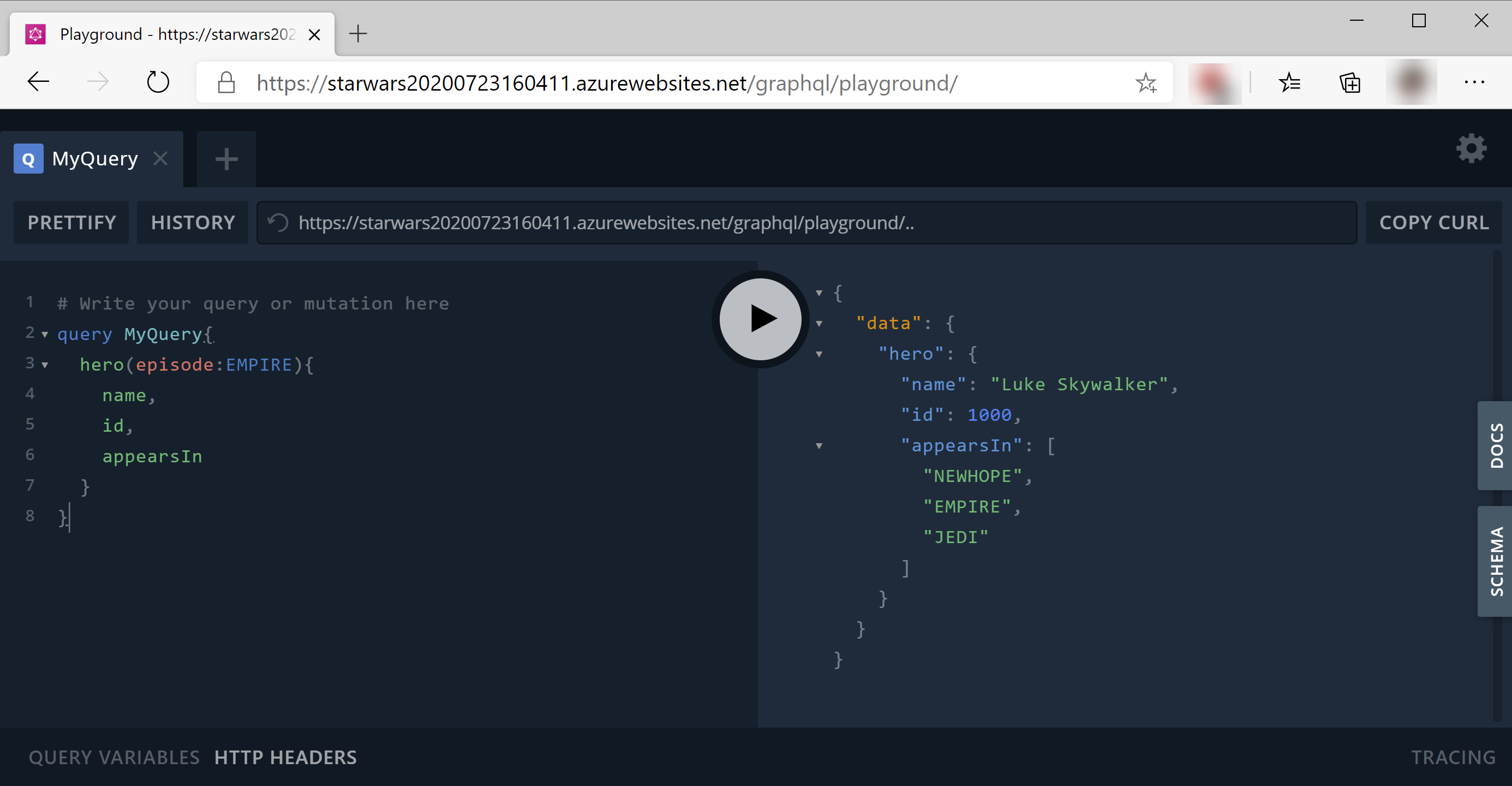
(GraphQL playground running in Azure)
You can now test the API in the playground by creating queries. In the Docs tab on the right, you can find out which queries and resources are available for this API. Here is an example of a query that you can use:
query MyQuery{
hero(episode:EMPIRE){
name
}
}
2
3
4
5
This query uses the hero query to retrieve character data for heroes that were featured in the EMPIRE episode of Star Wars. The fun thing of GraphQL is that you can define that you only want to retrieve certain fields, like the name of the character, and not the complete object.
# Conclusion
You can use implementations of the GraphQL (opens new window) specification to create APIs that allows clients to retrieve only the data that they need, without querying multiple URLs or dealing with API versions. And it is very easy to run a GraphQL API in Azure using an Azure App Service Web App (opens new window). Go and check it out!