This page was generated from
docs/examples/DataSet/Dataset_Performance.ipynb.
Interactive online version:
.
DataSet Performance¶
This notebook shows the trade-off between inserting data into a database row-by-row and as binary blobs. Inserting the data row-by-row means that we have direct access to all the data and may perform queries directly on the values of the data. On the other hand, as we shall see, this is much slower than inserting the data directly as binary blobs.
First, we choose a new location for the database to ensure that we don’t add a bunch of benchmarking data to the default one.
[1]:
import os
import qcodes as qc
cwd = os.getcwd()
qc.config["core"]["db_location"] = os.path.join(cwd, "testing.db")
Logging hadn't been started.
Activating auto-logging. Current session state plus future input saved.
Filename : /home/runner/.qcodes/logs/command_history.log
Mode : append
Output logging : True
Raw input log : False
Timestamping : True
State : active
Qcodes Logfile : /home/runner/.qcodes/logs/241015-11972-qcodes.log
[2]:
%matplotlib inline
import time
from pathlib import Path
import matplotlib.pyplot as plt
import numpy as np
import qcodes as qc
from qcodes.dataset import (
Measurement,
initialise_or_create_database_at,
load_or_create_experiment,
)
from qcodes.parameters import ManualParameter
[3]:
initialise_or_create_database_at(Path.cwd() / "dataset_performance.db")
exp = load_or_create_experiment(experiment_name="tutorial_exp", sample_name="no sample")
Here, we define a simple function to benchmark the time it takes to insert n points with either numeric or array data type. We will compare both the time used to call add_result
and the time used for the full measurement.
[4]:
def insert_data(paramtype, npoints, nreps=1):
meas = Measurement(exp=exp)
x1 = ManualParameter("x1")
x2 = ManualParameter("x2")
x3 = ManualParameter("x3")
y1 = ManualParameter("y1")
y2 = ManualParameter("y2")
meas.register_parameter(x1, paramtype=paramtype)
meas.register_parameter(x2, paramtype=paramtype)
meas.register_parameter(x3, paramtype=paramtype)
meas.register_parameter(y1, setpoints=[x1, x2, x3], paramtype=paramtype)
meas.register_parameter(y2, setpoints=[x1, x2, x3], paramtype=paramtype)
start = time.perf_counter()
with meas.run() as datasaver:
start_adding = time.perf_counter()
for i in range(nreps):
datasaver.add_result(
(x1, np.random.rand(npoints)),
(x2, np.random.rand(npoints)),
(x3, np.random.rand(npoints)),
(y1, np.random.rand(npoints)),
(y2, np.random.rand(npoints)),
)
stop_adding = time.perf_counter()
run_id = datasaver.run_id
stop = time.perf_counter()
tot_time = stop - start
add_time = stop_adding - start_adding
return tot_time, add_time, run_id
Comparison between numeric/array data and binary blob¶
Case1: Short experiment time¶
[5]:
sizes = [1, 500, 1000, 2000, 3000, 4000, 5000]
t_numeric = []
t_numeric_add = []
t_array = []
t_array_add = []
for size in sizes:
tn, tna, run_id_n = insert_data("numeric", size)
t_numeric.append(tn)
t_numeric_add.append(tna)
ta, taa, run_id_a = insert_data("array", size)
t_array.append(ta)
t_array_add.append(taa)
Starting experimental run with id: 1.
Starting experimental run with id: 2.
Starting experimental run with id: 3.
Starting experimental run with id: 4.
Starting experimental run with id: 5.
Starting experimental run with id: 6.
Starting experimental run with id: 7.
Starting experimental run with id: 8.
Starting experimental run with id: 9.
Starting experimental run with id: 10.
Starting experimental run with id: 11.
Starting experimental run with id: 12.
Starting experimental run with id: 13.
Starting experimental run with id: 14.
[6]:
fig, ax = plt.subplots(1, 1)
ax.plot(sizes, t_numeric, "o-", label="Inserting row-by-row")
ax.plot(sizes, t_numeric_add, "o-", label="Inserting row-by-row: add_result only")
ax.plot(sizes, t_array, "d-", label="Inserting as binary blob")
ax.plot(sizes, t_array_add, "d-", label="Inserting as binary blob: add_result only")
ax.legend()
ax.set_xlabel("Array length")
ax.set_ylabel("Time (s)")
fig.tight_layout()
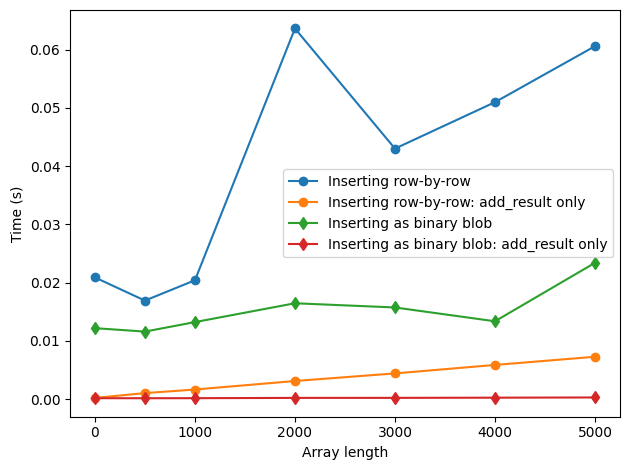
As shown in the latter figure, the time to setup and and close the experiment is approximately 0.4 sec. In case of small array sizes, the difference between inserting values of data as arrays and inserting them row-by-row is relatively unimportant. At larger array sizes, i.e. above 10000 points, the cost of writing data as individual datapoints starts to become important.
Case2: Long experiment time¶
[7]:
sizes = [1, 500, 1000, 2000, 3000, 4000, 5000]
nreps = 100
t_numeric = []
t_numeric_add = []
t_numeric_run_ids = []
t_array = []
t_array_add = []
t_array_run_ids = []
for size in sizes:
tn, tna, run_id_n = insert_data("numeric", size, nreps=nreps)
t_numeric.append(tn)
t_numeric_add.append(tna)
t_numeric_run_ids.append(run_id_n)
ta, taa, run_id_a = insert_data("array", size, nreps=nreps)
t_array.append(ta)
t_array_add.append(taa)
t_array_run_ids.append(run_id_a)
Starting experimental run with id: 15.
Starting experimental run with id: 16.
Starting experimental run with id: 17.
Starting experimental run with id: 18.
Starting experimental run with id: 19.
Starting experimental run with id: 20.
Starting experimental run with id: 21.
Starting experimental run with id: 22.
Starting experimental run with id: 23.
Starting experimental run with id: 24.
Starting experimental run with id: 25.
Starting experimental run with id: 26.
Starting experimental run with id: 27.
Starting experimental run with id: 28.
[8]:
fig, ax = plt.subplots(1, 1)
ax.plot(sizes, t_numeric, "o-", label="Inserting row-by-row")
ax.plot(sizes, t_numeric_add, "o-", label="Inserting row-by-row: add_result only")
ax.plot(sizes, t_array, "d-", label="Inserting as binary blob")
ax.plot(sizes, t_array_add, "d-", label="Inserting as binary blob: add_result only")
ax.legend()
ax.set_xlabel("Array length")
ax.set_ylabel("Time (s)")
fig.tight_layout()
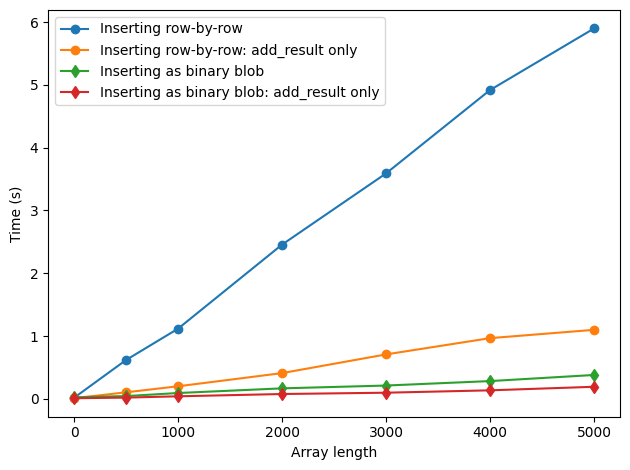
However, as we increase the length of the experiment, as seen here by repeating the insertion 100 times, we see a big difference between inserting values of the data row-by-row and inserting it as a binary blob.
Loading the data¶
[9]:
from qcodes.dataset import load_by_id
As usual you can load the data by using the load_by_id
function but you will notice that the different storage methods are reflected in shape of the data as it is retrieved.
[10]:
run_id_n = t_numeric_run_ids[0]
run_id_a = t_array_run_ids[0]
[11]:
ds = load_by_id(run_id_n)
ds.get_parameter_data("x1")
[11]:
{'x1': {'x1': array([0.71866226, 0.71866226, 0.39042342, 0.39042342, 0.6004229 ,
0.6004229 , 0.63482711, 0.63482711, 0.35814353, 0.35814353,
0.08278772, 0.08278772, 0.13191105, 0.13191105, 0.44266672,
0.44266672, 0.26609247, 0.26609247, 0.88546153, 0.88546153,
0.07631894, 0.07631894, 0.86349187, 0.86349187, 0.7148823 ,
0.7148823 , 0.25020365, 0.25020365, 0.97086847, 0.97086847,
0.54687865, 0.54687865, 0.87363543, 0.87363543, 0.59480561,
0.59480561, 0.24557528, 0.24557528, 0.16074907, 0.16074907,
0.86970374, 0.86970374, 0.59270124, 0.59270124, 0.76454583,
0.76454583, 0.33514043, 0.33514043, 0.6809273 , 0.6809273 ,
0.17221779, 0.17221779, 0.41895269, 0.41895269, 0.42033025,
0.42033025, 0.02383644, 0.02383644, 0.05803774, 0.05803774,
0.77502288, 0.77502288, 0.89597085, 0.89597085, 0.56309986,
0.56309986, 0.24676126, 0.24676126, 0.02584401, 0.02584401,
0.80243015, 0.80243015, 0.01774343, 0.01774343, 0.43092286,
0.43092286, 0.73103694, 0.73103694, 0.34602871, 0.34602871,
0.32647833, 0.32647833, 0.46966001, 0.46966001, 0.90270439,
0.90270439, 0.89030886, 0.89030886, 0.49262277, 0.49262277,
0.15977092, 0.15977092, 0.62201284, 0.62201284, 0.83990368,
0.83990368, 0.41166176, 0.41166176, 0.75287868, 0.75287868,
0.29146966, 0.29146966, 0.21007895, 0.21007895, 0.08469673,
0.08469673, 0.67924384, 0.67924384, 0.95277164, 0.95277164,
0.17799725, 0.17799725, 0.45479308, 0.45479308, 0.46453319,
0.46453319, 0.64372561, 0.64372561, 0.59638826, 0.59638826,
0.73109864, 0.73109864, 0.09964604, 0.09964604, 0.35243502,
0.35243502, 0.83000323, 0.83000323, 0.4598876 , 0.4598876 ,
0.31387187, 0.31387187, 0.23035444, 0.23035444, 0.77113142,
0.77113142, 0.71883677, 0.71883677, 0.19938377, 0.19938377,
0.74328821, 0.74328821, 0.8647432 , 0.8647432 , 0.58285112,
0.58285112, 0.5853515 , 0.5853515 , 0.88425435, 0.88425435,
0.31052817, 0.31052817, 0.12398444, 0.12398444, 0.08452815,
0.08452815, 0.53104282, 0.53104282, 0.47384458, 0.47384458,
0.17659882, 0.17659882, 0.34770426, 0.34770426, 0.26567611,
0.26567611, 0.72288678, 0.72288678, 0.94182063, 0.94182063,
0.2886015 , 0.2886015 , 0.55870128, 0.55870128, 0.52849205,
0.52849205, 0.77958768, 0.77958768, 0.02342402, 0.02342402,
0.3884887 , 0.3884887 , 0.28333748, 0.28333748, 0.62882575,
0.62882575, 0.56134386, 0.56134386, 0.86240225, 0.86240225,
0.01749747, 0.01749747, 0.2517392 , 0.2517392 , 0.22510414,
0.22510414, 0.0711581 , 0.0711581 , 0.04742403, 0.04742403])}}
And a dataset stored as binary arrays
[12]:
ds = load_by_id(run_id_a)
ds.get_parameter_data("x1")
[12]:
{'x1': {'x1': array([[0.50945252],
[0.50945252],
[0.58736464],
[0.58736464],
[0.75984761],
[0.75984761],
[0.18520785],
[0.18520785],
[0.01909334],
[0.01909334],
[0.40855023],
[0.40855023],
[0.75419488],
[0.75419488],
[0.93077756],
[0.93077756],
[0.27506882],
[0.27506882],
[0.954878 ],
[0.954878 ],
[0.35701867],
[0.35701867],
[0.60813489],
[0.60813489],
[0.35082855],
[0.35082855],
[0.66504002],
[0.66504002],
[0.34012516],
[0.34012516],
[0.86034861],
[0.86034861],
[0.13575505],
[0.13575505],
[0.94650665],
[0.94650665],
[0.66177974],
[0.66177974],
[0.55729462],
[0.55729462],
[0.19466361],
[0.19466361],
[0.14543661],
[0.14543661],
[0.86569407],
[0.86569407],
[0.90745176],
[0.90745176],
[0.59019987],
[0.59019987],
[0.3472265 ],
[0.3472265 ],
[0.50651513],
[0.50651513],
[0.22331501],
[0.22331501],
[0.04134666],
[0.04134666],
[0.20331549],
[0.20331549],
[0.57493977],
[0.57493977],
[0.10512776],
[0.10512776],
[0.48597126],
[0.48597126],
[0.67743243],
[0.67743243],
[0.67567434],
[0.67567434],
[0.88433927],
[0.88433927],
[0.39481657],
[0.39481657],
[0.29161622],
[0.29161622],
[0.11818124],
[0.11818124],
[0.29769665],
[0.29769665],
[0.41792287],
[0.41792287],
[0.560753 ],
[0.560753 ],
[0.95021406],
[0.95021406],
[0.2001277 ],
[0.2001277 ],
[0.47823083],
[0.47823083],
[0.81868302],
[0.81868302],
[0.14740075],
[0.14740075],
[0.09292116],
[0.09292116],
[0.81276 ],
[0.81276 ],
[0.88039079],
[0.88039079],
[0.06258391],
[0.06258391],
[0.16717479],
[0.16717479],
[0.3078916 ],
[0.3078916 ],
[0.54573161],
[0.54573161],
[0.99656548],
[0.99656548],
[0.87512193],
[0.87512193],
[0.37742499],
[0.37742499],
[0.45845407],
[0.45845407],
[0.17926097],
[0.17926097],
[0.15917684],
[0.15917684],
[0.29503389],
[0.29503389],
[0.31354605],
[0.31354605],
[0.37963301],
[0.37963301],
[0.26175505],
[0.26175505],
[0.45087296],
[0.45087296],
[0.13514925],
[0.13514925],
[0.51278344],
[0.51278344],
[0.93310148],
[0.93310148],
[0.02193074],
[0.02193074],
[0.65734879],
[0.65734879],
[0.8370163 ],
[0.8370163 ],
[0.80947504],
[0.80947504],
[0.30022974],
[0.30022974],
[0.50236876],
[0.50236876],
[0.67507625],
[0.67507625],
[0.51479413],
[0.51479413],
[0.13753884],
[0.13753884],
[0.29298064],
[0.29298064],
[0.46187673],
[0.46187673],
[0.60713446],
[0.60713446],
[0.45812405],
[0.45812405],
[0.73380209],
[0.73380209],
[0.05661568],
[0.05661568],
[0.28611635],
[0.28611635],
[0.39175695],
[0.39175695],
[0.27228503],
[0.27228503],
[0.7948499 ],
[0.7948499 ],
[0.30913822],
[0.30913822],
[0.52054483],
[0.52054483],
[0.65028495],
[0.65028495],
[0.35036828],
[0.35036828],
[0.91994668],
[0.91994668],
[0.22979063],
[0.22979063],
[0.8756904 ],
[0.8756904 ],
[0.20294772],
[0.20294772],
[0.60580231],
[0.60580231],
[0.9247468 ],
[0.9247468 ],
[0.80359229],
[0.80359229],
[0.60067768],
[0.60067768],
[0.91535234],
[0.91535234]])}}
[ ]: